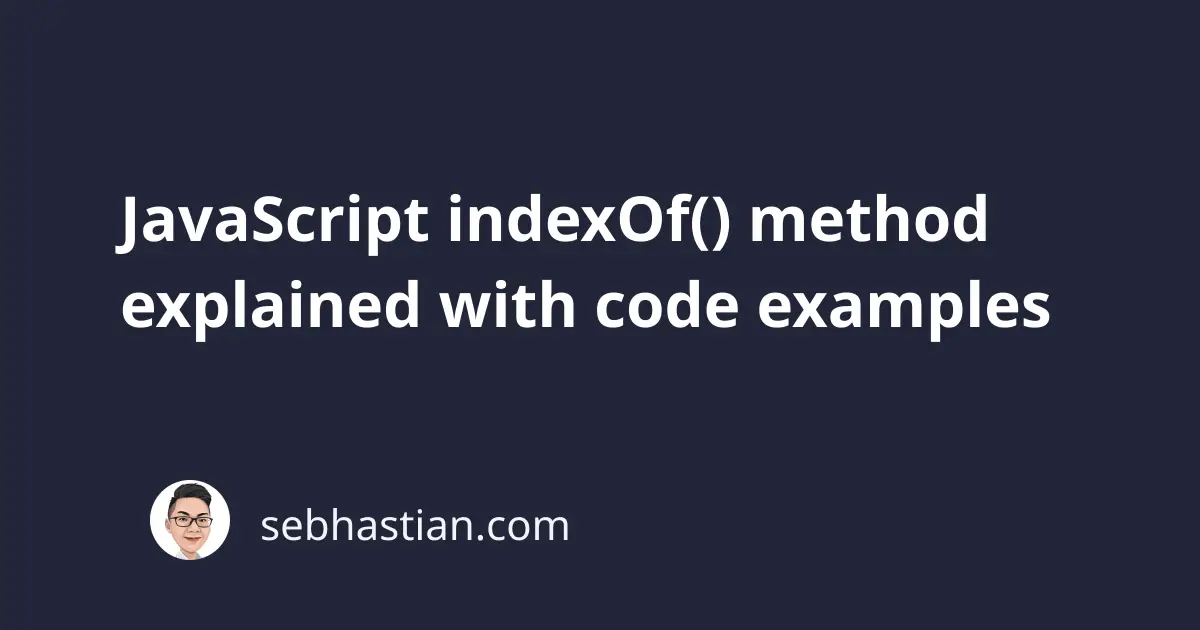
The JavaScript indexOf()
method is a method defined for String
and Array
prototype objects.
The method is used to return the index position of the value you passed as its argument.
Here’s an example of the indexOf()
method in action:
// indexOf for a string
console.log("Language".indexOf("a")); // 1
// indexOf for an array
console.log([1, 2, 3].indexOf(2)); // 1
Both the letter a
and the number 2
above are located on the index position of 1
, so the indexOf()
method returns 1
.
The full syntax of the method is as follows:
indexOf(searchValue)
indexOf(searchValue, startIndex)
The method will return -1
when the searchValue
is not found in the string or array:
console.log("Language".indexOf("z")); // -1
console.log([1, 2, 3].indexOf(5)); // -1
The startIndex
second parameter of the method is used to let the method search from a specific index.
The following example shows how to start the indexOf()
search at index 3
:
console.log("Language".indexOf("a", 3)); // 5
Even though the first occurrence of the letter a
in the string Language
is at index 2
, the second parameter causes the search to start from index 3
. The indexOf()
method returns 5
instead of 1
.
When you have multiple occurrences, then the indexOf()
method returns only the first occurrence found.
The method always searches for the specified value from left to right.
And that’s how the indexOf()
method works in JavaScript. 😉