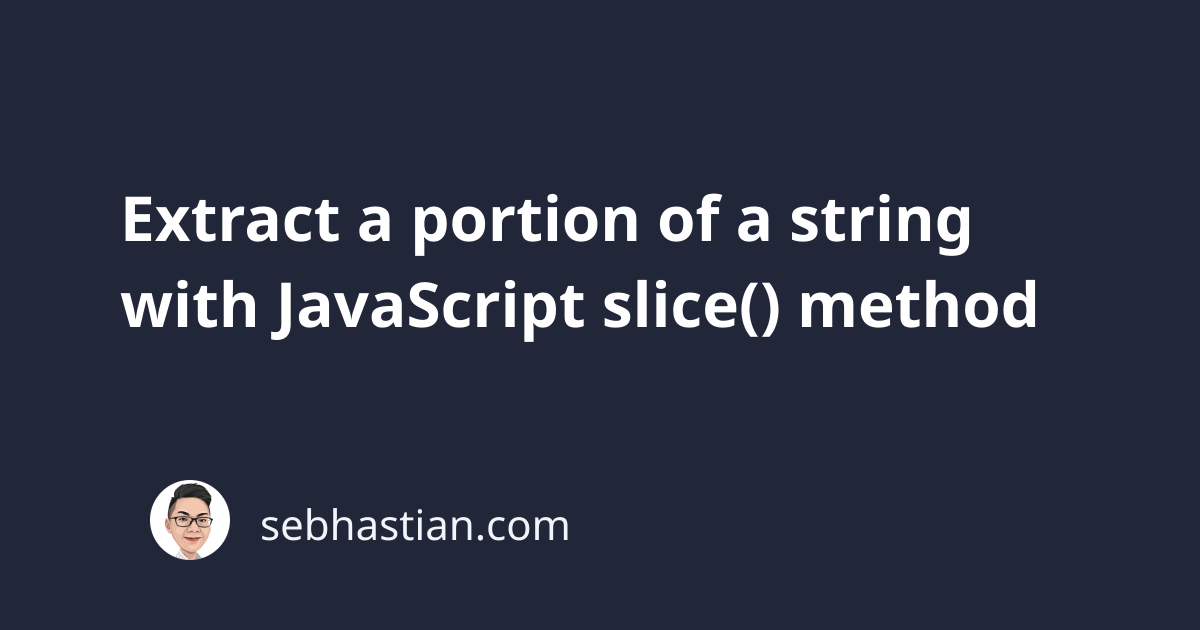
The JavaScript String
object comes with a slice()
method that you can use to extract a part of a string.
The method accepts two parameters:
- The
start
position of the portion innumber
- required - The
end
position of the portion innumber
- optional
Here’s an example of extracting a string using the slice()
method:
"Hello World!".slice(0, 5);
// Returns: 'Hello'
"ABCDEFGHIJ".slice(0, 3);
// Returns: 'ABC'
The index count for the string characters starts from zero.
The index you specify as the end
position of the slice()
method is excluded from the returned value.
You can also omit the end
parameter to extract the string up to the last character:
"Hello World!".slice(6);
// Returns: 'World!'
"ABCDEFGHIJ".slice(5);
// Returns: 'FGHIJ'
You can also use negative numbers to select a portion from the end of the string.
The indexing of the string goes like this:
0 1 2 3 4
H e l l o
-5 -4 -3 -2 -1
Let’s see some examples of extracting from the end of the string.
Note that even when you use negative numbers for the positions, the slicing process still goes from left to right:
"Good morning!".slice(-8);
// Returns: 'morning!'
"Good morning!".slice(-13, -9);
// Returns: 'Good'
"Good morning!".slice(-1, -5);
// Returns: ''
In the last example above, the slicing parameters -1
and -5
return an empty string because the indices are out of bounds.
The slice()
method always returns an empty string when it can’t perform the extraction.
The same applies when you pass an index that’s greater than the available string length:
"Hello".slice(20);
// Returns: ''
Finally, the slice()
method returns a new copy of the string value.
Changing the sliced string won’t change the original string:
let greetings = "Good morning!";
let sliced = greetings.slice(0, 4);
sliced = "Bad";
console.log(greetings); // 'Good morning!'
console.log(sliced); // 'Bad'
And that’s how you use the slice()
method to extract a part of a string.
The slice()
method is also available for the Array
object:
JavaScript Array slice() method
I hope this tutorial has been useful for you. 😉