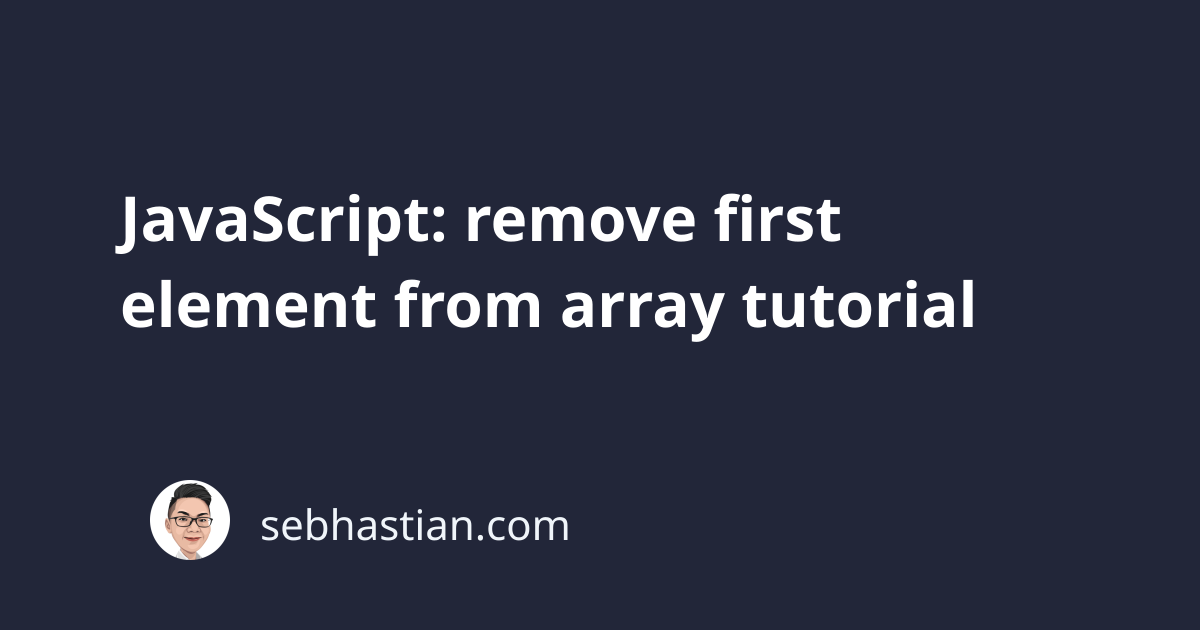
The JavaScript Array
object has a built-in method called shift()
that will remove the first element from an array for you.
To use it, you simply need to call the method with Array.shift()
syntax. You can also store the removed element in a variable if you need to.
Here’s an example:
let flowers = ["Rose", "Lily", "Tulip", "Orchid"];
// remove the first element with shift
let removedFlowers = flowers.shift();
console.log(flowers); // ["Lily", "Tulip", "Orchid"]
console.log(removedFlowers); // "Rose"
If you don’t need the removed element anymore, you can call the flowers.shift()
method without assigning the returned value to a variable:
let flowers = ["Rose", "Lily", "Tulip", "Orchid"];
// remove the first element with shift
flowers.shift();
console.log(flowers); // ["Lily", "Tulip", "Orchid"]
And that’s the easiest way you can remove the first element from an array in JavaScript.
See also: Various methods to remove elements from a JavaScript array