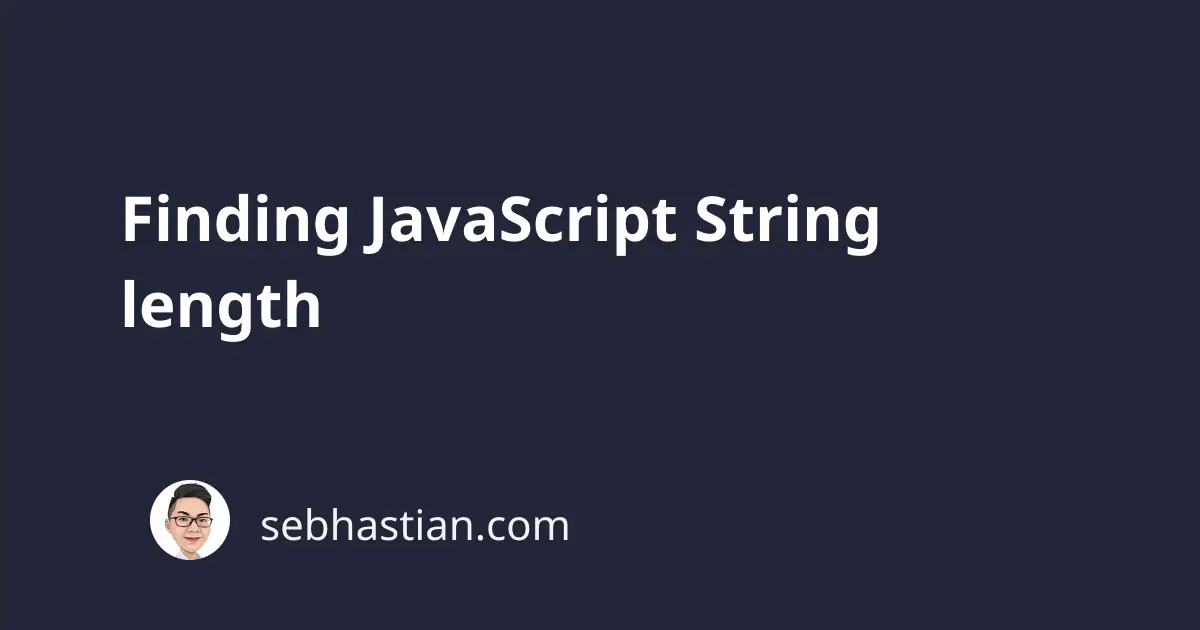
When you need to find the length of a JavaScript string, you can use the .length
property, which is available for any JavaScript string
you have in your code.
The following .length
property value returns 11
because the string
is 11 characters long:
let str = "Hello World";
console.log(str.length); // 11
You can even use it directly to any string
you encounter:
console.log("My name is Jack".length); // 15
This is because JavaScript automatically converts string
primitives to String
objects (with capital “S”), so you can call String
object methods on it directly.
The .length
property will return 0
for empty strings:
console.log("".length); // 0
A JavaScript Array
also has the .length
property that returns how many elements the Array
contains:
console.log([1, 2].length); // 2
To avoid type mismatch between an Array
and a String
, you can check the data type of your variable with typeof
operator before you get its length.
The following example assumes that the variable res
is a response from a web API call. You don’t know its type, so you check it with typeof
:
if(typeof res === "string"){
console.log(`The length of res variable is: ${res.length} characters`);
} else {
console.log("Variable res is not a string!");
}
See also: Checking types with JavaScript typeof