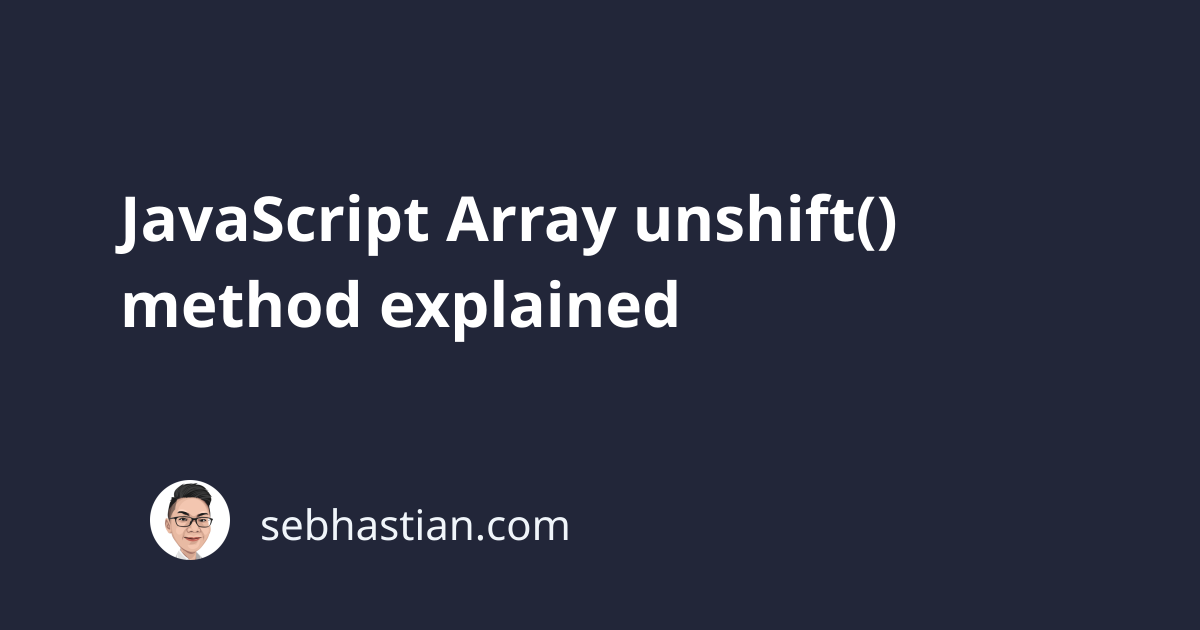
The JavaScript unshift()
method is a built-in method of JavaScript Array
wrapper object that you can use on any array type data to add new elements to an array from the beginning of the array.
The most common way to add new elements to an array is to use the push()
method, which adds new elements from the last index. Notice how the push()
method insert the element "horse"
next to "cat"
:
let animals = ["dog", "cat"];
animals.push("horse");
console.log(animals); // ["dog", "cat", "horse"]
By contrast, the unshift()
method will add "horse"
element before "dog"
as shown below:
let animals = ["dog", "cat"];
animals.unshift("horse");
console.log(animals); // ["horse", "dog", "cat"]
The unshift()
method can insert as many new elements as you need. You just need to pass all the elements you want to insert from the beginning index as its arguments:
let animals = ["dog", "cat"];
animals.unshift("horse", "fish", "lion");
console.log(animals); // ["horse", "fish", "lion", "dog", "cat"]
And that will be everything you need to know about the unshift()
method. JavaScript Array
also has the shift()
method, which removes the first element from an array.