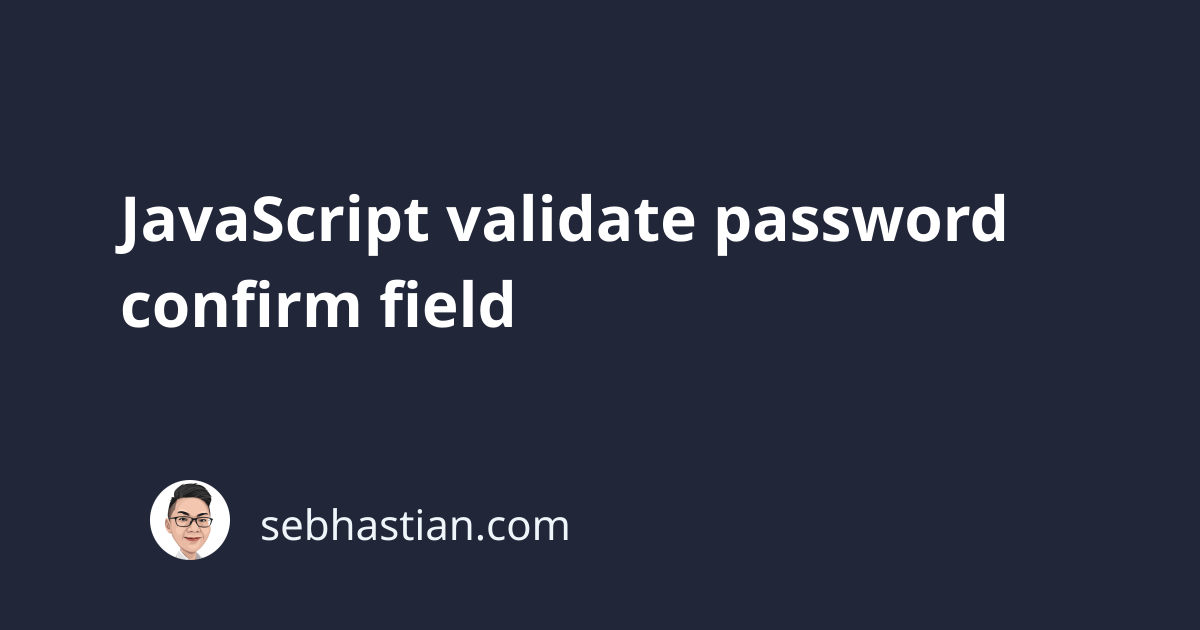
JavaScript can be used to validate if the Password and Confirm Password fields match before processing an HTML form.
This validation is usually implemented on social sites registration form to check if the user entered the right password for the new account.
There are two methods to validate Password and Confirm Password fields using JavaScript:
This tutorial will help you learn both methods mentioned above.
Let’s start with validating password confirmation when the form is submitted.
Validate password with onSubmit() form handler
To validate the password entered by your user, you need to add a JavaScript function that’s going to be called when the onSubmit
event is triggered.
Let’s create a simple example to demonstrate this:
<html>
<head>
<style>
.label {
display: inline-block;
width: 200px;
}
.form-group {
margin-bottom: 1rem;
}
</style>
</head>
<body>
<h1>Password confirm in JavaScript</h1>
<form method="POST">
<div class="form-group">
<label class="label">Password: </label>
<input type="password" name="password" required />
</div>
<div class="form-group">
<label class="label">Confirm Password: </label>
<input type="password" name="confirmPassword" required />
</div>
<div><input type="submit" value="Submit" /></div>
</form>
</body>
</html>
First, create a checkPassword()
function that will be called by the form.
This function will check whether the Password and Confirm Password fields are filled.
When both are filled, it will check whether the input values match or not.
Put the function in a <script>
tag above the closing </body>
tag as shown below:
<script>
// 👇 pass the form into the function as a parameter
function checkPassword(form) {
// 👇 get passwords from the field using their name attribute
const password = form.password.value;
const confirmPassword = form.confirmPassword.value;
// 👇 check if both match using if-else condition
if (password != confirmPassword) {
alert("Error! Password did not match.");
return false;
} else {
alert("Password Match. Congratulations!");
return true;
}
}
</script>
Finally, you only need to add the checkPassword()
function to the form using the onSubmit
attribute.
