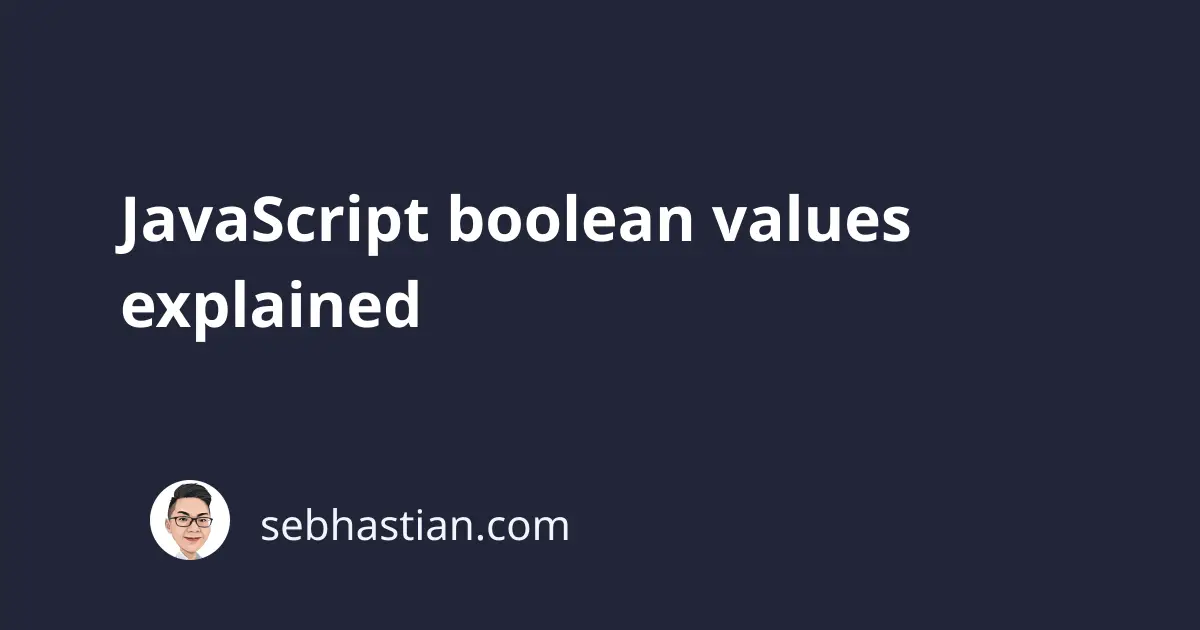
JavaScript boolean
values are used to represent truth logical values.
There are only two boolean type values: true
or false
.
The boolean values are commonly returned when you perform a comparison operator as follows:
50 > 20; // return true
10 === 15; // return false
You can assign a boolean value to variables like this:
let isAlive = true;
The boolean values are used when you need to perform a logical evaluation.
You can also use it to evaluate conditions required by an if
statement.
For example, the age
variable value must be greater than or equal to 18
to run the if
statement block below:
let age = 15;
if (age >= 18) {
console.log("You may enter");
} else {
console.log("You shall not enter");
}
Values of another type in JavaScript always have a boolean
type evaluation intrinsically.
For example, 0
equals false
while other numbers are equal to true
.
You can check the boolean representation of values by passing that value to the Boolean()
function as shown below:
Boolean(0); // false
Boolean(1); // true
Boolean(-10); // true
Boolean(""); // false
Boolean("H"); // true
Boolean(null); // false
Boolean(undefined); // false
An empty string also returns false
, while any other string returns true
.
You can see the list of values that evaluate to true
and false
here:
JavaScript truthy falsy values explained
Finally, JavaScript also provides the Boolean
constructor that you can use to create a Boolean
object.
But keep in mind that the primitive value boolean
is different than the object value Boolean
.
Here’s an example of the two:
const primitiveBool = true;
const objBool = new Boolean(true);
console.log(typeof primitiveBool); // boolean
console.log(typeof objBool); // object
As you can see above, the type of primitiveBool
evaluates to primitive boolean
. On the other hand, the objBool
value evaluates to an object
.
You need to keep this in mind because an object
will evaluate to true
even when the object is a Boolean
with a false
value.
Consider the following example:
const objBool = new Boolean(false);
if (objBool){
console.log(`Object value: ${objBool}`)
// Object value: false
}
When you use a Boolean
object for an if
statement condition, the condition will always evaluate to true
.
On the other hand, if you compare an object to a primitive boolean value, it will always return false
:
const objBool = new Boolean(false);
console.log(objBool === true); // false
console.log(objBool === false); // false
When developing JavaScript applications, you are recommended to always use the primitive boolean
type and avoid the Boolean
object.
The Boolean()
function can also be used when you need to find the primitive boolean value of an expression or value.
And that’s how the boolean values work in JavaScript. 👍