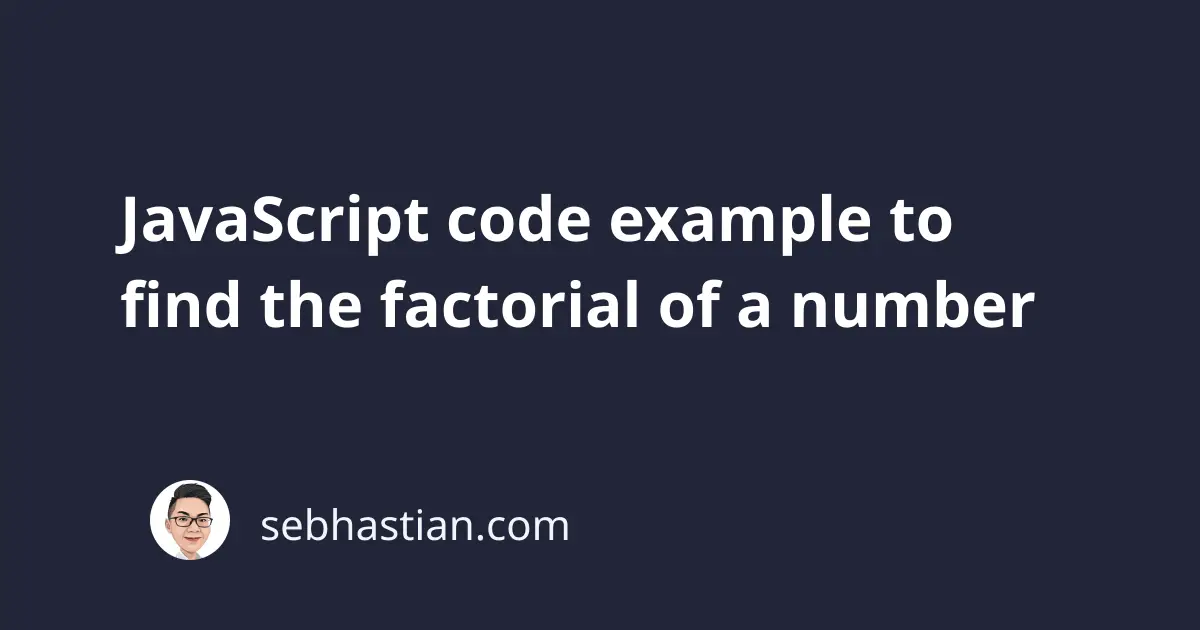
The factorial of a number is calculated by having the number n
multiplied by all positive numbers between 1
and n
. For example, to calculate the factorial number of 5
and 4
, you need to multiply the number as follows:
let factorial_5 = 5 * 4 * 3 * 2 * 1; // 120
let factorial_4 = 4 * 3 * 2 * 1; // 24
To find the factorial of a number without manually calculating it yourself, you can create a JavaScript function that calculates the factorial number for you. Let’s see how to use both iterative and recursive approaches to calculate the factorial in this tutorial.
Calculate factorial using the iterative approach
To calculate the factorial using the iterative approach, you can create a function that accepts a number
, then calculate the factorial of that number using a for
loop.
Here’s an example:
function calculateFactorial(num) {
if (num === 0 || num === 1) {
return 1;
} else {
for (let i = num - 1; i >= 1; i--) {
num *= i;
}
return num;
}
}
let factorial_7 = calculateFactorial(7);
console.log(factorial_7); // 5040
Factorials are only available for positive numbers, so it’s recommended to throw
an error when the user passes a number smaller than 0
. The following code example adds a try...catch
block to the calculateFactorial()
function:
function calculateFactorial(num) {
try {
if (num < 0) {
throw "Factorial number must be 0 or higher";
} else if (num === 0 || num === 1) {
return 1;
} else {
for (let i = num - 1; i >= 1; i--) {
num *= i;
}
return num;
}
} catch (err) {
console.log(err);
return undefined;
}
}
calculateFactorial(-7); // "Factorial number must be 0 or higher"
With the improvements above, the calculateFactorial()
function will be able to catch negative numbers and return undefined
as the factorial value for negative numbers. That will be all for calculating the factorial of a number using iterative approach. Let’s see how you can use recursive approach next.
Calculate factorial using the recursive approach
Recursive is an approach used to solve programming problems by creating a function that calls on itself until a desired outcome is achieved.
You can use a recursive approach to find the factorial of a number as follows:
function factorial(num) {
if (num === 0 || num === 1) {
return num;
}
return num * factorial(num - 1);
}
let n = factorial(8);
console.log(n); // 40320
Just like the iterative approach, you can add a try...catch
block to prevent negative numbers from getting calculated:
function factorial(num) {
try {
if (num < 0) {
throw "Factorial number must be 0 or higher";
} else if (num === 0 || num === 1) {
return 1;
} else {
return num * factorial(num - 1);
}
} catch (err) {
console.log(err);
return undefined;
}
}
let n = factorial(8);
console.log(n); // 40320
factorial(-2); // "Factorial number must be 0 or higher"
And that’s how you can calculate the factorial of a number using the recursive approach.
Conclusion
Both recursive approach and iterative approach can be used to calculate the factorial of a number, but the iterative approach using for
loop is commonly recommended because the algorithm is faster than the recursive approach. Unless you are required to use the recursive approach, it’s better to go with the iterative approach.