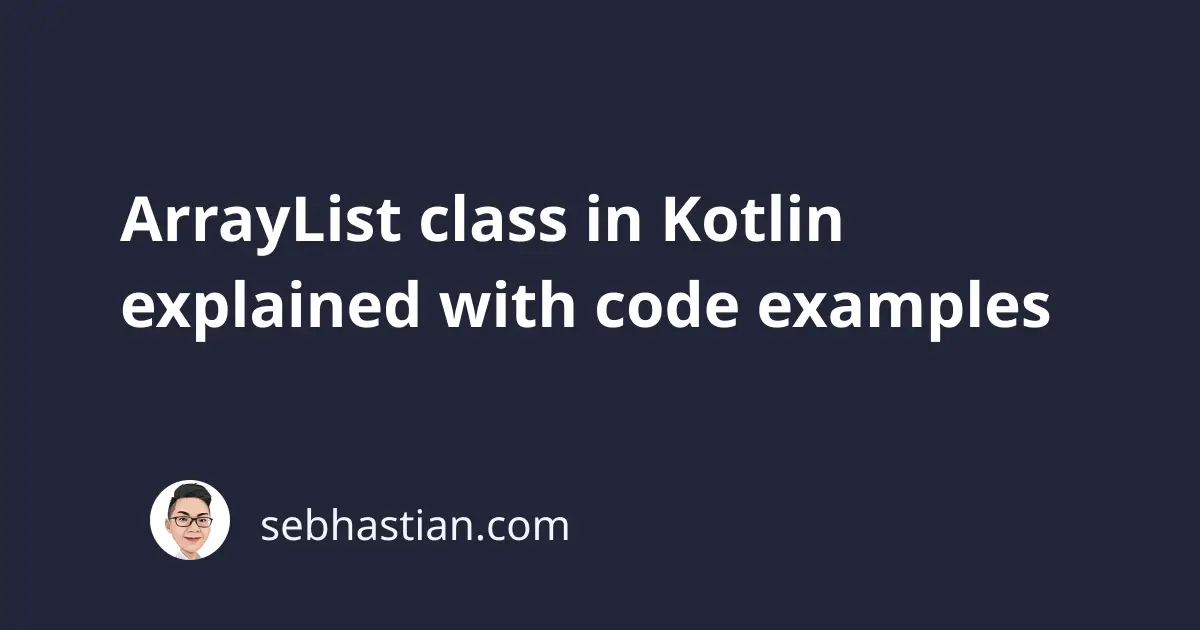
The ArrayList
class in Kotlin can be used to create a variable of ArrayList
type.
An ArrayList
type is a dynamic implementation of the array data type that allows you to add and remove element(s) from the array.
This means that the size of an ArrayList
type can also increase or decrease dynamically.
Creating an ArrayList variable
To create an ArrayList
instance, you can use the arrayListOf()
function and assign the return to a variable.
The following example creates an ArrayList
of Int
type named myArrayList
:
val myArrayList = arrayListOf(1, 2, 3)
println(myArrayList) // [1, 2, 3]
You can also declare the variable type as an ArrayList
with a specific generic as shown below:
val myArrayList: ArrayList<Int> = arrayListOf(1, 2, 3)
Finally, you can also declare an empty ArrayList
of a specific type like this:
val myArrayList = ArrayList<Int>()
println(myArrayList) // []
Once you declare an ArrayList
variable, you can add, remove, or get an element from a specific index.
Let’s learn how to add a new element first.
Kotlin ArrayList adding new element(s)
To add a new element to an ArrayList
variable, you can use the add()
or addAll()
method from the ArrayList
class.
The add()
method adds a specified element to the end of the ArrayList
:
val myArrayList = arrayListOf(1, 2, 3)
myArrayList.add(77)
println(myArrayList) // [1, 2, 3, 77]
myArrayList.add(102)
println(myArrayList) // [1, 2, 3, 77, 102]
The addAll()
method is used to add all elements of a collection (or list) to the end of the ArrayList
.
The following example adds a List
named myList
to an ArrayList
named numbers
:
val numbers = arrayListOf(1, 2, 3)
val myList = listOf(55, 179)
numbers.addAll(myList)
println(numbers) // [1, 2, 3, 55, 179]
Next, let’s look at how to remove elements from an ArrayList
.
Kotlin ArrayList removing element(s)
Kotlin ArrayList
class has multiple methods that you can use to remove element(s) from its instance.
Some of these remove methods are as shown below:
clear()
remove()
removeAll()
retainAll()
removeLast()
The clear()
method will remove all elements from an ArrayList
as shown below:
val myArrayList = arrayListOf(1, 2, 3)
println(myArrayList) // [1, 2, 3]
myArrayList.clear()
println(myArrayList) // []
The remove()
method will remove the element you specify as its argument.
When the ArrayList
contains multiple identical elements, only the element at the lowest index will be removed.
When the element isn’t found, the method simply does nothing:
val myArrayList = arrayListOf(1, 2, 3, 1)
myArrayList.remove(1)
println(myArrayList) // [2, 3, 1]
myArrayList.remove(20)
println(myArrayList) // [2, 3, 1]
The remove()
method also returns true
when an element is removed. Otherwise, it returns false
.
You don’t need to capture the return value if you don’t use it.
The removeAll()
method accepts a list as its argument, and it removes all elements in an ArrayList
that are identical to the ones in the list.
Here’s an example of the removeAll()
method in action:
val myArrayList = arrayListOf(1, 2, 3, 1)
val myList = listOf(1, 2, 3)
myArrayList.removeAll(myList)
println(myArrayList) // []
As you can see from the above example, the removeAll()
method will also remove all duplicates of elements that are in the list.
The retainAll()
method is the opposite of the removeAll()
method.
It will remove all elements that are not present in the list:
val myArrayList = arrayListOf(1, 2, 3, 1)
val myList = listOf(2, 3)
myArrayList.retainAll(myList)
println(myArrayList) // [2, 3]
Finally, the removeLast()
method allows you to remove the element at the last index of the ArrayList
variable.
Take a look at the following example:
val myArrayList = arrayListOf(1, 2, 3, 44, 99)
myArrayList.removeLast()
println(myArrayList) // [1, 2, 3, 44]
Kotlin ArrayList set() method
The set()
method replaces the element at a certain index with another element.
The method requires two arguments:
- The
index
where you want to set the new value - The
element
you want to set in the list
Here’s how to use the set()
method:
val myArrayList = arrayListOf(1, 2, 3, 44, 99)
myArrayList.set(2, 88)
println(myArrayList) // [1, 2, 88, 44, 99]
Alternatively, you can also replace the set
call with indexing operator assignment as shown below:
myArrayList[2] = 88
Kotlin ArrayList get() method
The get()
method of the ArrayList
class allows you to fetch an element at a certain index from your list.
You need to specify the index as an argument to the method as shown below:
val myArrayList = arrayListOf(1, 2, 3, 44, 99)
val element = myArrayList.get(3)
println(element) // 44
Just like the set()
method, you can also replace the get
method call with indexing operator assignment as follows:
val element = myArrayList[3]
And that’s how you can fetch a specific element from an ArrayList
variable.
For more information on the ArrayList
class, you can visit Kotlin ArrayList documentation.