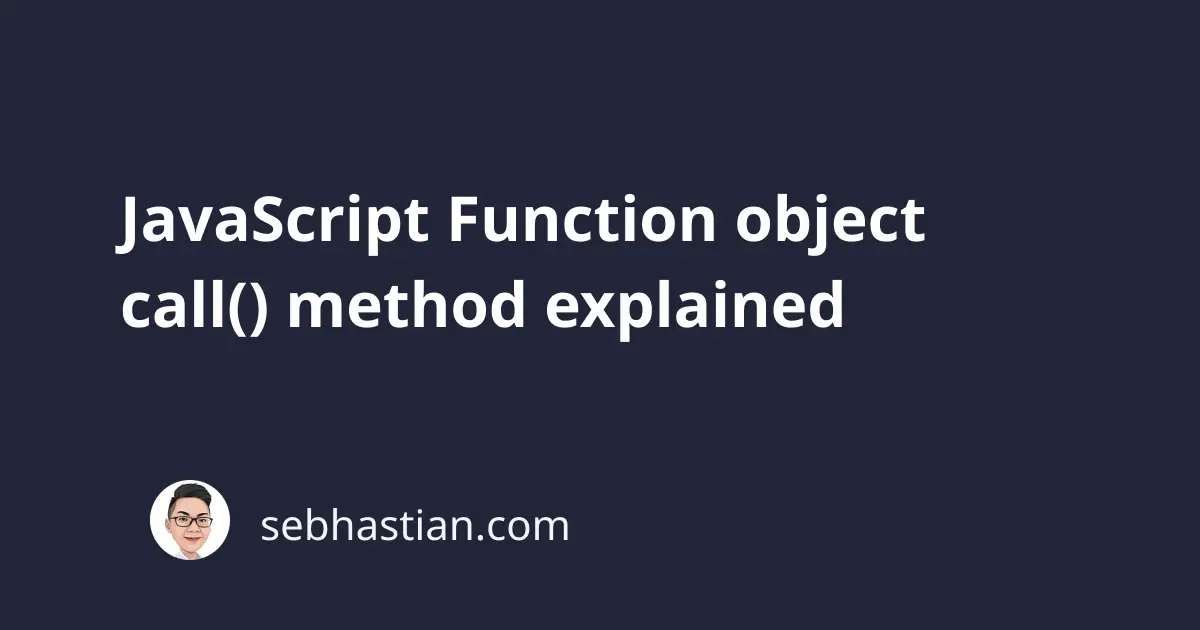
JavaScript Function
object prototype has a method named call()
that allows you to change the context of this
keyword inside the function block.
In JavaScript, this
refers to the context object from which you call the this
keyword.
In the following example, the hello()
function will log the name
property of the this
keyword, which refers to the function itself:
function hello() {
console.log(this.name);
}
hello(); // undefined
But since the hello()
function doesn’t have a name
property, this.name
will return undefined
as its value.
The call()
method is provided by JavaScript in the Function
object prototype, which means you can call it from JavaScript functions.
The code below shows how you can use the call
method to make the this
keyword refers to the person
object:
const person = {
name: "Nathan",
};
function hello() {
console.log(this.name);
}
hello.call(person); // Nathan
Because you pass the person
object to the call()
method of the hello
function, the this
keyword will refer to the person
object inside the hello()
function block.
The syntax of the call method is as follows:
call()
call(thisArg)
call(thisArg, arg1)
call(thisArg, arg1, ... , argN)
When you call the call()
method without passing any argument, then the function will be executed as if you’re calling it normally:
hello.call(); // the same as hello()
The first argument of the call()
method will always be the this
object.
Any arguments defined after the first argument will be passed to the function as its parameters:
const person = {
name: "Nathan",
};
function hello(age, gender) {
console.log(this.name);
console.log(age);
console.log(gender);
}
hello.call(person, 27, "Male");
// Output:
// Nathan
// 27
// Male
Keep in mind that the call()
method can’t change the this
keyword context for arrow functions.
If you create your functions using the arrow function syntax, the this
keyword always refers to the global object defined in the environment where you run your JavaScript code.
Consider the following example:
const person = {
name: "Nathan",
};
const hello = (age, gender) => {
console.log(this.name);
};
this.name = "John";
hello.call(person); // John
Even though you passed the person
object as an argument to the call()
method above, the context of the this
keyword doesn’t change.
The global object is the window
object when you run the code from the browser.
In Node.js, it will be the global
object.
Without the this.name = "John"
defined, the above code will return undefined
.
And that’s how the call()
method works in JavaScript functions. This method allows you to change the context of this
keyword when running a function:
const person = {
name: "Nathan",
};
const dog = {
name: "Logan",
};
function hello() {
console.log(this.name);
}
hello.call(person); // Nathan
hello.call(dog); // Logan
As of today, the call()
method is available and supported by all modern browsers as you can see here.
Good work on learning about the Function.prototype.call()
method. 😉