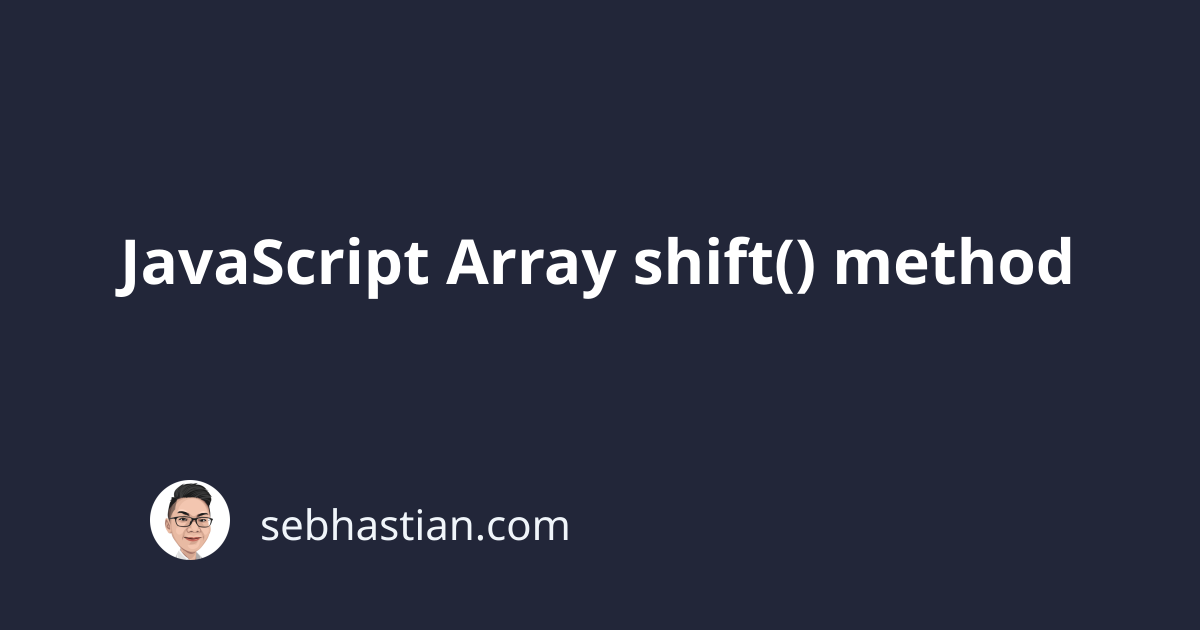
The JavaScript Array shift()
method allows you to remove the first element (at index zero) of your array, while also returning that element to you. The shift()
method does not have any parameters.
Here’s an example of shift()
in action:
let programmingLangs = ["JavaScript", "Ruby", "Python"];
let shiftedLang = programmingLangs.shift();
console.log(programmingLangs); // ["Ruby", "Python"]
console.log(shiftedLang); // "JavaScript"
Because the first element is a string, the value returned by shift()
is also a string. If your array contains another array or an object, that will be returned as well:
let fruitCart = [
{
name: "Apple",
quantity: 3,
},
{
name: "Orange",
quantity: 1,
},
{
name: "Banana",
quantity: 3,
},
];
let shiftedFruit = fruitCart.shift();
console.log(shiftedFruit); // { name: "Apple", quantity: 3 }
You can use the shift()
method for any array type data you have.