Here’s the complete code for on submit validation:
<html>
<head>
<style>
.label {
display: inline-block;
width: 200px;
}
.form-group {
margin-bottom: 1rem;
}
</style>
</head>
<body>
<h1>Password confirm in JavaScript</h1>
<form onSubmit="return checkPassword(this)" method="POST">
<div class="form-group">
<label class="label">Password: </label>
<input type="password" name="password" required />
</div>
<div class="form-group">
<label class="label">Confirm Password: </label>
<input type="password" name="confirmPassword" required />
</div>
<div><input type="submit" value="Submit" /></div>
</form>
<script>
function checkPassword(form) {
const password = form.password.value;
const confirmPassword = form.confirmPassword.value;
if (password != confirmPassword) {
alert("Error! Password did not match.");
return false;
} else {
alert("Password Match. Congratulations!");
return true;
}
}
</script>
</body>
</html>
The form will only be submitted when the onSubmit
event returns true
.
Here’s an example of the alert when you run the code:
And that’s how you validate a Confirm Password field.
Next, let’s see how you can validate password match using the
Validate password with addEventListener() handler
You can also validate confirm password field as the user types into the Confirm Password field.
First, you need to add an element to the form that will display feedback whenever the Confirm Password field did not match the Password field.
Edit the HTML form with the highlighted changes below:
<form id="myForm" method="POST">
<div class="form-group">
<label class="label">Password: </label>
<input type="password" name="password" required />
</div>
<div class="form-group">
<label class="label">Confirm Password: </label>
<input type="password" name="confirmPassword" required />
<small id="confirmPassword-feedback"></small>
</div>
<div><input type="submit" value="Submit" /></div>
</form>
Also, add a little styling to the <small>
tag above as follows:
#confirmPassword-feedback {
color: red;
}
Next, create a script that will get the form above using the getElementById()
function.
You can grab the form’s <input>
values like this:
const form = document.getElementById("myForm");
const password = form.password;
const confirmPassword = form.confirmPassword;
const feedback = document.getElementById("confirmPassword-feedback");
let isPasswordMatch = false;
Next, attach an event listener to the confirmPassword
field using the addEventListener()
function as follows:
confirmPassword.addEventListener("input", () => {
if (password.value != confirmPassword.value) {
feedback.innerHTML = "Password did not match.";
isPasswordMatch = false;
} else {
feedback.innerHTML = "";
isPasswordMatch = true;
}
});
Finally, add an onsubmit
handler to the form. When the isPasswordMatch
value is true
, submit the form.
Else, you need to cancel the form submission. You can use the alert()
function to notify the user:
form.onsubmit = function () {
if (isPasswordMatch) {
alert("Form submitted. Great!");
return true;
}
alert("Wait! Password did not match.");
return false;
};
Now the form is finished. The full code for the form is as follows:
<html>
<head>
<style>
.label {
display: inline-block;
width: 200px;
}
.form-group {
margin-bottom: 1rem;
}
#confirmPassword-feedback {
color: red;
}
</style>
</head>
<body>
<h1>Password confirm in JavaScript</h1>
<form id="myForm" method="POST">
<div class="form-group">
<label class="label">Password: </label>
<input type="password" name="password" required />
</div>
<div class="form-group">
<label class="label">Confirm Password: </label>
<input type="password" name="confirmPassword" required />
<small id="confirmPassword-feedback"></small>
</div>
<div><input type="submit" value="Submit" /></div>
</form>
<script>
const form = document.getElementById("myForm");
const password = form.password;
const confirmPassword = form.confirmPassword;
const feedback = document.getElementById("confirmPassword-feedback");
let isPasswordMatch = false;
confirmPassword.addEventListener("input", () => {
if (password.value != confirmPassword.value) {
feedback.innerHTML = "Password did not match.";
isPasswordMatch = false;
} else {
feedback.innerHTML = "";
isPasswordMatch = true;
}
});
form.onsubmit = function () {
if (isPasswordMatch) {
alert("Form submitted. Great!");
return true;
}
alert("Wait! Password did not match.");
return false;
};
</script>
</body>
</html>
With that, the Confirm Password field will be validated each time you type an input.
And now you’ve learned how to validate if the Confirm Password field matches the Password field using JavaScript.
Feel free to use the code in this tutorial for your projects. I hope this tutorial has been useful for you 🙏