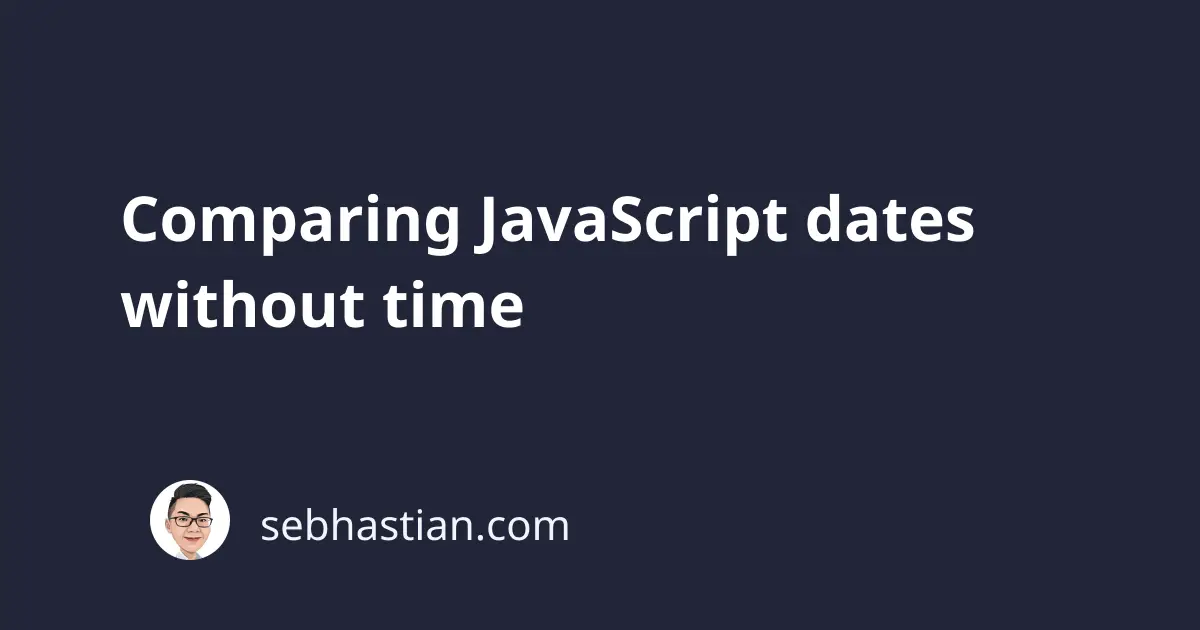
To compare dates without taking into account the time associated with each date, you need to set the time part of the date to midnight.
You can do this in JavaScript by using the setHours()
method provided by the Date
object.
The setHours()
method accept hours
, minutes
, seconds
, and milliseconds
parameters and sets them on as the time portion of the Date
object.
The code to set the time to midnight is as follows:
Date.setHours(0, 0, 0, 0);
Let’s see an example. Suppose you need to compare two dates with different time parts as follows:
const date1 = new Date('December 11, 2022 07:15:30');
const date2 = new Date('December 11, 2022 17:30:20');
if (date1.getTime() === date2.getTime()) {
console.log('Dates are equal');
} else {
console.log('Dates are NOT equal'); // ✅
}
The code above will print “Dates are NOT equal” because the time part of the dates are different.
To compare the dates without taking the time part into account, call the setHours()
method on each date as shown below:
const date1 = new Date('December 11, 2022 07:15:30');
const date2 = new Date('December 11, 2022 17:30:20');
date1.setHours(0,0,0,0);
date2.setHours(0,0,0,0);
if (date1.getTime() === date2.getTime()) {
console.log('Dates are equal'); // ✅
} else {
console.log('Dates are NOT equal');
}
Because you set the time part (hours, minutes, seconds, and milliseconds) to zero for both dates, JavaScript will print “Dates are equal”.
Keep in mind that the setHours()
method mutates the value of your Date
object.
If you need to keep the original dates, then you need to copy the original dates using the getTime()
method:
const date1 = new Date('December 11, 2022 07:15:30');
const date2 = new Date('December 11, 2022 17:30:20');
// copy the dates
const date1copy = new Date(date1.getTime());
const date2copy = new Date(date2.getTime());
date1copy.setHours(0,0,0,0);
date2copy.setHours(0,0,0,0);
if (date1copy.getTime() === date2copy.getTime()) {
console.log('Dates are equal'); // ✅
} else {
console.log('Dates are NOT equal');
}
The getTime()
method returns the number of milliseconds since January 1st, 1970 that represents the Date
object value.
Once you copy the dates, set the time part of those dates to midnight and do the comparison again.
This allows you to compare the date part while ignoring the time part (without mutating the original dates)
If you want to compare dates while taking time into account, then you can compare the Date
objects directly as long as you’re not using the equality comparison operator.
For more information, you can see my Comparing JavaScript dates article.
Now you’ve learned how to compare JavaScript dates without comparing their time part. Nice!