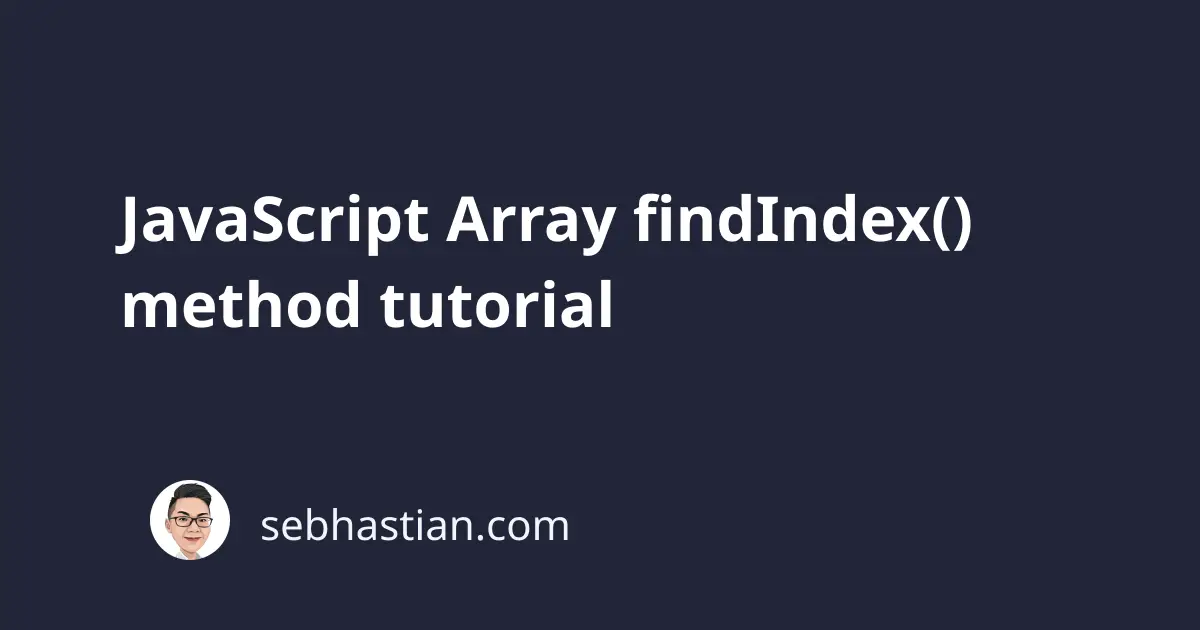
The JavaScript Array.findIndex()
method allows you to find the index of the first element that fulfills certain criteria. You are free to define the criteria in a callback function.
The following example returns the index of the first element that’s higher than 7
:
let index = [4, 5, 9, 12].findIndex(function (element) {
return element > 7;
});
console.log(index); // 2
The first element
that fulfills the element > 7
test is 9
at index 2
, so the findIndex()
method returns 2
.
If no element fulfills the criteria, findIndex()
will return -1
:
let index = [4, 5, 9, 12].findIndex(function (element) {
return element > 20;
});
console.log(index); // -1
The findIndex()
method will pass the current element
, the current index
, and the array
itself into the callback function, but usually you only need the element
:
let index = [4, 5, 9, 12].findIndex(function (element, index, array) {
console.log(element);
console.log(index);
console.log(array);
return element > 8;
});
The following will be logged to the console:
> 4
> 0
> Array [4, 5, 9, 12]
> 5
> 1
> Array [4, 5, 9, 12]
> 9
> 2
> Array [4, 5, 9, 12]
The last element 12
is not logged to the console because findIndex()
will stop the iteration after finding the first element that fulfills the criteria.
Finding indices of all elements that match the criteria
Since findIndex()
returns the first element immediately, you can’t use it to find the indices of all elements that match your criteria.
When you need to find multiple indices, you need to use the reduce()
method instead of findIndex()
.
See also: JavaScript Array.reduce()
method tutorial
The reduce()
method allows you to create a new array that fulfills certain criteria as follows:
let indices = [4, 5, 9, 12].reduce(function (accumulator, element, index ) {
if(element > 8){
return accumulator.concat(index);
}
return accumulator;
}, []);
console.log(indices); // [2, 3]
The reduce()
method above will return 2
and 3
, the indices of 9
and 12
respectively.
Now you’ve learned how the JavaScript findIndex()
method can be used to find the index of the first element that match your criteria. Good job! 👍