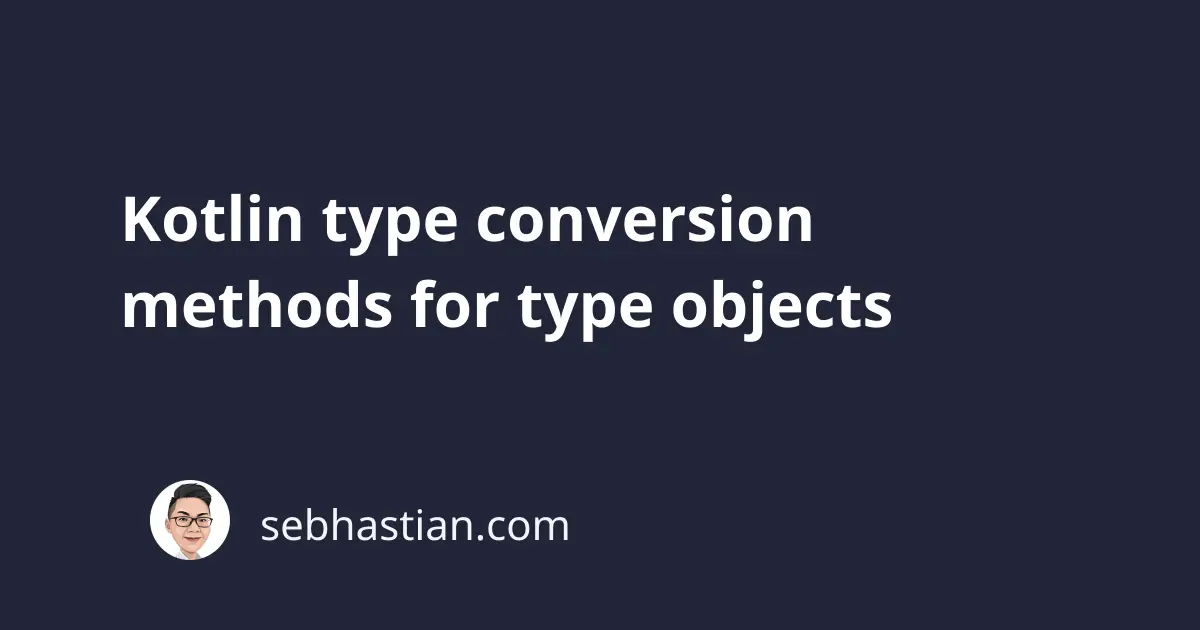
Even though the Kotlin as
operator is used to cast one value type into another, the operator can’t convert a String
type to an Int
type even when the string has numerical representation.
For example, the following String
casting will cause an error:
var myStr: String = "9"
var myInt: Int = myStr as Int
// Error ClassCastException
Kotlin Object classes have specific methods that you can use to convert value types from one type into another.
For example, the String
object has the toInt()
and toBoolean()
methods that you can use to convert a string into an integer or a boolean.
Here’s how you can convert a String
value into Int
and Boolean
values:
var myStr: String = "9"
var myInt: Int = myStr.toInt()
var myBool: Boolean = myStr.toBoolean()
print(myInt) // 9
print(myBool) // false
But when myStr
value doesn’t have an Int
equivalent, then the toInt()
method will throw a NumberFormatException
error.
To convert successfully, you need to use the toIntOrNull()
method, which will return null
when the String
value doesn’t have an Int
equivalent.
You also need to declare the myInt
variable as nullable Int
type as shown below:
var myStr: String = "Hello"
var myInt: Int? = myStr.toIntOrNull()
print(myInt) // null
All value types are objects in Kotlin, so you can use specific methods from each type object to convert its value.
The String
object type methods that can do type conversion are as follows:
toInt()
toLong()
toFloat()
toDouble()
toBoolean()
toCharArray()
For non String
types like Int
, the object should also have the toString()
method to convert the value to String
.
All conversion methods will have the OrNull()
alternative method to return null
instead of throwing an error when the value can’t be converted.
And that’s how type conversion methods work in Kotlin.