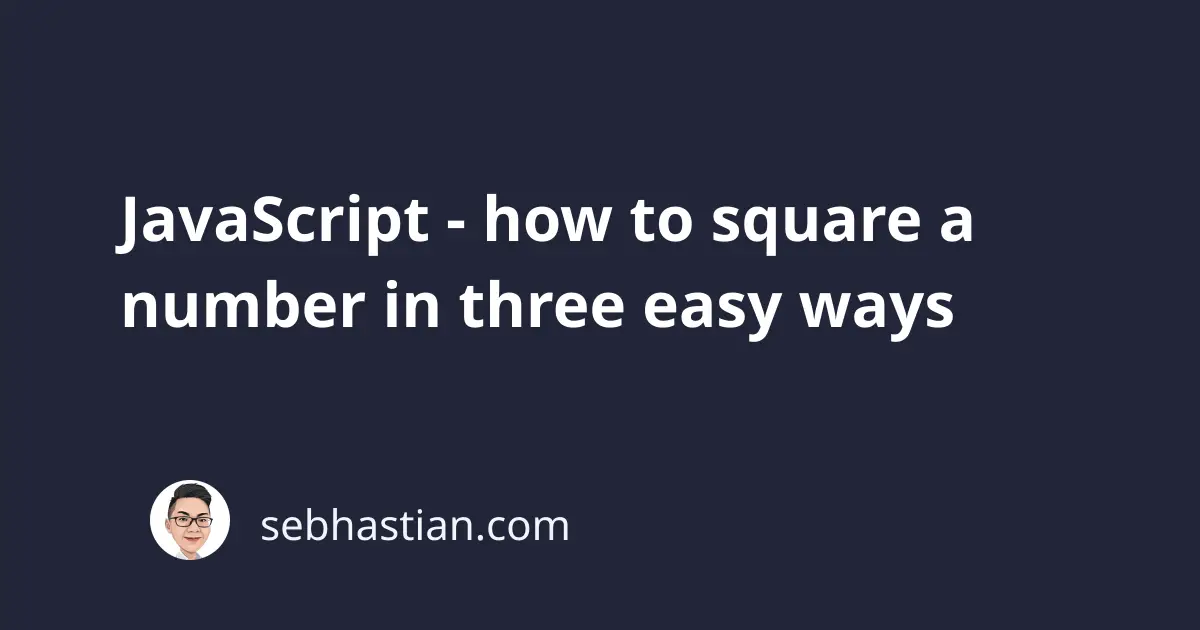
A square number is a number that you get as a result of multiplying a number by itself. For example, 4
is a square number of 2
because 4 = 2*2
.
This article will show you the three easy ways to square a number using JavaScript. The three different ways you can square a number are as follows:
- Using the
Math.pow()
method - Using the exponentiation operator (
**
) - Using a custom helper function
square()
Let’s begin with using the Math.pow()
method.
Square a number using Math.pow() method
The Math.pow()
method is used to find the exponent value of a number. The exponent value of a number is simply the number itself multiplied as many as X times by an exponent number.
Math.pow(number, exponent);
For example, The result of 2
exponent of 4
is 2*2*2*2 = 16
let num = Math.pow(2, 4);
console.log(num); // 16
By using the Math.pow()
method, you can easily find the square of a number by passing the number 2
as your exponent
parameter.
Here’s an example of finding the square of the number 6
, which is 36
:
let num = Math.pow(6, 2);
console.log(num); // 16
And that’s how you find the square of a number using Math.pow()
method. Let’s see how you can use the exponentiation operator next.
Square a number using the exponentiation operator
JavaScript provides you with the exponentiation operator marked by two asterisk symbols (**
) like this:
let num = 2 ** 3 // 2*2*2 = 8
By using the exponentiation operator, you can easily square any number you want.
Square a number by creating your own helper function
Finally, you can also find the square a number by writing a small helper function as follows:
function square(num){
return num * num;
}
By writing a function like square()
above, you can call the function anytime you need to find the square of a number:
console.log(square(2)); // 4
console.log(square(5)); // 25
console.log(square(8)); // 64
This way, you can reduce repetition in your code and make it cleaner.
Bonus: Finding the square root of a number using JavaScript
Sometimes, you may need to find the square root of a number instead of the square. A square root number is a number that will produce the square number when it’s multiplied by itself:
root * root = square;
You can find the square root of a number easily by using the JavaScript Math.sqrt()
method which accepts the square number as its argument and return the square root number.
Suppose you have the square number of 9
, you can use the Math.sqrt()
method to find the square root number, which is 3
. Take a look at the code below:
let root = Math.sqrt(9);
console.log(root); // 3
Now you’ve learned both how to square a number and how to find the square root of a number using JavaScript. Congratulations! 😉