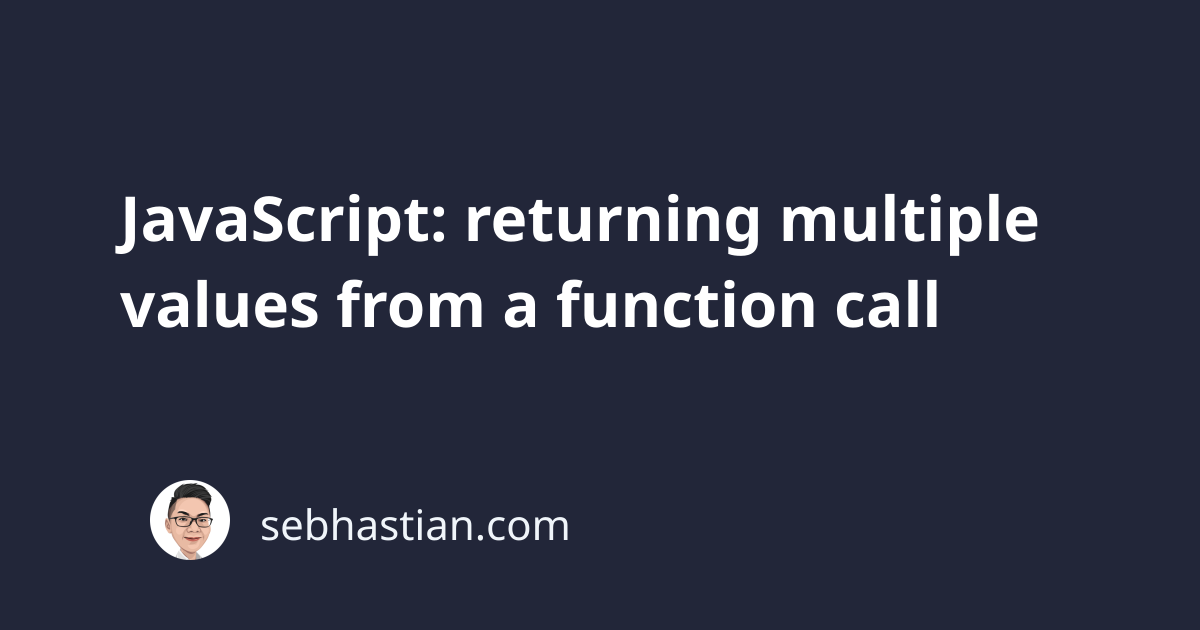
A JavaScript function can only return
a single value:
function getUser() {
const name = "Nathan";
return name;
}
But there will be times when you need to return multiple values from a function. Suppose that the getUser
function from the example above needs to return both name
and role
value. You can’t return multiple values as follows:
function getUser() {
const name = "Nathan";
const role = "Web Developer";
return name, role;
}
const user = getUser();
console.log(user); // "Web Developer"
In the example above, JavaScript will return the last variable role
and ignore the previous variables you write down next to the return
statement.
When you need to return multiple values, you need to wrap the values in an array
as shown below:
function getUser() {
const name = "Nathan";
const role = "Web Developer";
return [name, role];
}
const user = getUser();
console.log(user[0]); // "Nathan"
console.log(user[1]); // "Web Developer"
Or you can also use put the values as properties of an object
like this:
function getUser() {
const name = "Nathan";
const role = "Web Developer";
return { name, role };
}
const user = getUser();
console.log(user.name); // "Nathan"
console.log(user.role); // "Web Developer"
By wrapping your values using either an array
or an object
, you can return as many values as you need from a JavaScript function
.
Finally, you can also extract the returned values by using a destructuring assignment as follows:
function getUser() {
const name = "Nathan";
const role = "Web Developer";
return [name, role];
}
const [name, role] = getUser();
console.log(name); // "Nathan"
console.log(role); // "Web Developer"
Notice how two variables in a square bracket are assigned the value returned by getUser()
call. You can call the variables using the name you assign with destructuring assignment instead of using user[0]
and user[1]
.
The same is also possible with object
data type:
function getUser() {
const name = "Nathan";
const role = "Web Developer";
return { name, role };
}
const { name, role } = getUser();
console.log(name); // "Nathan"
console.log(role); // "Web Developer"
Learn more about JavaScript destructuring assignment in this tutorial.
And that’s how you can return multiple values from a function call in JavaScript. Since the return
statement can only return a single value, you can wrap multiple values in an array
or an object
before returning it to the caller.