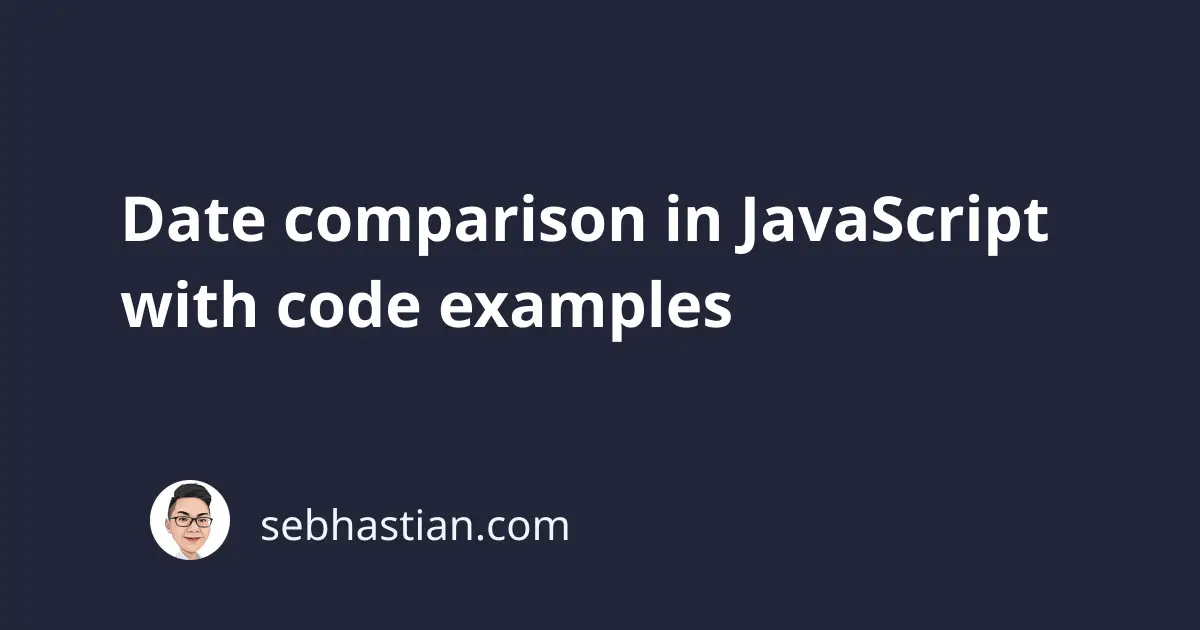
Comparing dates in JavaScript can be useful in a variety of situations, such as checking whether a certain date has passed, or determining whether a date falls within a specific year or date range.
This article will explain how to do date comparisons in JavaScript.
Comparing two dates
To compare two dates, you can use the Date
object’s built-in getTime()
method, which returns the number of milliseconds since January 1, 1970 that represents a given date.
You can then use this information to compare the two dates using comparison operators and determine which is earlier or later.
Here is an example of how to compare two dates in JavaScript:
// define two dates
let date1 = new Date("12/31/2022"); // December 31, 2022
let date2 = new Date(2022, 0, 1); // January 1, 2022
// compare the two dates
if (date1.getTime() > date2.getTime()) {
console.log("Date1 is later than Date2");
} else if (date1.getTime() < date2.getTime()) {
console.log("Date1 is earlier than Date2");
} else {
console.log("Date1 is the same as Date2");
}
In the code above, two dates are created using the new Date()
constructor: December 31, 2022 and January 1, 2022.
The getTime()
method is called to get the number
that represents both dates.
Finally, the comparison operators greater than (>
) and less than (<
) are used to compare the two dates.
The if
and else-if
conditions are set to print a message to the console indicating which date is earlier or later. The output will be:
Date1 is later than Date2
The JavaScript Date
object has a flexible construct where you can pass any kind of string that represents a date.
