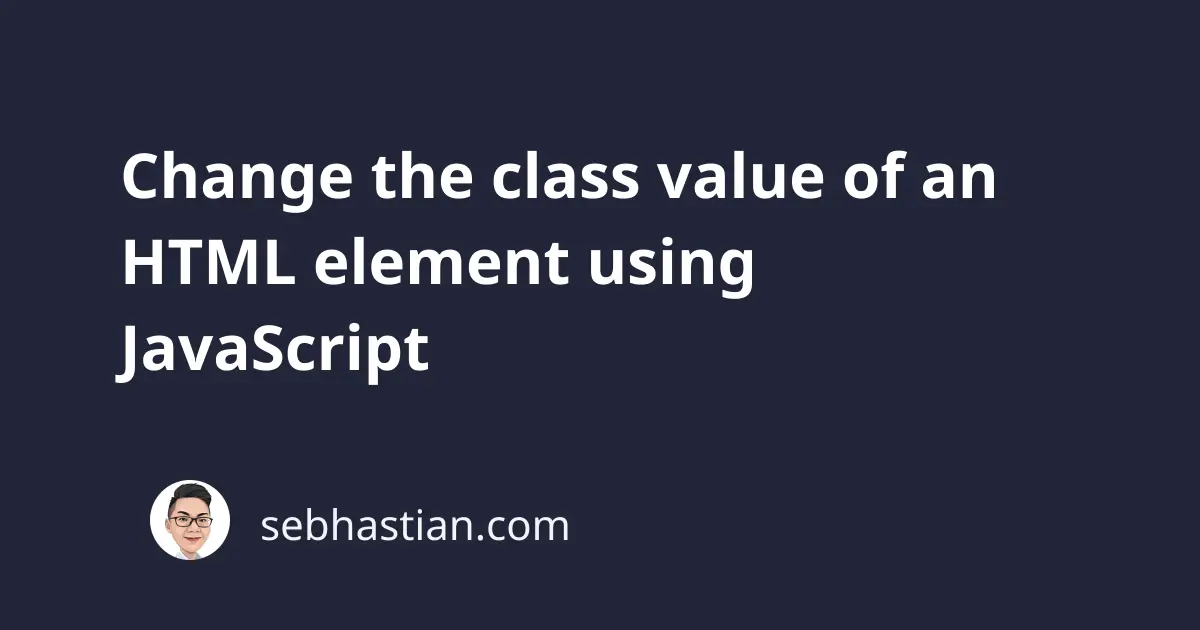
To change an HTML element’s class
attribute value using JavaScript, you can use either the className
or the classList
property provided for every single HTML element.
This tutorial will help you learn how to use both methods, starting from the className
property.
Change the class value using className property
The className
property allows you to fetch an HTML element’s class
attribute value as a string.
Here’s an example of how className
property works:
<body>
<p id="header" class="red">Hello World! This is a paragraph element.</p>
<script>
const paragraph = document.getElementById("header");
console.log(paragraph.className); // "red"
</script>
</body>
Now that you know how the className
property works, you can change the class
value of an element by assigning a new value to the property as follows:
<body>
<p id="header" class="red">Hello World! This is a paragraph element.</p>
<script>
const paragraph = document.getElementById("header");
paragraph.className = "yellow blue";
console.log(paragraph.className); // "yellow blue"
</script>
</body>
Using the className
property allows you to change the class
attribute value, but you need to add the previous value manually if you want to keep it.
For example, to keep the class "red"
from the <p>
element above and adds a new class named "font-large"
, you need to assign both classes as follows:
const paragraph = document.getElementById("header");
paragraph.className = "red font-large";
Even though the "red"
class is already present, you still need to assign it again, creating a small redundancy in your code.
To avoid this kind of redundancy, you can use the classList
property instead.
Change the class value using classList property
The classList
property is a read-only property of an HTML element that returns the class
attribute value as an array. The property also provides several methods for you to manipulate the class
attribute value.
For example, to add new class names without removing the current value, you can use the classList.add()
method.
Using the same <p>
element example above, here’s how to add the "font-large"
class without removing the "red"
element:
<body>
<p id="header" class="red">Hello World! This is a paragraph element.</p>
<script>
const paragraph = document.getElementById("header");
paragraph.classList.add("font-large");
console.log(paragraph.classList); // ["red", "font-large"]
</script>
</body>
When you want to remove an element’s class
attribute value, you can use the remove()
method, which allows you to remove()
class names as a comma-delimited string:
<body>
<p id="header" class="red">Hello World! This is a paragraph element.</p>
<script>
const paragraph = document.getElementById("header");
paragraph.classList.add("font-large");
paragraph.classList.remove("red");
console.log(paragraph.classList); // ["font-large"]
</script>
</body>
For more information on classList
property you can read the following post:
JavaScript classList() property and methods explained
And that’s how you can change the class
attribute value using the className
or classList
property methods.