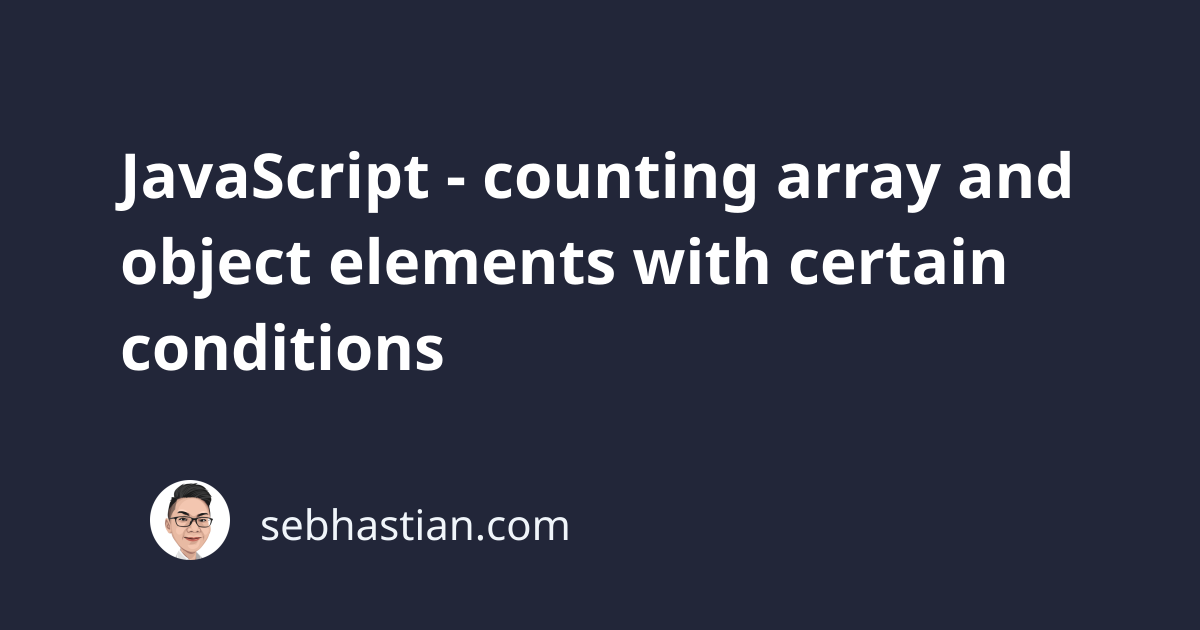
The .length
parameter of Array
type data can be used to count how many elements you have inside an array
or object
variable.
For an array, you can simply call the .length
property:
let arr = [1, 2, 3, 4, 5];
console.log(arr.length); // 5
For an object, you need to first use the Object.keys()
method, which will give you an array filled with the object variable’s keys. You can then count the length
of the keys to count how many properties owned by the object.
Here’s an example:
let obj = {
name: "John",
age: 27,
role: "Software Engineer",
};
let keys = Object.keys(obj);
console.log(keys.length); // 3
You can also chain the call between the keys()
method and the .length
property:
console.log(Object.keys(obj).length); // 3
But sometimes, you may need to count the elements conditionally. For example, you may need to count how many elements in an array are unique (no duplicates) or how many properties in object have certain values (name !== null || age > 20
)
This tutorial will help you learn how to count elements manually in an array or an object. Let’s start with counting array elements conditionally.
Counting array elements conditionally
To count array elements conditionally, you can use a combination of the Array.filter()
method and the Array.indexOf()
method.
The indexOf()
method returns the index of the element that you specify as its argument. When there are duplicate elements, the indexOf()
method returns the index of the first element it found inside the array.
Knowing how the indexOf()
method works, you can set a filter condition where the element’s indexOf()
value matches the actual index
passed by the filter()
method to the callback function. This way, you will be able to create an array of non-duplicate values.
The code below shows how this combination works:
let arr = [1, 2, 3, 4, 2, 5, 1];
let uniqueArr = arr.filter(function(element, index){
return arr.indexOf(element) === index;
})
console.log(uniqueArr); // [1, 2, 3, 4, 5]
As you can see, uniqueArr
elements are the same as the arr
array but without the duplicates. You can also change the filter condition to return only elements with duplicate values:
let arr = [1, 2, 3, 4, 2, 5, 1];
let dupeArr = arr.filter(function(element, index){
return arr.indexOf(element) !== index;
})
console.log(dupeArr); // [2, 1]
After that, you just need to count how many elements are left inside the new array by calling the .length
property.
See also: JavaScript - filtering array with multiple values
Now you know how to filter an array and count how many elements it has that meet certain conditions. Next, you will learn how to do the same with objects.
Counting object properties conditionally.
The JavaScript Object
type has the Object.keys()
method which allows you to turn an object’s keys into an array. You’ve seen how you can use it to count how many properties owned by an object before.
JavaScript also has the Object.entries()
method, which allows you to turn the object’s values into an array. You can use a combination of Object.entries()
, Array.filter()
, and Object.fromEntries()
methods to filter an object type properly.
See also: JavaScript filter object type tutorial
Here’s an example of filtering out properties with null
or undefined
values:
let user = {
name: "Nathan",
username: null,
age: 25,
role: "Software Engineer",
address: undefined
}
let objArr = Object.entries(user);
const filteredUser = objArr.filter(function ([key, value]) {
return !value; // the condition for filter. Change this as you need.
});
const newObj = Object.fromEntries(filteredUser);
console.log(newObj); // { name: "Nathan", age: 25, role: "Software Engineer" }
After that, you only need to call the Object.keys()
method chained with the length
property on the newObj
variable:
console.log(Object.keys(newObj).length); // 3
And with that, you now know how to filter object type data. You can follow the same steps but change the filter condition as you need 😉