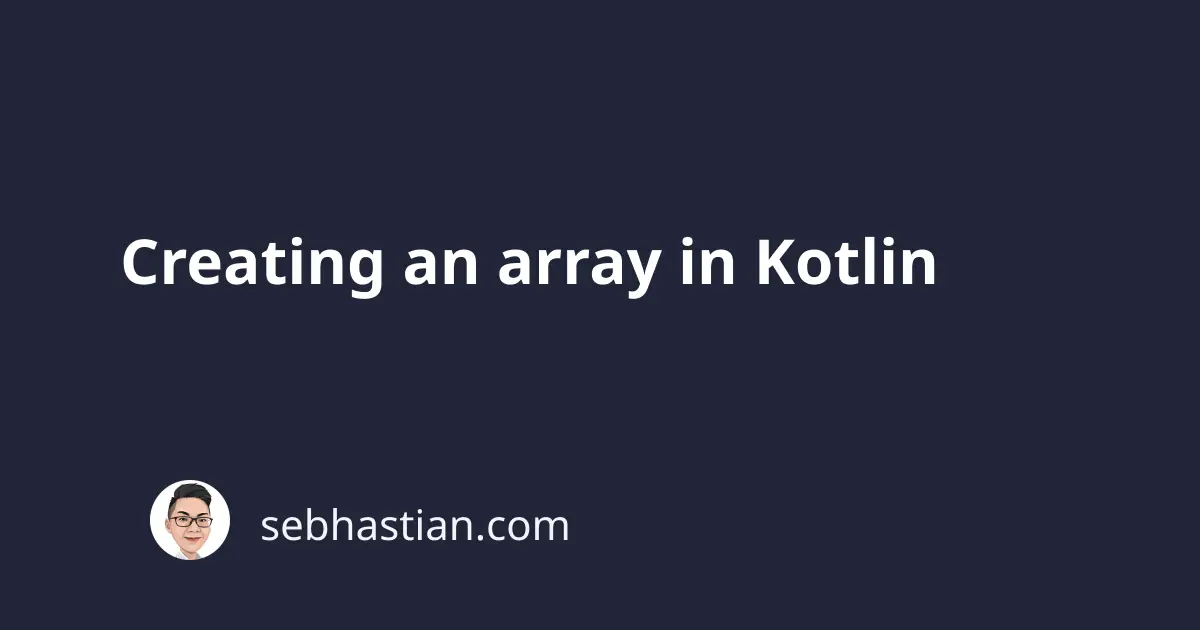
Kotlin has the Array
class which is used to represent an array of a specific type.
An Array
instance is a container that can store multiple values under one variable. The values are also indexed, which means you can access them from the index order that starts from 0
.
To create an array in Kotlin, you need to call the arrayOf()
function and provide the values of your array as arguments to the function.
For example, here’s how to create an array of numbers 1
to 3
:
val myArray = arrayOf(1, 2, 3)
Since the arguments are Int
types, the type of the array will be inferred as Int
.
You can also specify the type of the array as shown below:
val strArray = arrayOf<String>("Nathan", "Kotlin")
But Kotlin IDEs might recommend you to move the explicit type because Kotlin can infer native types just fine.
Kotlin can even infer from a custom type created using a class
as follows:
class Dog (var name:String)
val dogs = arrayOf(
Dog("Puppy"),
Dog("Jackson")
)
Aside from using the arrayOf()
function, you can also use the Array
class constructor to create an array.
The Array
class constructor requires two arguments:
- The first is an
Int
specifying the size of the array - The second is a callback function that returns the value of each index. Kotlin will provide you with the index.
The code below will create an Array
that has a size of 3
where each index will have the value of index number + 2
:
val myArray = Array(3) { i -> i + 2 }
The values of myArray
above will be:
2
(0 + 2
)3
(1 + 2
)4
(2 + 2
)
The second argument for the Array()
constructor above is a lambda expression, and lambda expressions in Kotlin can be written outside the parentheses.
See also: Kotlin lambda expression explained
Next, let’s see how you can access the values of a Kotlin Array
instance.
Accessing and setting array values
To access an array value at a specific index, you can use the array name followed by an index operator as follows:
val myArray = arrayOf(1, 2, 3)
println(myArray[0]) // 1
println(myArray[1]) // 2
println(myArray[2]) // 3
An Array
index starts from 0
, so index operator [0]
will point to the first element you insert into the Array
.
To set a new value to a specific index, you need to follow the index operator with the assignment operator =
as shown below:
val myArray = arrayOf(1, 2, 3)
myArray[0] = 759
println(myArray[0]) // 759
println(myArray[1]) // 2
println(myArray[2]) // 3
And that’s how you can get and set values from an Array
.
Traversing an array
A Kotlin Array
variable is also a collection, so you can loop over the array using a for...in
syntax as shown below:
class Dog (var name:String)
val dogs = arrayOf(
Dog("Puppy"),
Dog("Jackson")
)
for (dog in dogs) {
println(dog.name)
}
You can also use the Array.forEach
method like this:
dogs.forEach { println(it.name) }
Both for...in
and Array.forEach
will generate the same output:
Puppy
Jackson
An Array
instance size is fixed on initialization, which means you can’t add or remove elements from the Array
once initialized.
If you need an Array
with dynamic size, you need to use the ArrayList
class.