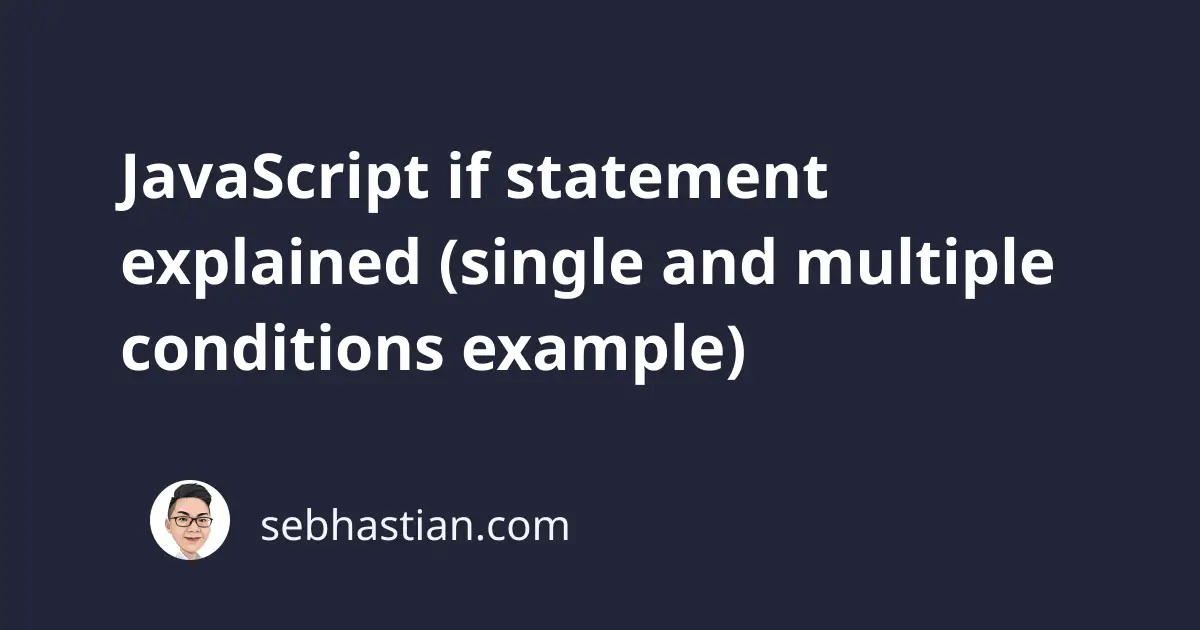
The if
statement is one of the building blocks of JavaScript programming language.
The statement is used to create a conditional branch that runs only when the condition for the statement is fulfilled.
To use the if
statement, you need to specify the condition in parentheses:
if (10 > 5) {
console.log("Ten is bigger than five");
}
When the expression inside the parentheses of the if
statement evaluates to true
, the code specified in the statement body is executed.
You define an if
statement body using the curly brackets ({}
) like a function.
In the above code, the expression of 10 > 5
evaluates to true
, so the console.log is executed by JavaScript.
When you specify an expression that evaluates to false
, the code inside the if
block won’t be executed.
Nothing happens when you run the code below:
if (10 > 70) {
console.log("Ten is bigger than seventy");
}
When you have only one line of code to run in the if
statement, you can omit the curly brackets from the statement.
Write the code you want to run next to the parentheses as shown below:
if (10 > 5) console.log("Ten is bigger than five");
The above code will run without any issue.
You can use variables as part of the condition for the if
statement as follows:
let occupation = "Programmer";
if (occupation === "Programmer") {
console.log("Your are a Programmer!");
}
You can replace the values used in the if
condition with variables and constants.
Next, let’s see how you can define multiple conditions in an if
statement.
Defining multiple conditions in Javascript if statement
Up to this point, you’ve seen how to create a JavaScript if
statement with a single condition:
if (10 > 70) {
console.log("Ten is bigger than seventy");
}
if (10 > 3) {
console.log("Ten is bigger than three");
}
When you have multiple requirements for the code to run, you can actually specify multiple conditions inside parentheses.
The code below has an if
statement with two conditions:
let num = 50;
if (num > 10 && num < 90) {
console.log("Number is between 10 and 90");
}
The AND operator (&&
) above is used to specify two conditions for the if
statement to run.
When the expressions evaluate to true
, then Javascript will execute the body of the if
statement.
You can specify as many conditions as you need using the AND or OR (||
) operator.
When using the OR operator, the code will be run if one of the conditions evaluate to true
as follows:
let num = 25;
if (num === 10 || num === 25) {
// true so code below will be executed
console.log("Number is either 10 or 25");
}
When using the AND
operator, all conditions must evaluate to true
for the code to run.
By default, JavaScript will evaluate if
statement conditions from left to right.
Depending on the result you want to have, you can modify the evaluation order by using parentheses as shown below:
let num = 20;
let str = "xyz";
// 1. No parentheses between conditions
if ((num == 25 && str == "abc") || str == "xyz") {
console.log("first if statement");
}
// 2. Parentheses for the `str` evaluation
if (num == 25 && (str == "abc" || str == "xyz")) {
console.log("second if statement");
}
In the code above, the first if
statement will be executed while the second will be ignored.
This is because the first if statement evaluates to true
with the following steps:
# first if statement
num == 25 && str == "abc" || str == "xyz"
👆 || 👆
false || true
The expression false || true
evaluates to true
so the code is executed.
In the second if
statement, the expression inside parentheses will be executed first as shown below:
# second if statement
num == 25 && (str == "abc" || str == "xyz")
👆
num == 25 && true
The expression num == 25 && true
evaluates to false
because the num
value is 20
in the example above. The second if statement is ignored because of this.
And that’s how you can specify multiple conditions with the if
statement.
You can define as many conditions as you need for your if
statement, but keep in mind that too many conditions may cause confusion.
Try to keep the conditions as minimum as you can.
Finally, you can also pair the if
statement with an else
statement.
Learn more here: JavaScript if and else statements
Great work on learning about the JavaScript if
statement. 👍