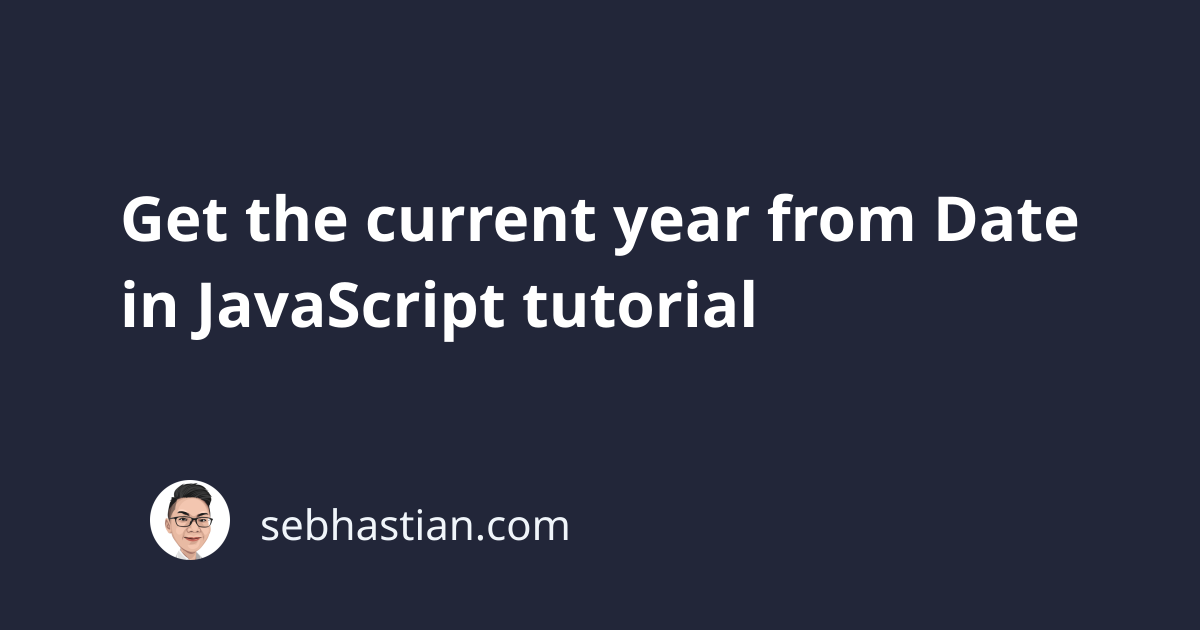
When you need to get the current year value from a JavaScript Date
object, you need to use the Date.getFullYear()
method, which returns a number
value representing the year of the date.
For example, the following Date
object will return 2021
when you call the getFullYear()
method:
const date = new Date("01/20/2021"); // 20th January 2021
const year = date.getFullYear();
console.log(year); // 2021
If you want to get the year represented by 2 digits, you can transform the returned year
value into a string
and use the substring()
method to extract the last 2 digits. Here’s an example:
const date = new Date("01/20/2021"); // 20th January 2021
const year = date.getFullYear(); // 2021
const year2digits = year.toString().substring(2);
console.log(year2digits); // "21"
You can cast the 2 digits string representing the year back into number value using the Number()
method if you need to.
Finally, you can use JavaScript to automatically update an HTML <footer>
to always show the current year. In the following example, the <script>
tag will put the year
value inside the <span>
tag:
<footer>
© <span id="year"></span>
</footer>
<script>
const date = new Date();
const year = date.getFullYear(); // 2021
document.getElementById("year").innerHTML = year;
</script>
And that’s how you can get the current year value using JavaScript.