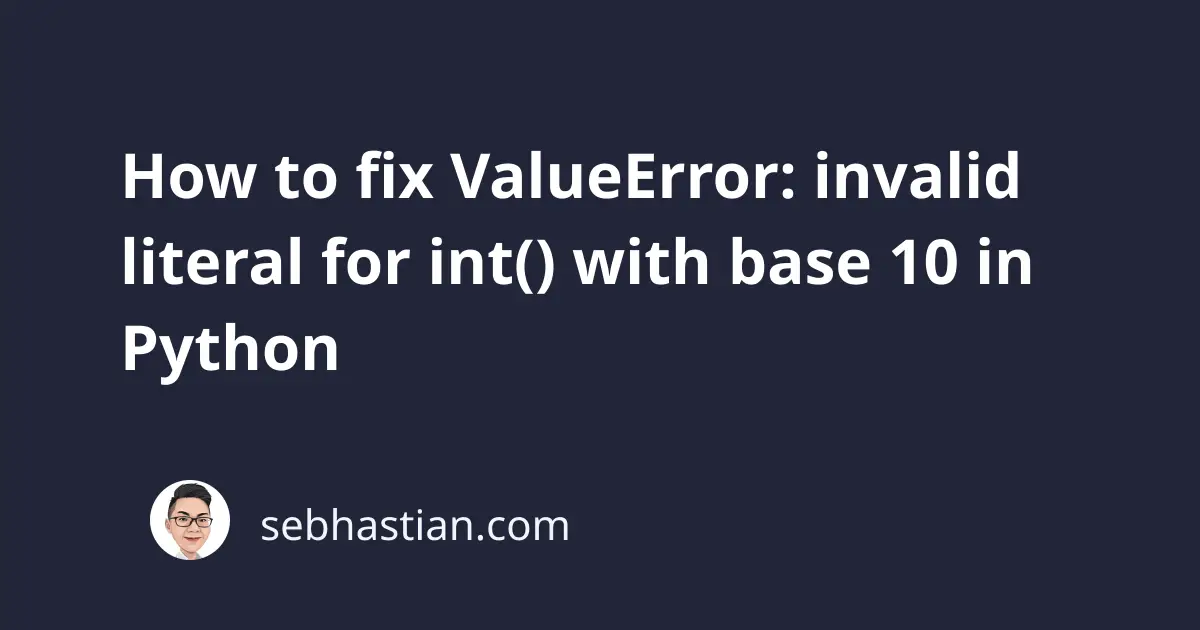
When running Python code, you might encounter the following error:
ValueError: invalid literal for int() with base 10
This error occurs when you attempt to convert a string that represents a floating number into an integer using the int()
function.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you run an input()
function to ask the user for a decimal number as follows:
answer = input("Enter a decimal number: ")
Next, you want to convert the decimal number into an integer, so you called the int()
function to the answer
as follows:
num_result = int(answer)
print(num_result)
When running the code, you’ll get an error as follows:
Enter a decimal number: 4.5
Traceback (most recent call last):
File "main.py", line 3, in <module>
num_result = int(answer)
ValueError: invalid literal for int() with base 10: '4.5'
The error occurs when you passed a string that can’t be converted into an integer directly.
In the example, we passed a string ‘4.5’ to the int()
function because the input()
function always returns a string. Python can’t convert the answer
into an integer this way.
How to fix this error
To resolve this error, you need to convert the string of decimal numbers into a float by calling the float()
function.
You can call both float()
and int()
functions in one line as follows:
answer = input("Enter a decimal number: ")
num_result = int(float(answer))
print(num_result)
This way, Python can convert the string float into an integer without triggering the error.
Keep in mind that if you have a non-numerical value in the string, you might get the could not convert string to float
error.
Conclusion
The ValueError: invalid literal for int() with base 10
occurs in Python when you try to convert a string representing decimal numbers into an integer using the int()
function.
Because Python can’t convert a string of decimal numbers into an integer directly, you need to call the float()
function to convert the string into a float first. Only then you can call the int()
function.
I hope this tutorial is useful. Happy coding! 🙌