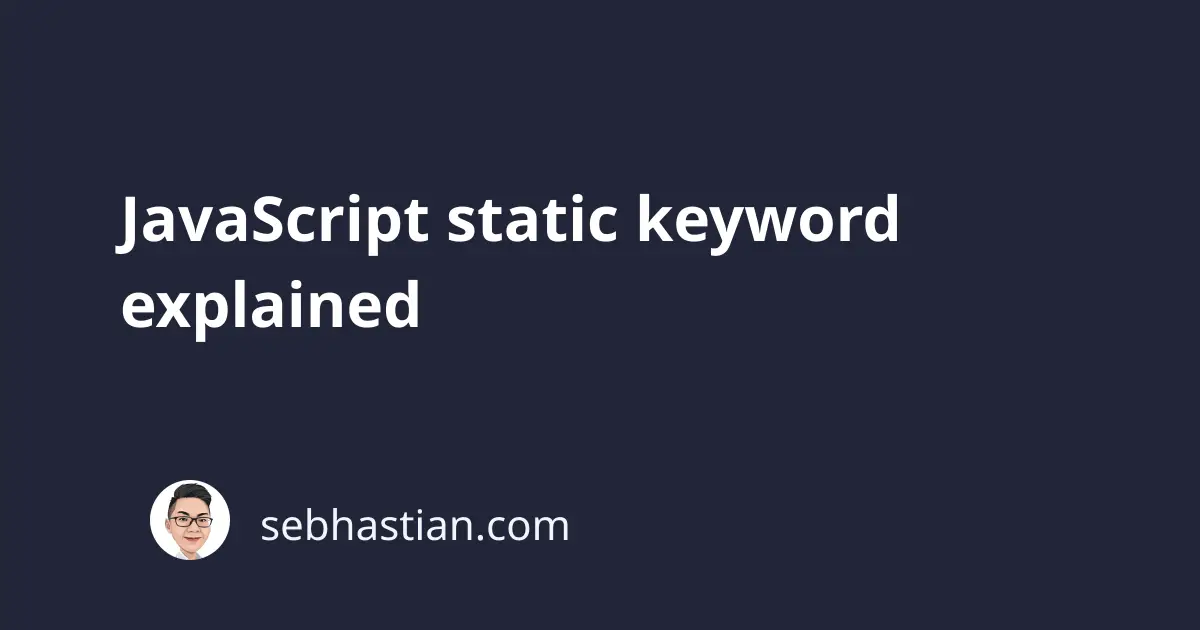
The JavaScript static
keyword is used to create a static property or method for JavaScript classes. The static
methods and properties of a class can’t be called on a class instance. It needs to be called directly from the class.
Take a look at the following example:
class Rectangle {
constructor(height, width) {
this.height = height;
this.width = width;
}
static displayName = "StaticName";
static print(){
return "Static method print() is called from Rectangle";
}
}
console.log(Rectangle.displayName); // "StaticName"
console.log(Rectangle.print()); // "Static method print() is called from Rectangle"
Calling static
property from a class instance will return undefined
:
let rec = new Rectangle(2, 3);
console.log(rec.displayName); // undefined
console.log(rec.print()); // rec.print is not a function
While calling a static
method from a class instance will throw an error:
let rec = new Rectangle(2, 3);
console.log(rec.print()); // rec.print is not a function
The static
keyword is generally used to create fixed configurations or utility functions. The best example of static
usage in JavaScript is the Math
class, which allows you to perform many mathematical tasks without having to create your own methods.