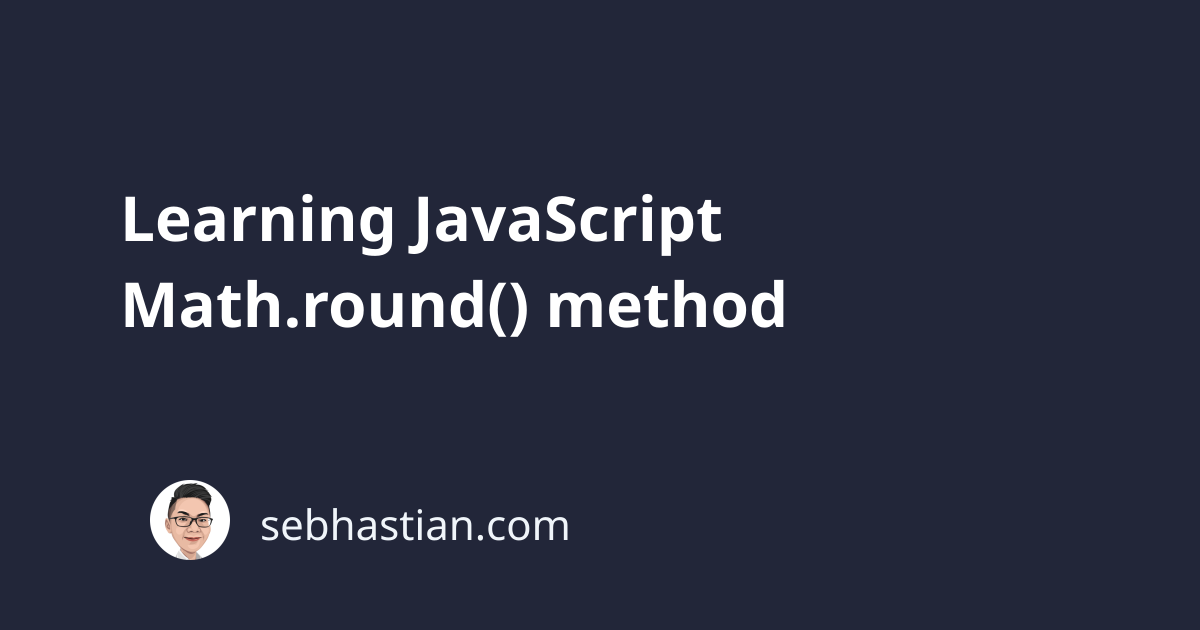
The JavaScript Math.round()
method is used to round a number with decimal values to the nearest whole number (also called an integer)
If the decimal value is .5
or higher, the method will return the higher integer:
Math.round(6.5);
// returns 7
When the decimal value is lower than .5
the method will return the lower integer:
Math.round(6.4);
// returns 6
The method only accepts one number, so if you pass multiple numbers, it will only return the first number and ignore the rest:
Math.round(4.5, 7, 8);
// returns 5
If you pass a string
, an object
, or an array
to the method, it will return NaN
which stands for Not a Number
:
Math.round("Hello there!");
Math.round([1, 2, 3]);
Math.round({ name: "Andy" });
// returns NaN
When you pass a boolean
value of true
or false
it will return 1
for true
and 0
for false
:
Math.round(true);
// returns 1
Math.round(false);
// returns 0
Other rounding methods
If you want to always round a number up, use [the Math.ceil()
method]( {{ <ref “/18-javascript-math-ceil”> }} ).
If you want to always round a number down, use the Math.floor()
method.