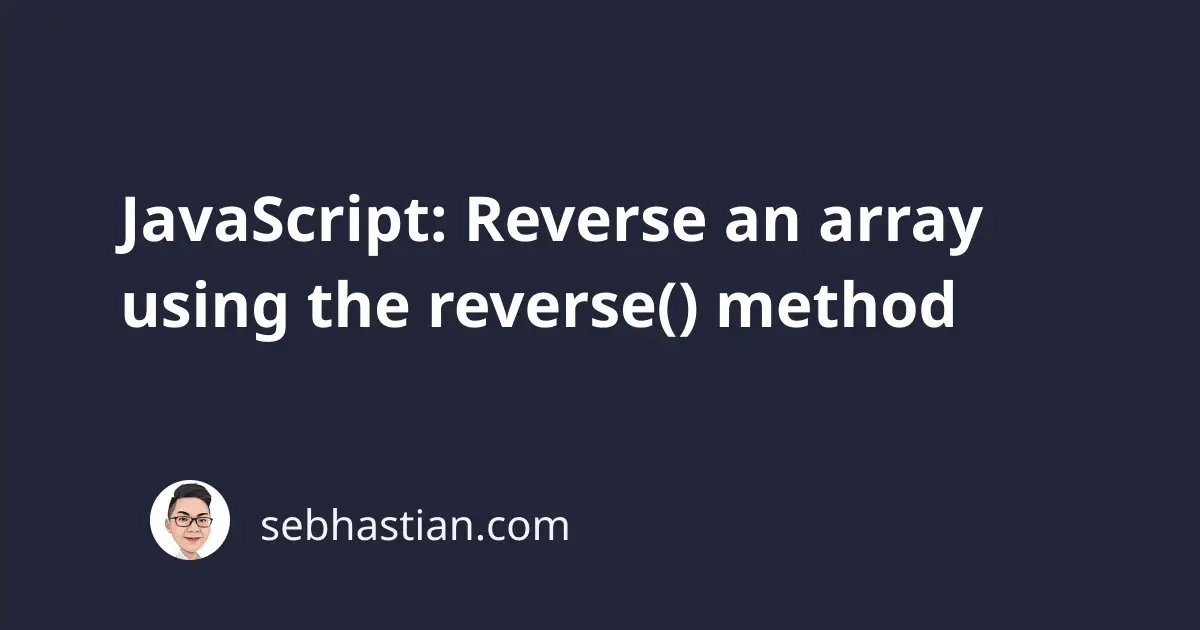
To reverse an array in JavaScript, you can call the Array reverse()
method.
The reverse()
method can be called from any valid array object you defined in your code:
const myArr = [1, 2, 3];
const reverseArr = myArr.reverse();
console.log(reverseArr); // [3, 2, 1]
console.log([10, 11, 12].reverse()); // [12, 11, 10]
But keep in mind that the reverse()
method mutates the original array where you called this method:
const myArr = [1, 2, 3];
const reverseArr = myArr.reverse();
console.log(reverseArr); // [3, 2, 1]
console.log(myArr); // [3, 2, 1]
To keep the original array unchanged, you need to create a shallow copy of the array using the slice()
method first.
You can chain the method call as shown below:
const myArr = [1, 2, 3];
const reverseArr = myArr.slice().reverse();
console.log(reverseArr); // [3, 2, 1]
console.log(myArr); // [1, 2, 3]
By using the slice()
method, the elements in myArr
won’t be mutated by the reverse()
method.
Manually reversing an array
If you need to reverse an array manually without using the reverse()
method, then the most basic way is to use the for
loop to iterate over your array.
You need to start the loop from the end of the array moving to the beginning as shown below:
const myArr = [1, 2, 3, 4, 5];
const reverseArr = [];
for (let i = myArr.length; i > 0; i--) {
reverseArr.push(myArr[i - 1]);
}
console.log(reverseArr); // [5, 4, 3, 2, 1]
The iterator let i
use the array length
to determine where to start the loop.
Then, you do the loop backward as long as the value of i
is greater than 0
.
In each iteration, you call the push()
method to put the original array elements into the reversed array variable.
When finished, you’ll have the reverseArr
populated with the original array elements in reverse.
And that’s how you reverse an array in JavaScript. Good work! 😉