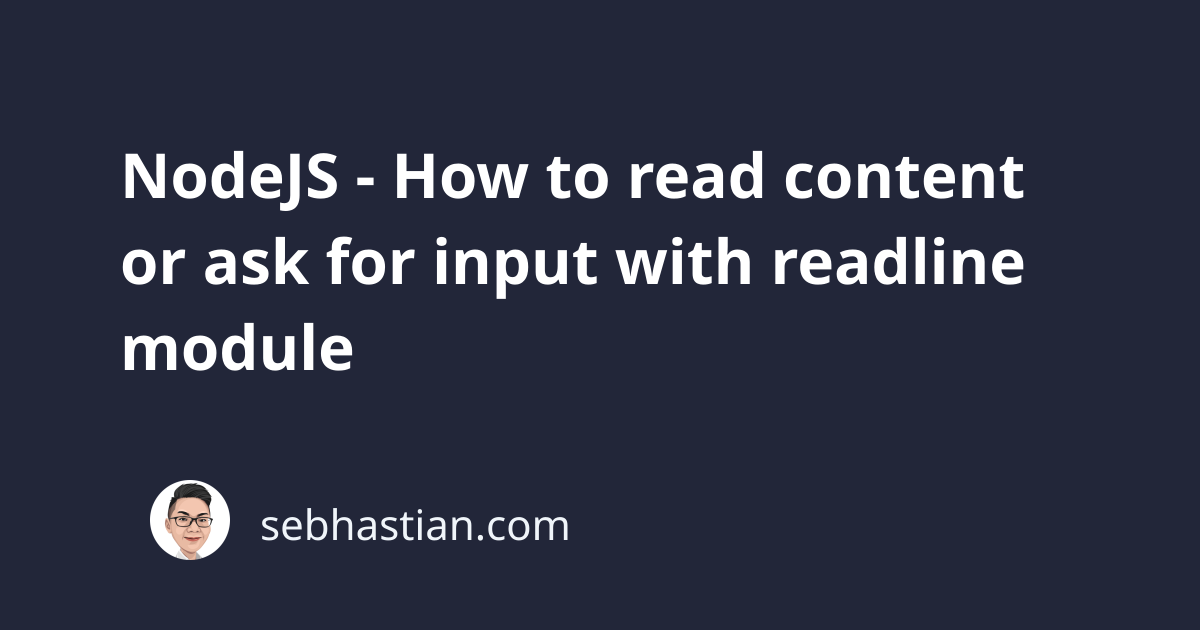
The readline
module in NodeJS provides you with a way to read data stream from a file or ask your user for an input.
To use the module, you need to import it to your JavaScript file as follows:
const readline = require('readline');
Next, you need to write the code for receiving user input or reading file content, depending on your requirement. Let’s look at how you can receive user input first.
You can see the full code for this tutorial in GitHub
Using readline to receive user input
To receive user input using readline
module, you need to create an Interface
instance that is connected to an input stream. You create the Interface
using readline.createInterface()
method, while passing the input
and output
options as an object argument.
Here’s an example of creating the readline interface:
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('What is your name? ', (answer) => {
console.log(`Oh, so your name is ${answer}`);
});
Both process.stdin
and process.stdout
means input and output is connected to the terminal.
Once created, the interface will be active until you close it by triggering the 'close'
event, which can be triggered by writing the code in your JavaScript file, or using
CTRL +
D or
CTRL +
C command.
To ask for user input, you need to call the question()
method from the Interface
instance, which is assigned to rl
variable on the code above.
The question()
method receives two parameters:
- The
string
question you want to ask your user - The
options
object (optional) where you can pass the'abort'
signal - The
callback
function to execute when the answer is received, passing theanswer
to the function
You can skip the options
object and pass the callback
function as the second parameter.
Here’s how you use question()
the method:
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('What is your name? ', (answer) => {
console.log(`Oh, so your name is ${answer}`);
});
Finally, you can close the rl
interface by calling the rl.close()
method inside the callback function:
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('What is your name? ', (answer) => {
console.log(`Oh, so your name is ${answer}`);
console.log("Closing the interface");
rl.close();
});
Save the file as ask.js
, then call the script using NodeJS like this:
$ node ask.js
What is your name? Nathan
Oh, so your name is Nathan
Closing the interface
$
And that’s how you can ask for user input using NodeJS readline
module.
You can also use the AbortController()
from NodeJS to add a timer to your question and cancel it when a certain amount of time has passed.
But please be aware that the AbortController()
method is only available for NodeJS version 15 and up. And even then, the method is still experimental.
The following question will be aborted when no answer was given in 5 seconds after the prompt. The code has been tested to work on NodeJS version 16.3.0 and up:
const readline = require("readline");
const ac = new AbortController();
const signal = ac.signal;
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
rl.question("What is your name? ", { signal }, (answer) => {
console.log(`Oh, so your name is ${answer}`);
console.log("Closing the console");
process.exit();
});
signal.addEventListener(
"abort",
() => {
console.log("The name question timed out!");
},
{ once: true }
);
setTimeout(() => {
ac.abort();
process.exit();
}, 5000); // 5 seconds
The rl.close()
method is replaced with process.exit()
method because the readline interface will still wait for the abort
signal to close the interface if you use the rl.close()
method.
Using readline to read file content
To read file content using readline
module, you need to create the same interface using createInterface()
command, but instead of connecting the input
option to the process.stdin
object, you connect it to the fs.createReadStream()
method.
Take a look at the following example:
const readline = require("readline");
const fs = require("fs");
const path = "./file.txt";
const rl = readline.createInterface({
input: fs.createReadStream(path),
});
Next, you need to add an event listener to the 'line'
event, which is triggered everytime an end-of-line input (\n
, \r
, or \r\n
) is received from the input
stream.
You listen for the 'line'
event by using rl.on()
method:
rl.on("line", function (input) {
console.log(input);
});
Finally, let’s test the code by creating a new file named file.txt
with the following content:
Roses are red
Violets are blue
Sunflowers are yellow
Pick the flower you like the most :)
Then in the same folder, create a file named read.js
to read the file.txt
content:
const readline = require("readline");
const fs = require("fs");
const path = "./file.txt";
const rl = readline.createInterface({
input: fs.createReadStream(path),
});
let lineno = 1;
rl.on("line", function (input) {
console.log("Line number " + lineno + ": " + input);
lineno++;
});
Then execute the script using NodeJS. You’ll have the output as shown below:
$ node read.js
Line number 1: Roses are red
Line number 2: Violets are blue
Line number 3: Sunflowers are yellow
Line number 4: Pick the flower you like the most :)
$
The full code for this tutorial can be found on GitHub.
For more information, you can visit NodeJS readline
module documentation
Conclusion
The JavaScript readline
module is a module provided by NodeJS so you can create an interactive command-line program that receives user input or read file content.
This module is only available in NodeJS, so you can’t use it from the browser. If you want to read file content from the browser, you need to use the FileReader
class provided by the browser.
See also: Using FileReader
to read a CSV file from the browser tutorial.
When you need to take user inputs from the browser, you can use HTML <form>
and <input>
elements or the JavaScript prompt()
function.