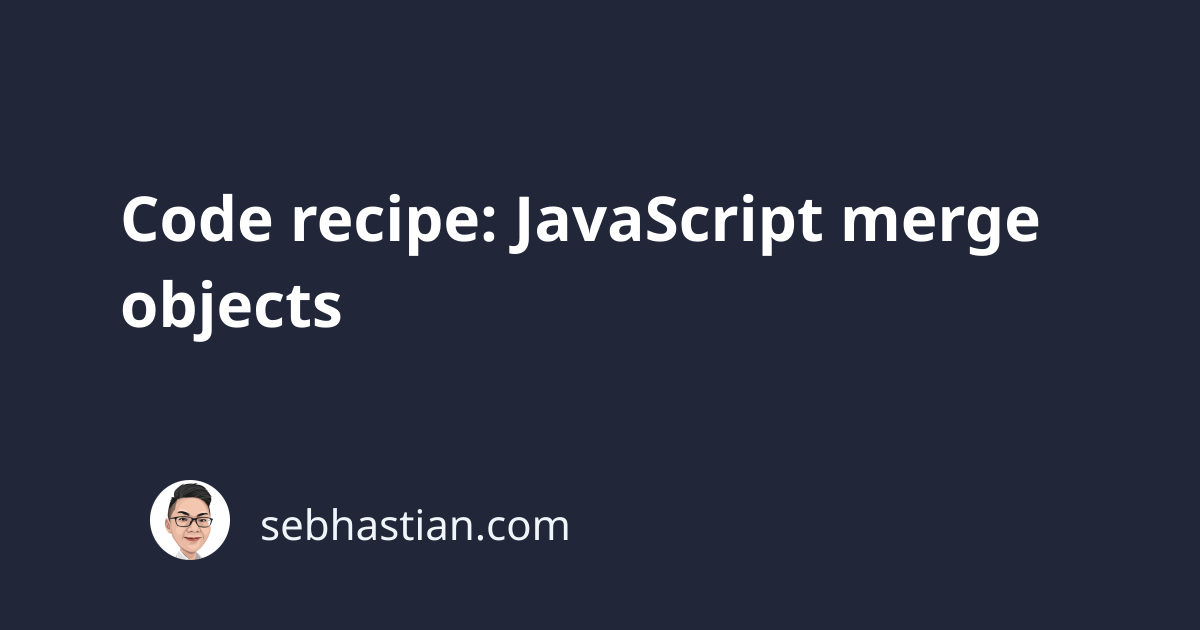
There are at least 2 ways to merge two or more JavaScript objects in JavaScript easily:
- Using spread operator
- Using
Object.assign()
method
Let’s see how to use the spread operator first:
Merging objects with the spread operator
You can use the spread operator to extract its properties into a new single property.
Here’s an example of how you can merge human
and traits
objects to create a person
object:
const human = {
name: "John",
};
const traits = {
age: 29,
hobby: "Programming computers",
};
const person = { ...human, ...traits };
The person
object will have the following properties:
{
name: "John",
age: 29,
hobby: "Programming computers"
}
Keep in mind that the properties of the previous object will be overwritten with the next object. For example, if the human
object already has the age
property, it will be overwritten by the age
property from the traits
object:
const human = {
name: "John",
age: 37,
};
const traits = {
age: 29,
hobby: "Programming computers",
};
const person = { ...human, ...traits };
console.log(person.age); // 29
The same thing happens when you have a third object that has the age
property.
Merging objects using Object.assign()
The Object.assign()
method is a built-in JavaScript Object method that allows you to copy defined properties from the source
objects into the target
object:
Object.assign(target, source);
You can pass as many source objects as you need to the method. Please take note that the Object.assign()
method will modify the target
object and return it too.
Here’s an example of creating the person
object with the assign()
method:
const human = {
name: "John",
age: 37,
};
const traits = {
age: 29,
hobby: "Programming computers",
};
const person = Object.assign(human, traits);
console.log(human); // { name: "John", age: 29, hobby: "Programming computers" }
console.log(person); // { name: "John", age: 29, hobby: "Programming computers" }
As shown in the code above, both human
and person
objects will have the same properties. If you don’t want to modify the original array, you can pass a new empty object ({}
) as the target
argument while the rest of the objects are passed as the sources:
const human = {
name: "John",
age: 37,
};
const traits = {
age: 29,
hobby: "Programming computers",
};
const person = Object.assign({}, human, traits);
console.log(person); // { name: "John", age: 29, hobby: "Programming computers" }
console.log(human); // { name: "John", age: 37 }
Reusable function to merge many objects
As you can see from the examples above, you can use both spread operator or Object.assign()
method to merge two or more objects. I will leave you with a reusable function that can merge as many objects passed into its arguments using the rest parameter and reduce()
method:
function mergeObj(...arr){
return arr.reduce((acc, val) => {
return { ...acc, ...val };
}, {});
}
const human = {
name: "John",
age: 37,
};
const traits = {
age: 29,
hobby: "Programming computers",
};
const attribute = {
age: 40,
nationality: "Belgian"
};
const person = mergeObj(human, traits, attribute);
console.log(person);
// { name: "John", age: 40, hobby: "Programming computers", nationality: "Belgian" }
You can freely use and modify the mergeObj()
function in your code.
See also: Understanding JavaScript reduce method