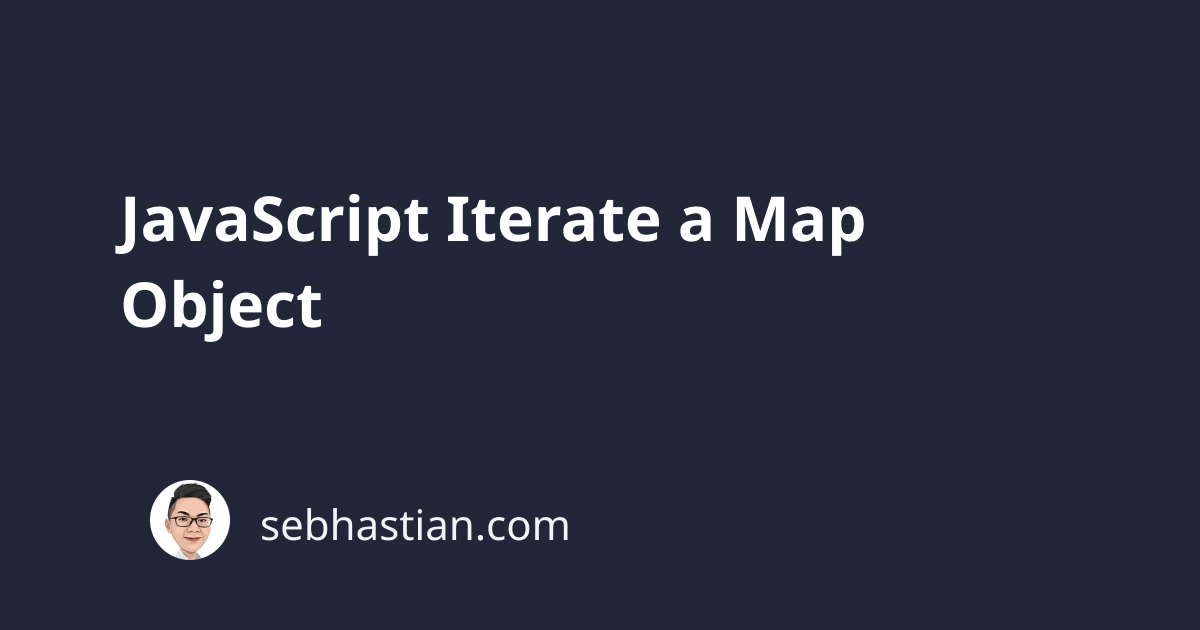
When working on a piece of JavaScript program, you might encounter a task requiring you to iterate over a Map object.
This article will help you learn how to iterate over a Map object in JavaScript using available methods from the programming language.
1. Using Map.foreach
The Map
object prototype has the forEach()
method that you can use to iterate over any Map
object you created in your program.
First, create a Map
object using the Map()
constructor as shown below:
let myMap = new Map();
myMap.set(1, 'A element');
myMap.set(2, 'B element');
myMap.set(3, 'C element');
Next, you call the forEach()
method from the myMap
object and pass a callback function that accepts the value
and key
parameters from the object like this:
myMap.forEach(function (value, key) {
console.log(key + ' = ' + value);
});
The forEach()
method will iterate over the myMap
object, passing each key-value pair to the callback function. You will see this output as the result:
1 = A element
2 = B element
3 = C element
And that’s how you iterate over a Map object in JavaScript. Easy Peasy isn’t it?
2. Using the for… of loop
Another way you can iterate over a Map object is to use the for...of
loop. Here’s how you can do it:
let myMap = new Map();
myMap.set(1, 'A element');
myMap.set(2, 'B element');
myMap.set(3, 'C element');
for (let [key, value] of myMap) {
console.log(key + ' = ' + value);
}
In the example above, we used the array destructuring assignment to capture the key-value pair extracted from the of
operator.
The variable key
and value
will contain the respective values, and in each iteration you can manipulate those values as required.
There’s almost no difference between the two methods, so you can use which one you prefer. These have the same performance for iterating over a Map object.
Conclusion
To iterate over a Map object in JavaScript, you can use either the forEach()
method defined in the Map object prototype, or the for...of
loop.
Both solutions are very capable to iterate over a Map object with no issue whatsoever. I hope this article helps!
I also have more articles related to JavaScript Map object here:
These might be useful in your journey as a JavaScript developer. Happy coding and see you again.