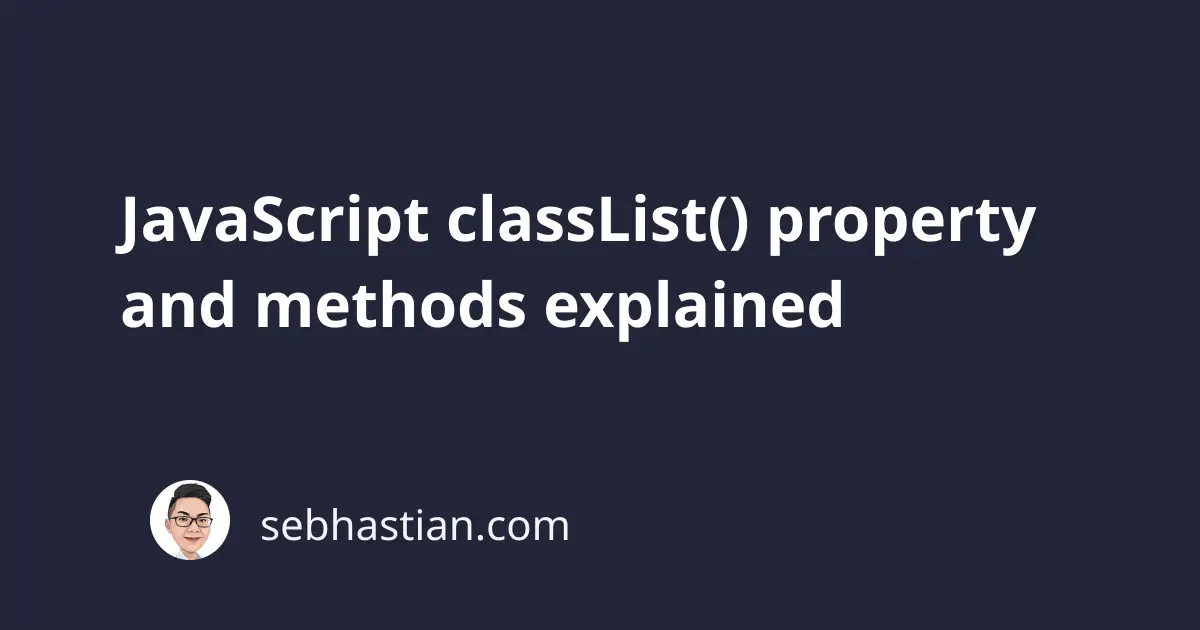
The classList
property is a property of an HTML element that holds the class names of the element currently selected. You can use the property to fetch the class names of an HTML element as an array.
For example, suppose you have the following HTML element in your <body>
tag:
<body>
<div id="header" class="navbar open">Header div</div>
</body>
You can grab the <div>
element above using the document.getElementById()
method, then you can fetch the class names of the element using either className
or classList
property.
The className
property returns the class names as a string, while classList
property returns the class names as an array:
const div = document.getElementById("header");
console.log(div.className); // "navbar open"
console.log(div.classList); // ["navbar", "open"]
console.log(div.classList[0]); // "navbar"
console.log(div.classList[1]); // "open"
The classList
property is a read-only property. If you want to manipulate the HTML element’s classes, you need to use the methods included in the classList
property. They are as follows:
add()
contains()
item()
remove()
toggle()
Let’s learn how these methods work, starting from the add()
method.
classList add() method explained
The add()
method allows you to add one or more class names to an element. You only need to pass the class names you want to add as a comma-delimited string:
Here’s an example of adding a new class name to the <div>
element above:
const div = document.getElementById("header");
div.classList.add("expand");
If the class name is already present on the element, then it won’t be added again to the element. The code below does nothing because the class name "navbar"
is already present on the <div>
element above:
div.classList.add("navbar");
Next, let’s learn how contains()
method works.
classList contains() method explained
The contains()
method will check on your element’s class
attribute and check if it contains a specific class name.
You need to pass the class name you want to check as its argument. The method will return true
if the class name is present or false
when it is not present.
For example, here’s how to check if an element has the "navbar"
class present:
const div = document.getElementById("header");
div.classList.contains("navbar"); // true
div.classList.contains("lg-block"); // false
And that’s all about the contains()
method. Next, we have the item()
method.
classList item() method explained
The item()
method of the classList property returns the class name at the index you specified as its argument. It works the same way as using the classList[index]
check except for when the specified index is non-existent:
const div = document.getElementById("header");
console.log(div.classList.item(0)); // "navbar"
console.log(div.classList.item(1)); // "open"
console.log(div.classList.item(2)); // null
console.log(div.classList[0]); // "navbar"
console.log(div.classList[1]); // "open"
console.log(div.classList[2]); // undefined
As you can see from the code above, the item()
method returns null
but the classList[index]
check returns undefined
when the index is non-existent.
Next, let’s learn the remove()
method.
classList remove() method explained
The classList.remove()
method allows you to remove one or more class names from an HTML element. Like the add()
method, you need to pass the class names you want to remove as a comma-delimited string. You can pass as many string parameters as you need:
const div = document.getElementById("header");
// remove three class names
div.classList.remove("navbar", "expand", "lg-block");
When the class name you want to remove is not present in the element’s class
attribute, JavaScript will continue and try to remove the next class name you passed into the method.
In other words, removing a class that doesn’t exist won’t throw an error.
Finally, let’s see how the toggle()
method works.
classList toggle() method explained
The classList.toggle()
method allows you to add or remove a class name based on the current class
attribute value.
If the class name you want to toggle already present inside the element, the toggle()
method will remove that class name, and vice versa.
For example, suppose you have a <p>
element as follows:
<p id="header" class="red">Hello World! This is a paragraph element.</p>
You can call the toggle()
method once to remove the class "red"
from the element as in the code below:
const paragraph = document.getElementById("header");
paragraph.classList.toggle("red"); // false
paragraph.classList.toggle("red"); // true
You can know if the toggle()
method adds or removes a class by the value it returns. If the class name is removed, toggle()
will return false
. When the class name is added, it will return true
.
Conclusion
You’ve just learned about the classList
property and how you can use the provided methods to manipulate the class names of an HTML element. Now go ahead and try to manipulate your own HTML element’s class 😉