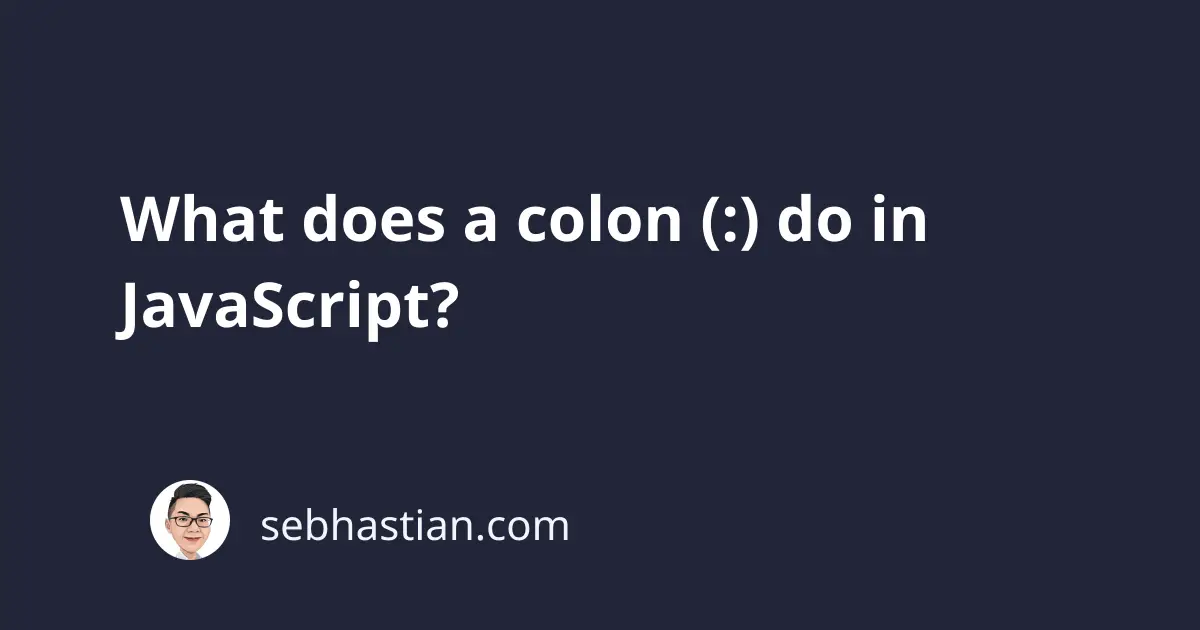
There are three ways a colon can be used in JavaScript, and this tutorial will help you to understand them all. First, a colon can be used as a delimiter to the key/value pair of an object properties.
Colon as an object property key/value pair delimiter
The colon symbol (:
) is generally used by JavaScript as a delimiter between key/value pair in an object
data type.
For example, you may initialize an object named car
with key values like brand
and color
as follows:
let car = {
brand: "Toyota",
color: "red",
};
In the above example, the colon symbol is used to delimit the properties of the car
object. The property brand
has the value "Toyota"
while the property color
has the value "red"
, and both used a colon to separate between the key and the value.
The following assignment expressions also do the same thing as the above:
let car = {};
car.brand = "Toyota";
car.color = "red";
Without using the colon as a delimiter, you need to initialize the car
object as an empty object first, then assign the properties one by one.
Colon as a delimiter of the ternary operator expressions
The ternary operator is a short-hand operator that allows you to evaluate a condition. It’s commonly used as a shortcut to the if
statement.
The colon symbol is used to delimit the two expressions inside a ternary operator.
Take a look at the following example:
let name = "John";
console.log(name ? `Hello, ${name}` : "No one to greet!");
In the code above, the console will output Hello, ${name}
when the name
variable value evaluates to true
or "No one to greet!"
when the variable evaluates to false
.
The colon symbol is used to separate the expressions that the operator will execute.
Colon as a delimiter to switch statement cases
Finally, the colon symbol can also be used to delimit a case inside a switch
statement.
The symbol will separate between the case
expression and its code block as in the code below:
let age = 15;
switch (age) {
case 10:
console.log("Age is 10");
break;
case 20:
console.log("Age is 20");
break;
default:
console.log("Age is neither 10 or 20");
}
The code after the colon symbol will be executed when the case
expression evaluates to true
.
And those are the three ways that a colon symbol is used in JavaScript 😉