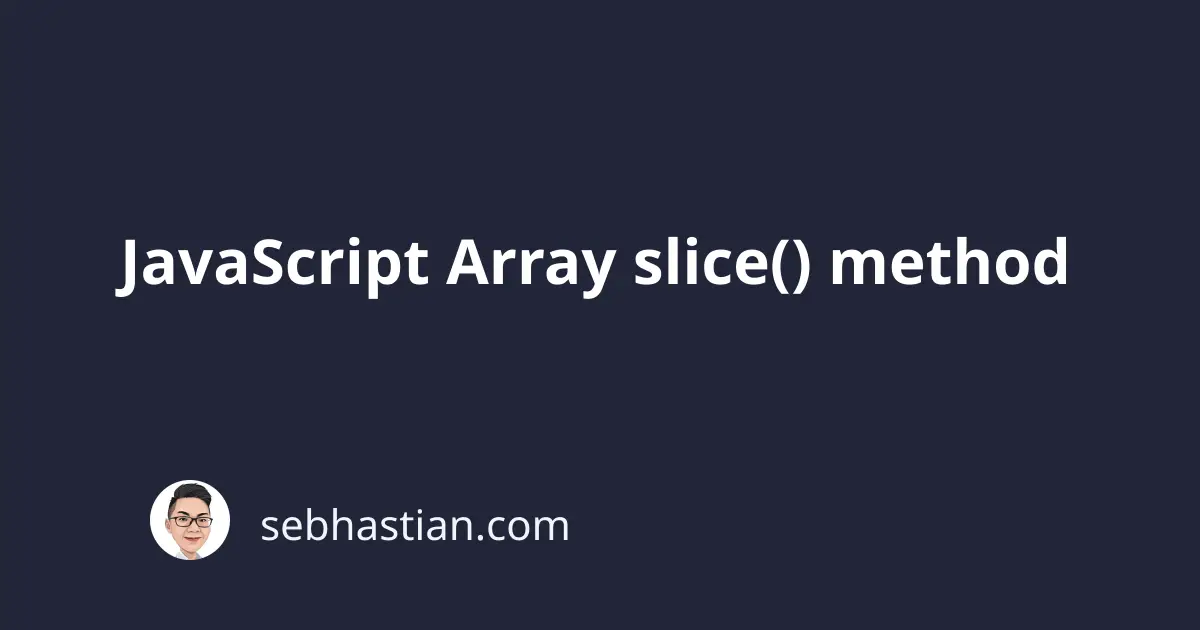
The array slice
method is used to return a portion of an array elements. This method won’t change the original array but will create a new array instead.
array.slice(startIndex, endIndex);
It has two parameters:
- The
startIndex
(optional) is the index number that specifies where to start taking the elements from the array - The
endIndex
(optional) is the index number that specifies where to end the extract.
The slice
method will stop right before the endIndex
index number, meaning if you want to stop at index 2
, you need to write 3
as the endIndex
argument.
Here’s an example of slicing array values at index 1
and 2
. Remember that an array index starts at 0
:
let colors = ["red", "blue", "yellow", "green", "gold"];
let sliced = colors.slice(1, 3);
console.log(sliced);
// output is ["blue", "yellow"]
You can omit the second parameter, which means slice
method will copy from the startIndex
argument toward the end of the array:
let colors = ["red", "blue", "yellow", "green", "gold"];
let sliced = colors.slice(2);
console.log(sliced);
// output is ["yellow", "green", "gold"]
If you omit the first parameters, slice
will start from index 0
. Both parameters are optional, and you will receive a perfect copy of the original array when you omit both parameters.
let colors = ["red", "blue", "yellow", "green", "gold"];
let sliced = colors.slice();
console.log(sliced);
// output is ["red", "blue", "yellow", "green", "gold"]
Finally, you can use negative numbers to slice
the array from the last element instead of the first. The index -1
equals to the second-to-last element of the array:
let colors = ["red", "blue", "yellow", "green", "gold"];
let sliced = colors.slice(-3, -1);
console.log(sliced);
// ["yellow", "green"]
Keep in mind that the endIndex
index argument must always be greater than the startIndex
index argument. The slice
method will simply return an empty array when the parameters are wrong instead of throwing an error:
let colors = ["red", "blue", "yellow", "green", "gold"];
let sliced = colors.slice("a", "n");
console.log(sliced); // []
And that’s all for the slice
method.