You can also pass number values that represent a date:
// pass string dates:
new Date("12/31/2022"); // Dec 31 2022 00:00:00
new Date("July 20, 1990"); // Jul 20 1990 00:00:00
// pass number dates:
// year, month, day
new Date(2022, 0, 1); // Jan 01 2022 00:00:00
// year, month, day, hour, minute, second
new Date(2022, 0, 1, 13, 30, 10); // Jan 01 2022 13:30:10
Most of the time, you will get a date value out of a date picker UI. That means you can pass the string or numbers representing your date into the Date()
constructor to create a Date
object.
Also, two Date
objects can be compared using the comparison operator directly like this:
// define two dates
let date1 = new Date("12/31/2022"); // December 31, 2022
let date2 = new Date(2022, 0, 1); // January 1, 2022
// compare dates without getTime()
if (date1 > date2) {
console.log("Date1 is later than Date2");
} else if (date1 < date2) {
console.log("Date1 is earlier than Date2");
} else {
console.log("Date1 is the same as Date2");
}
But the problem with comparing dates directly is that you can’t use the equality comparison operators to compare them (==
, !=
, ===
, and !==
operators).
Consider the following example:
// define two dates with the current time
let date1 = new Date();
let date2 = new Date();
// compare dates
if (date1 === date2) {
console.log("Both dates are equal");
} else {
console.log("Not equal");
}
The code above will produce the following output:
Not equal
This is because a Date
object created using the Date()
constructor will always create a unique object. In JavaScript’s eyes, the two date objects are different, even when the time value is actually the same.
As long as you’re not using an equality comparison operator, then you can compare the Date
objects directly.
Compare a date with the current date
To compare a date with the current date in JavaScript, you can use the Date object and its getTime()
method.
Here is an example of how you can compare a given date with the current date:
const givenDate = new Date("2022-12-12"); // the date you want to compare
const currentDate = new Date(); // the current date
if (givenDate.getTime() > currentDate.getTime()) {
// the given date is after the current date
} else if (givenDate.getTime() < currentDate.getTime()) {
// the given date is before the current date
} else {
// the given date is the same as the current date
}
By comparing the result of calling getTime()
on the given date and the current date, you can determine which date comes before or after the other.
Compare dates by year
To compare dates by year in JavaScript, you can use the Date
object and its getFullYear()
method.
See the example below:
const date1 = new Date("2020-12-01"); // 2020
const date2 = new Date("2022-06-01"); // 2022
if (date1.getFullYear() > date2.getFullYear()) {
console.log("Date1 year is later than Date2 year");
} else if (date1.getFullYear() < date2.getFullYear()) {
console.log("Date1 year is earlier than Date2 year");
} else {
console.log("The dates are in the same year");
}
First, you create two Date
objects, date1
and date2
, to represent two different dates.
Next, call the getFullYear()
method to retrieve the year for each date and compare them using the comparison operators.
The output of the code above will be:
Date1 year is earlier than Date2 year
Next, let’s see how you can compare dates by day value.
Compare dates by day
To compare dates by day in JavaScript, you can use the Date object and its getDate() method. Here is an example:
const date1 = new Date("2022-12-10");
const date2 = new Date("2022-12-15");
if (date1.getDate() === date2.getDate()) {
console.log("The dates are on the same day");
} else {
console.log("The dates are on different days");
}
The code above uses the getDate()
method to retrieve the day of the month for each Date
object.
The dates are then compared using the strict equality operator (===
). The code output is as follows:
The dates are on different days
Conclusion
Comparing dates in JavaScript can be useful in a variety of situations, such as creating a countdown timer or checking whether a user’s subscription has expired.
To compare two dates, you can use the Date
object’s getTime()
method.
The returned value from the getTime()
method can be compared to determine which date is earlier or later.
The Date
object is also able to compare directly as long as you’re not using the equality comparison operators.
You’ve also seen how to compare dates using the year or day value of the date. Great work!
Sometimes, you might want to compare JavaScript dates without taking into account the time part.
I’ve written another article showing how you can ignore the time part of JavaScript dates when comparing them.