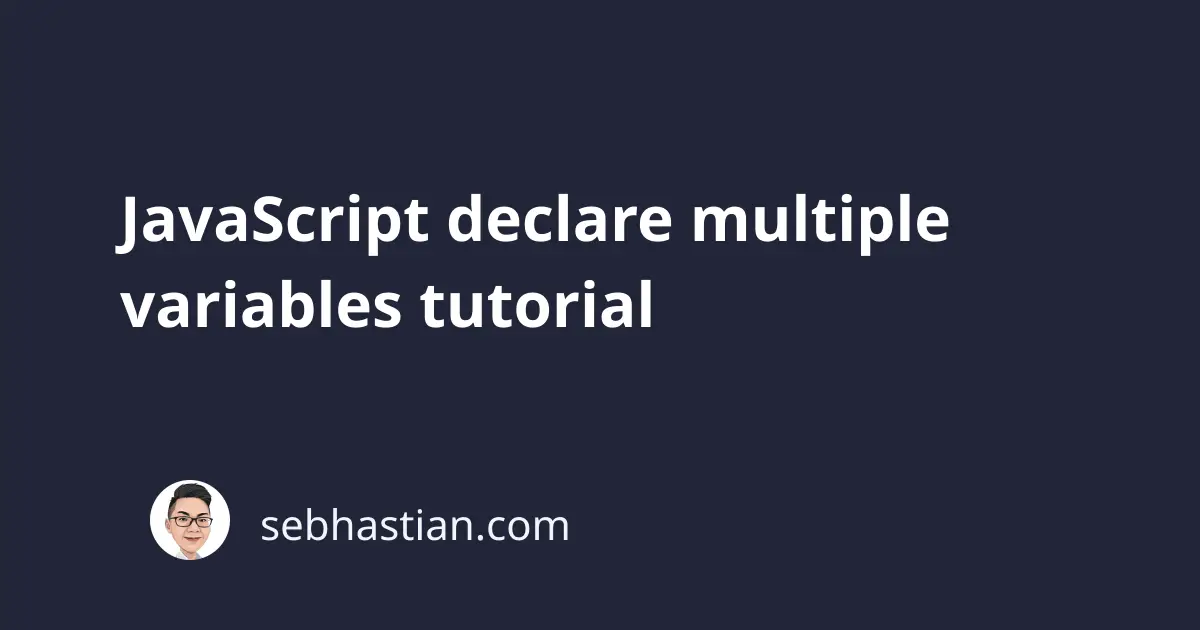
The most common way to declare and initialize JavaScript variables is by writing each variable in its own line as follows:
var price = 2;
const name = "Potato";
let currency = "SGD";
But there are actually shorter ways to declare multiple variables using JavaScript. First, you can use only one variable keyword (var, let, or const) and declare the variable names and values separated by commas.
Take a look at the following example:
let name = "Nathan", age = 28, message = "Hello there!";
The declaration above just use one line, but it’s a bit harder to read than separated declarations. Furthermore, all the variables are declared using the same keyword let
. You can’t use other variable keywords like const
and var
.
You can also make a multi-line declaration using comma-separated declaration as shown below:
let name = "Nathan",
age = 28,
message = "Hello there!";
Finally, you can also use destructuring assignment to declare and initialize multiple variables in one line as follows:
const [name, age, message] = ["Nathan", 28, "Hello there!"];
console.log(name); // "Nathan"
console.log(age); // 28
console.log(message); // "Hello there!"
The destructuring assignment from the code above extracts the array elements and assigns them to the variables declared on the left side of the =
assignment operator.
The code examples above are some tricks you can use to declare multiple variables in one line with JavaScript. Still, the most common way to declare variables is to declare them one by one, because it decouples the declaration into its own line:
const name = "Nathan";
let age = 28;
let message = "Hello there!";
The code above will be the easiest to change later as your project grows.
You can only use one variable keyword when using comma-separated declaration and destructuring assignment, so you can’t change the variables from const
to let
without changing them all.