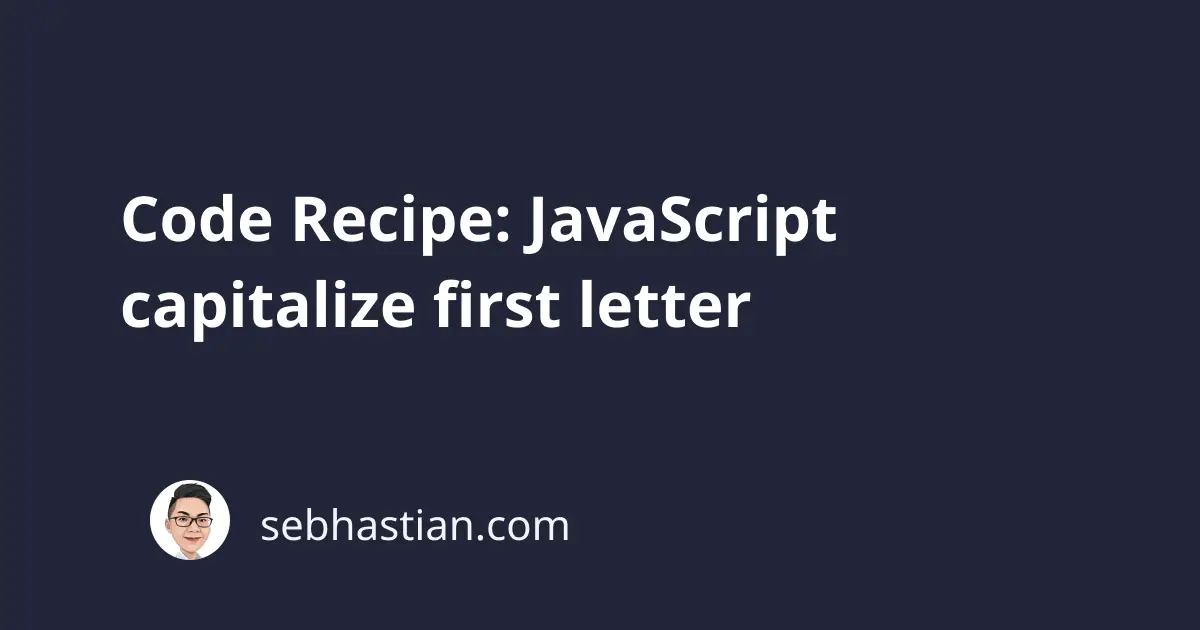
JavaScript doesn’t have any built-in methods to capitalize the first letter of a word or a sentence of words. You will need to create your own function to do so.
Luckily, JavaScript still provides built-in methods for string
data manipulation that will make the job easier for you.
Capitalizing the first letter of a word
First, you need to access the first letter of your word using the charAt()
method, which returns the character at the specified index. Here’s an example:
let word = "fire";
console.log(word.charAt(0)); // f
console.log(word.charAt(1)); // i
console.log(word.charAt(2)); // r
console.log(word.charAt(3)); // e
Next, you can chain the toUpperString()
method to transform the letter returned by the charAt()
method:
let word = "fire";
console.log(word.charAt(0).toUpperCase()); // F
Finally, you can use the slice()
method to retrieve the second letter of the word until the last one. Let’s put it all together:
let word = "fire";
let cpWord = word.charAt(0).toUpperCase() + word.slice(1);
console.log(cpWord); // Fire
Finally, to make this code complete, you can use toLowerCase()
method to fix a string with random cases like this one:
let word = "vAlEnTInE";
let lcWord = word.toLowerCase(); // valentine
let cpWord = lcWord.charAt(0).toUpperCase() + lcWord.slice(1);
Reusable capitalize first function
Now you can conveniently put the code snippet as a function that you can call whenever you need to capitalize the first letter of a word. Let’s call this function cpFirst()
which stands for “Capitalize First”:
function cpFirst(word) {
let lcWord = word.toLowerCase();
return lcWord.charAt(0).toUpperCase() + lcWord.slice(1);
}
You can test and see if the function works properly:
console.log(cpFirst("ProgramMinG")); // Programming
console.log(cpFirst("welL helLo TheRE")); // Well hello there
You now have a reusable JavaScript function for capitalizing the first letter of a string. Next, you will learn how to create a function that can capitalize each word in a sentence.
Capitalizing a sentence of words
If you want to capitalize each word of a sentence, you need to split the sentence string
into an array
of words using the split()
method. For example:
let sentence = "welcome to the world of programming";
let words = sentence.split(" ");
console.log(words);
Here’s the value of the words
variable above:
Array ["welcome", "to", "the", "world", "of", "programming"]
Now you can use the array map()
method to loop over the entire words and capitalize the first letter of each word:
let sentence = "welcome to the world of programming";
let words = sentence.split(" ");
let cpWords = words.map((word) => {
let lcWord = word.toLowerCase();
return lcWord.charAt(0).toUpperCase() + lcWord.slice(1);
});
console.log(cpWords);
The value of cpWords
will be as follows:
Array ["Welcome", "To", "The", "World", "Of", "Programming"]
Finally, you need to put the words back together as a sentence using the join()
method:
let cpSentence = cpWords.join(" ");
Reusable capitalize all function
Now you can put it all together as a reusable function. Let’s name this function cpAll()
which stands for “Capitalize All”:
function cpAll(input) {
let words = input.split(" ");
let cpWords = words.map((word) => {
let lcWord = word.toLowerCase();
return lcWord.charAt(0).toUpperCase() + lcWord.slice(1);
});
return cpWords.join(" ");
}
You can now test the function. It will work both for a single word and a sentence:
console.log(cpAll("ProgramMinG")); // Programming
console.log(cpAll("welL helLo TheRE")); // Well Hello There
Conclusion
You have just learned how to create reusable JavaScript functions to capitalize the first letter of words. Great job!
Depending on your need, you can choose between a function that capitalizes only the first word or capitalize all the words. Feel free to use cpFirst()
and cpAll()
functions in your code. They are free of charge 😉