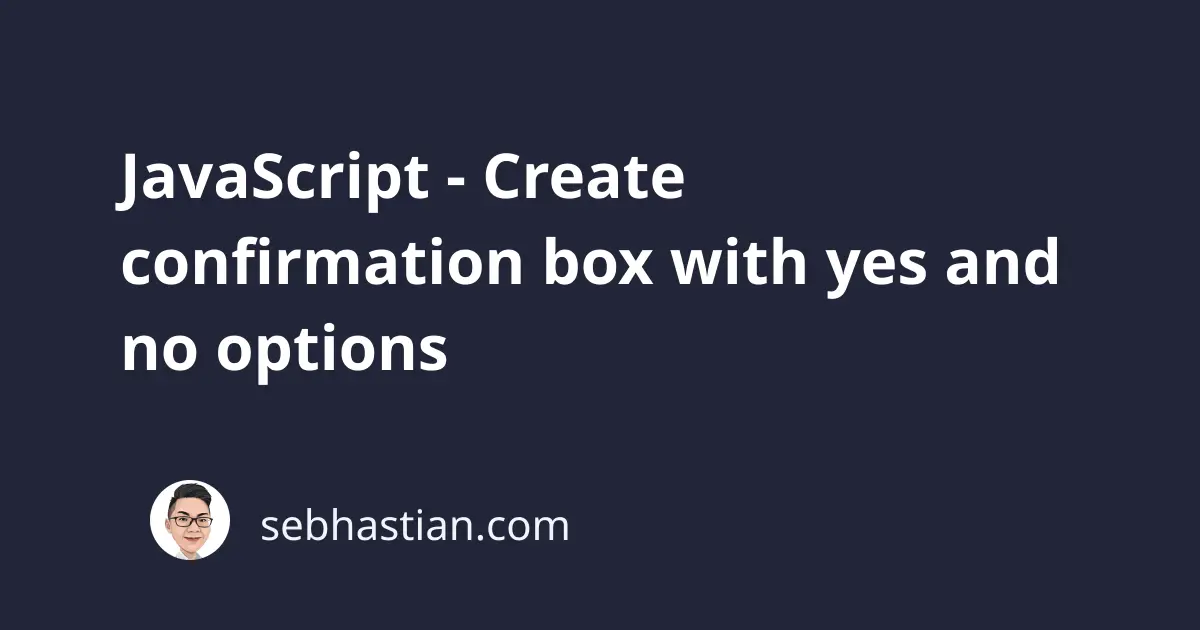
You can create a JavaScript confirmation box that offers yes and no options by using the confirm()
method.
The confirm()
method will display a dialog box with a custom message that you can specify as its argument.
It’s similar to the JavaScript alert()
method, but the difference is that confirm()
will have two buttons instead of one.
The following example code:
let isExecuted = confirm("Are you sure to execute this action?");
console.log(isExecuted); // OK = true, Cancel = false
Will produce the following dialog box:
When the user clicked the OK button, then confirm()
returns true
. Clicking on the Cancel button makes the function return false
. What you do with the return value is up to you.
Please keep in mind that the confirm()
method is part of the window
object, which means you can only call it from the browser.
You can test the confirm()
method by simply opening your browser’s console and type in confirm()
as in the screenshot below:
The following HTML page code will start the confirmation dialog when you click on the Delete button:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript confirmation box</title>
<script>
// The function below will start the confirmation dialog
function confirmAction() {
let confirmAction = confirm("Are you sure to execute this action?");
if (confirmAction) {
alert("Action successfully executed");
} else {
alert("Action canceled");
}
}
</script>
</head>
<body>
<h1>Call the confirmation box</h1>
<button onclick="confirmAction()">Delete</button>
</body>
</html>
You need to place the code you wish to run from the result of the confirmation dialog inside the if/else
code block:
if (confirmAction) {
// if true
alert("Action successfully executed");
} else {
// if false
alert("Action canceled");
}
And that’s how you can create a confirmation dialog using JavaScript.
Create confirmation dialog with Yes and No buttons
The confirmation dialog created using the confirm()
method has no parameters for changing the button labels.
You can’t replace the OK and Cancel buttons because it’s defined by the browser you are using.
To replace the button labels, you need to create your own interface using HTML elements.
You can create a simple HTML layout for the dialog box as shown below:
<body>
<div class="overlay" id="overlay" hidden>
<div class="confirm-box">
<div onclick="closeConfirmBox()" class="close">✖</div>
<h2>Confirmation</h2>
<p>Are you sure to execute this action?</p>
<button onclick="isConfirm(true)">Yes</button>
<button onclick="isConfirm(false)">No</button>
</div>
</div>
<button onclick="showConfirmBox()">Delete</button>
</body>
The <button>
element will be used to call the dialog box.
Next, add the JavaScript code to the page using the <script>
tag.
There are three functions you need to write for the page:
- The
showConfirmBox()
function - The
closeConfirmBox()
function - The
isConfirm()
function
Place the following script before the closing </body>
tag:
<script>
function showConfirmBox() {
document.getElementById("overlay").hidden = false;
}
function closeConfirmBox() {
document.getElementById("overlay").hidden = true;
}
function isConfirm(answer) {
if (answer) {
alert("Answer is yes");
} else {
alert("Answer is no");
}
closeConfirmBox();
}
</script>
Finally, you need some CSS to style the page better.
Add the following style inside your <head>
tag:
<style>
html,
body {
height: 100%;
}
.overlay {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
background: rgba(0, 0, 0, 0.8);
z-index: 2;
}
.confirm-box {
position: absolute;
width: 50%;
height: 50%;
top: 25%;
left: 25%;
text-align: center;
background: white;
}
.close {
cursor: pointer;
}
</style>
With the above style, the confirmation box will be placed in the middle of your browser window.
You can see the complete code in CodePen here:
JavaScript confirmation with yes and no buttons
And that’s how you create a confirmation box with yes and no buttons. Feel free to modify the code to meet your requirements.
Thanks for reading! 👍