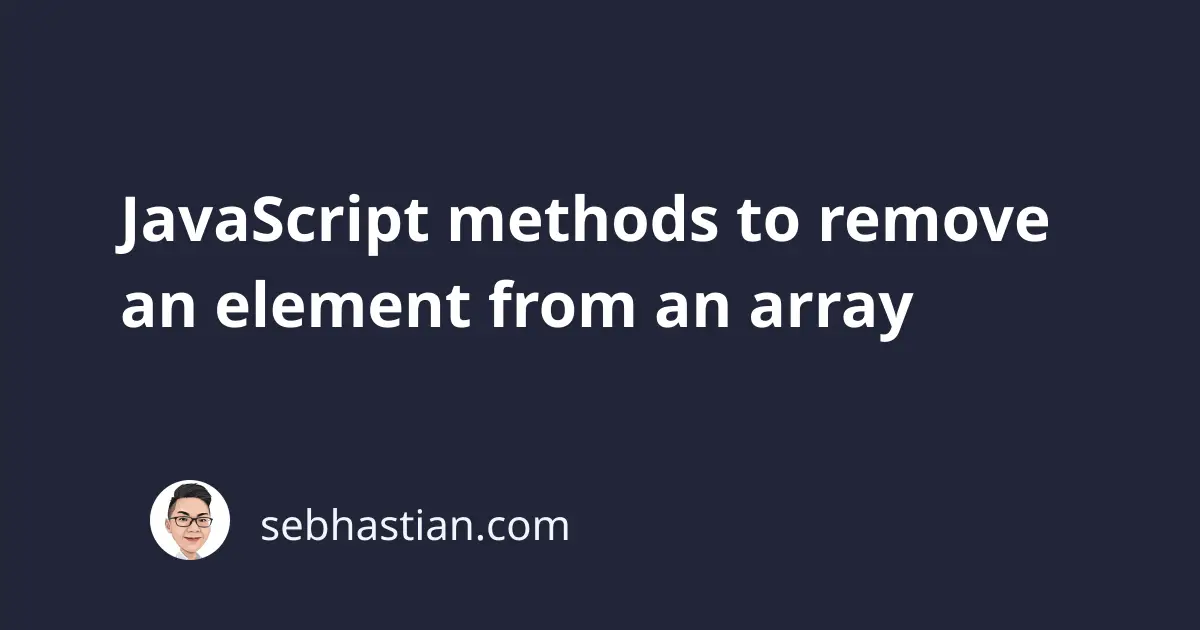
Removing one or more elements from an array is one of the most common JavaScript tasks that you may find as you code your web application. This tutorial will show you 7 ways you can remove an element from an array, with snippets that you can copy to your code.
Remove the last element with pop method
The pop
method is a built-in JavaScript method that will remove the last element from an array:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
animals.pop();
console.log(animals); // ["Lion", "Tiger", "Elephant"]
This method also returns the last element, so you can also use it just to grab the last element:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
let lastElement = animals.pop(); // "Monkey"
But in real projects, it’s more common to use pop simply to remove the last element. The second use case is nice to keep in mind though.
Remove the first element with shift method
The shift
method behaves exactly like pop
method, except that it removes the first element instead of the last:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
animals.shift();
console.log(animals); // ["Tiger", "Elephant", "Monkey"]
shift
also returns the first element like pop
:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
let firstAnimal = animals.shift(); // "Lion"
Using delete method
You can also use delete
method to remove an element in a specific index from an array:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
delete animals[2];
console.log(animals); // ["Lion", "Tiger", empty, "Monkey"]
When you log the array after delete
operation, the array length is not reduced and the element that you deleted will leave an empty slot. But although the empty slot is not removed, any array method like map
and forEach
will skip over that empty slot:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
delete animals[2];
animals.forEach((val) => console.log(val));
> "Lion"
> "Tiger"
> "Monkey"
Now if you want to remove the empty slot and reduce the length, you need to use another method.
Using splice method to update the index
The splice
method is used to remove and add new elements at the same time, depending on what arguments you passed into it. The method itself has three parameters:
startIndex
where splice will begin its operation, beginning from0
deleteCount
- an integer representing how many elements to delete from the start index. You can pass0
as well....elements
- elements that you want to add to the array beginning from thestartIndex
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
animals.splice(2, 1);
console.log(animals); // ["Lion", "Tiger", "Monkey"]
If you want to add new elements, you can simply pass the elements after the deleteCount
parameter. The following example will delete "Elephant"
at index 2
:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
animals.splice(2, 1, "Bird", "Fish");
console.log(animals); // ["Lion", "Tiger", "Bird", "Fish", "Monkey"]
The element at index 2
will be replaced with "Monkey"
, then new elements "Bird"
and "Fish"
will be added before "Monkey"
.
But if you just want to remove elements, then you only need to add the first two arguments.
Removing elements with filter method.
You can use the filter
method to remove elements from an array. The key is you need to return only elements that don’t match the element you want to remove:
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
let filterAnimals = animals.filter(function (currentElement) {
return currentElement !== "Elephant";
});
console.log(filterAnimals); // ["Lion", "Tiger", "Monkey"]
Creating your own custom functions
To avoid repeating yourself when deleting elements from an array, you can create your own custom functions for deleting an element:
function arrayRemoveOnce(array, element) {
elementIndex = array.indexOf(element);
if (elementIndex >= 0) {
array.splice(elementIndex, 1);
}
return array;
}
let animals = ["Lion", "Tiger", "Elephant", "Monkey"];
let filteredAnimals = arrayRemoveOnce(animals, "Tiger");
console.log(filteredAnimals);
Or you can remove multiple elements with the filter
method:
function arrayRemoveAll(array, element) {
return array.filter(function(value){
return value !== element
});
}
let animals = ["Lion", "Tiger", "Elephant", "Monkey", "Tiger"];
let filteredAnimals = arrayRemoveAll(animals, "Tiger");
console.log(filteredAnimals);
You can use any of these methods as you see fit.