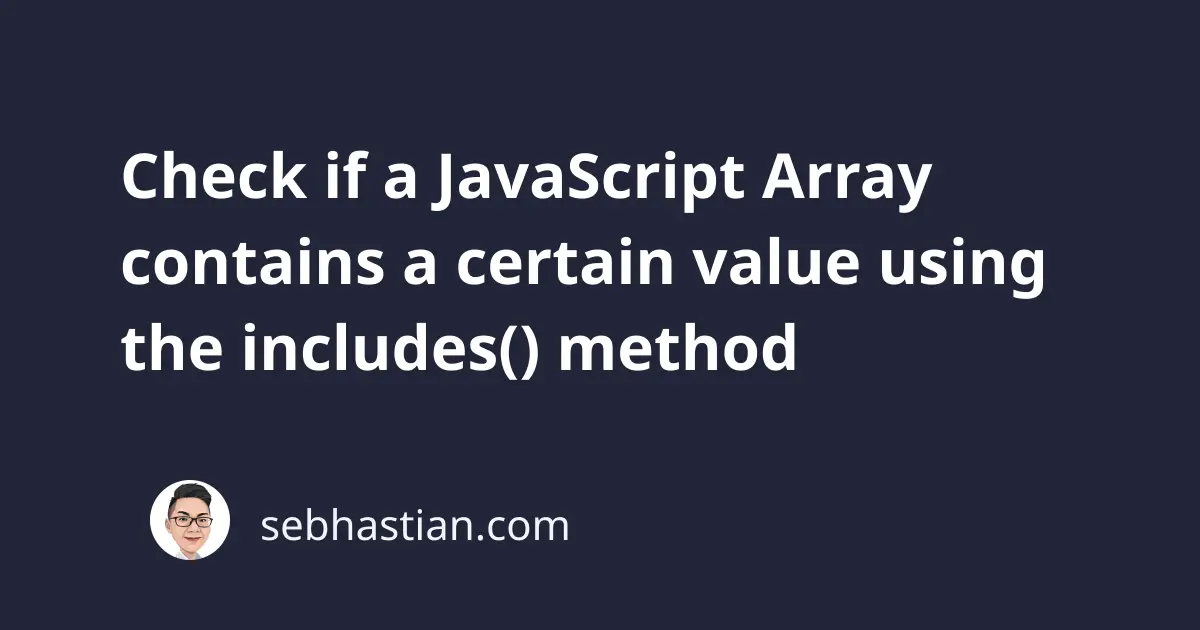
To check if a JavaScript array contains a certain value or element, you can use the includes()
method of the Array
object.
The includes()
method returns the Boolean true
if your array contains the value you specified as its argument. Otherwise, the method returns false
.
The code below shows how you can use the includes()
method to check if the names
array contains the string value "John"
const names = ["Nathan", "Jane", "John"];
console.log(names.includes("John")); // true
The includes()
method has two parameters:
- The first parameter is the value you want to search for in the array
- The second parameter is the start index to perform the search
The second parameter of the method is optional:
Array.includes(value);
Array.includes(value, startIndex);
Without the startIndex
second parameter, the includes()
method will start from index zero.
When you define the second parameter, the method will skip the previous index even if it contains the value you look for:
const names = ["Nathan", "Jane", "John"];
console.log(names.includes("Nathan", 1)); // false
The includes()
method above returns false
because the value "Nathan"
is at index 0
, but the search starts from index 1
.
Keep in mind that the includes()
method is case sensitive, so please make sure you use the right case when calling the method:
const letters = ["a", "b", "c"];
console.log(letters.includes("A")); // false
console.log(letters.includes("a")); // true
Finally, the includes()
method only informs you whether the array contains a specific value.
If you want to find the index of the value as well, you need to use the indexOf()
method:
const letters = ["a", "b", "c"];
console.log(letters.indexOf("A")); // -1
console.log(letters.indexOf("a")); // 0
When the value is not found, the indexOf()
method returns -1
.
This means when the method returns any other value than -1
, the array contains the specified value:
const letters = ["a", "b", "c"];
if (letters.indexOf("A")) {
console.log("Value is found");
} else {
console.log("Value is not found");
}
// or
if (letters.includes("A")) {
console.log("Value is found");
} else {
console.log("Value is not found");
}
Depending on what you want to do next, you can use either the includes()
or indexOf()
method to check if a JavaScript array contains a certain value.