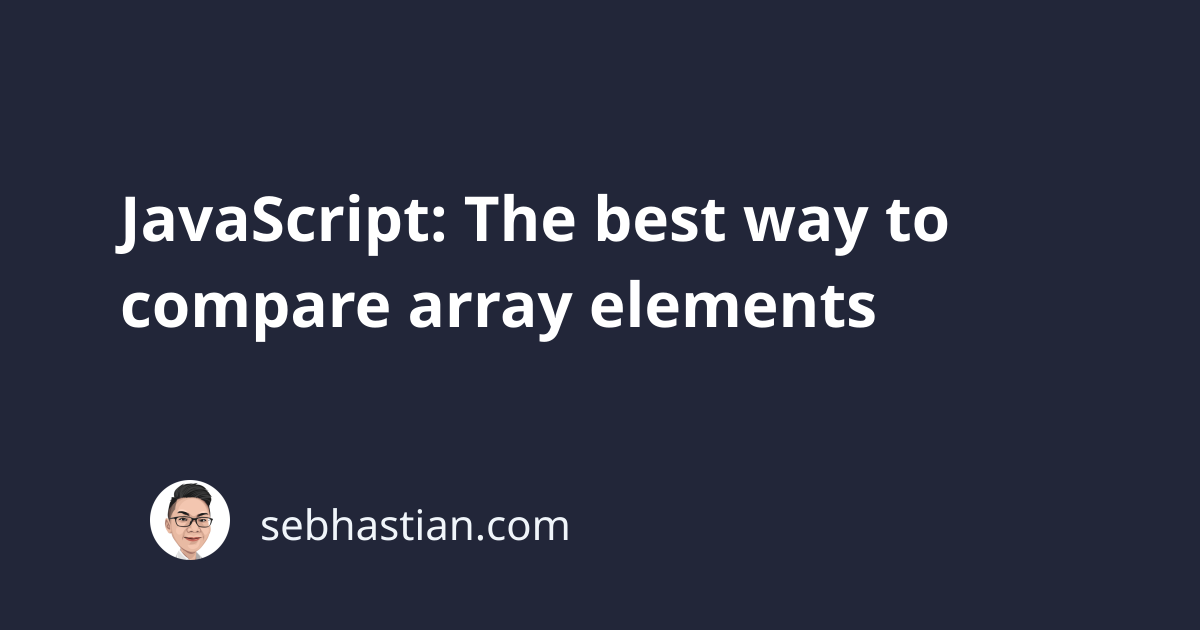
JavaScript arrays are a special type of objects, and just like regular objects, comparison of two arrays will return false
even when they contain the same elements:
let arrOne = [7, 8, 9];
let arrTwo = [7, 8, 9];
console.log(arrOne === arrTwo); // false
The equality operator will compare the reference for arrays and objects, so the only way to return true
from equality operator is to copy the array object as follows:
let arrOne = [7, 8, 9];
let arrTwo = arrOne;
console.log(arrOne === arrTwo); // true
But this won’t help much when you need to compare two arrays from different references, so let’s see how you can compare the elements of an array
Compare arrays using JSON
The most common solution is to compare the arrays using JSON.stringify()
method so you have two serialized strings.
Here’s an example:
let arrOne = [7, 8, 9];
let arrTwo = [7, 8, 9];
console.log(JSON.stringify(arrOne) === JSON.stringify(arrTwo)); // true
But this method compares the arrays indirectly, and having the same values in different orders will return false
instead of true
. If you want to actually compare the elements of two arrays programmatically, you need to use other methods.
Compare arrays with every() method
You can use the combination of comparing the arrays length
values and their elements using every()
method as follows:
let arrOne = [7, 8, 9];
let arrTwo = [7, 8, 9];
let result =
arrOne.length === arrTwo.length &&
arrOne.every(function (element, index) {
// compare if the element matches in the same index
return element === arrTwo[index];
});
console.log(result); // true
This way, you compare if the element at a specific index is really equal or not. Still, this method requires both arrays to have the same element at the index in order to return true
. If you don’t care about the order and only want both arrays to have the same elements, you can use the following method.
Compare out of order array elements
In order to compare array elements that are out of order, you can use the combination of every()
and includes()
method.
The includes()
method determines whether an array includes a certain element among its entries, regardless of the index:
let arrOne = [7, 8, 9];
let arrTwo = [9, 7, 8];
let result =
arrOne.length === arrTwo.length &&
arrOne.every(function (element) {
return arrTwo.includes(element);
});
console.log(result); // true
The includes()
method are added into JavaScript on ES7, so if you need to support older JavaScript versions, you can use indexOf()
method as an alternative.
Since indexOf()
returns -1
when the specified element is not found, you can make every()
return true
when indexOf(element) !== -1
.
Here’s how it works:
let arrOne = [7, 8, 9];
let arrTwo = [9, 7, 8];
let result =
arrOne.length === arrTwo.length &&
arrOne.every(function (element) {
return arrTwo.indexOf(element) !== -1;
});
console.log(result); // true
Comparing arrays in JavaScript is not straightforward as comparing strings, but if what you need is to compare whether the elements are included, the above methods should suffice for your project.