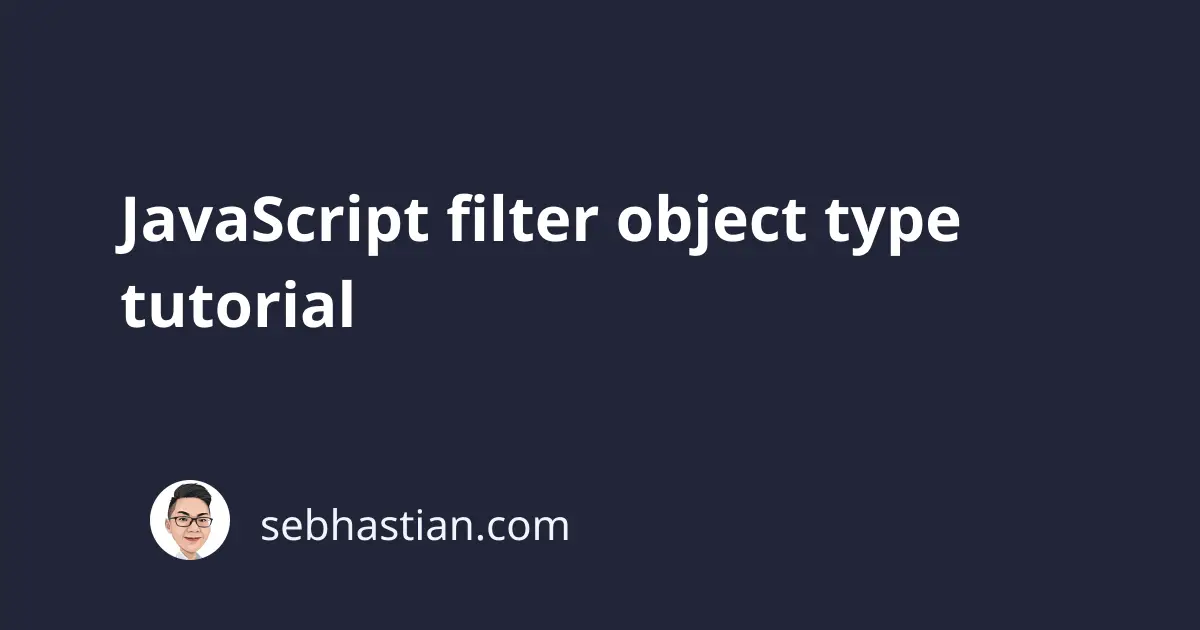
Unlike JavaScript array
type, the JavaScript object
type doesn’t have a filter()
method. When you need to filter object
type data, you need to create your own helper function.
This tutorial will show you two different ways to filter object
types:
- Filter object by its key value
- Filter object by its property value
No matter which value you want to use to filter the object
, you need to use a combination of Object.entries()
, Array.filter()
and Object.fromEntries()
methods to perform the filtering.
First, you need to transform the object
type you have into an array using Object.entries()
method. The method accepts an object
parameter and transforms it into a two-dimensional array:
const score = {
Nathan: 8,
Mia: 10,
Jack: 7,
Sunny: 6,
};
const scoreArr = Object.entries(score);
console.log(scoreArr);
The value of scoreArr
will be an array as follows:
[
["Nathan", 8],
["Mia", 10],
["Jack", 7],
["Sunny", 6],
];
Now that you have an array representing the object, you can use the Array.filter()
method to filter this array. For example, the code below will remove an element with the key "Jack"
and return the rest:
const score = {
Nathan: 8,
Mia: 10,
Jack: 7,
Sunny: 6,
};
const scoreArr = Object.entries(score);
const filteredArr = scoreArr.filter(function ([key, value]) {
return key !== "Jack";
});
The filteredArr
value will be as follows:
[
["Nathan", 8],
["Mia", 10],
["Sunny", 6],
];
Now that the array has all the elements you wanted, you need to transform the array back into an object by using Object.entries()
method. The method will transform an array of key/value pairs into an object:
const score = {
Nathan: 8,
Mia: 10,
Jack: 7,
Sunny: 6,
};
const scoreArr = Object.entries(score);
const filteredArr = scoreArr.filter(function ([key, value]) {
return key !== "Jack";
});
const newScore = Object.fromEntries(filteredArr);
console.log(newScore);
// { Nathan: 8, Mia: 10, Sunny: 6 }
And that’s how you filter an object by its key value.
Filter an object by its property value
When you want to filter an object by its property value, you need to use the same methods you just need to change the condition inside the filter()
method to filter by the property value.
For example, the code below will include only properties with a value of more than 7:
const score = {
Nathan: 8,
Mia: 10,
Jack: 7,
Sunny: 6,
};
const scoreArr = Object.entries(score);
const filteredArr = scoreArr.filter(function ([key, value]) {
return value > 7;
});
const newScore = Object.fromEntries(filteredArr);
console.log(newScore);
// { Nathan: 8, Mia: 10 }
As you can see from the code above, you can change the filter by property value by simply changing the filter()
condition. Feel free to use and modify the code example above to meet your requirement 😉