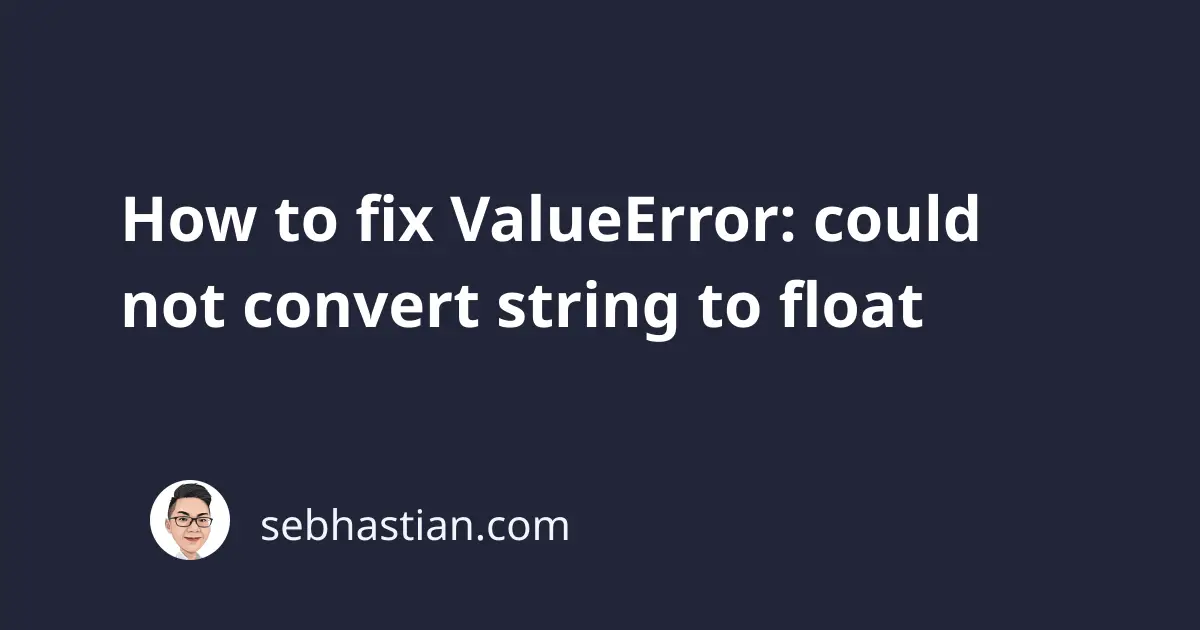
One error you might encounter when running Python code is:
ValueError: could not convert string to float
This error occurs when you attempt to convert a string type to a float type, but the string contains non-numerical value(s)
This tutorial will show an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you have a string type value in your Python code as follows:
my_str = "30,3"
Next, suppose you attempt to convert the string data into a float using the float()
function as follows:
my_float = float(my_str)
You’ll get this error when running the code:
Traceback (most recent call last):
File "main.py", line 2, in <module>
my_float = float(my_str)
ValueError: could not convert string to float: '30,3'
The error tells you that the string value ‘30,3’ can’t be converted to a float. The float()
function can only convert strings that inherently represent float values.
If your string has special characters or alphabets, then Python will throw this error. Here are several other strings that will cause this error:
my_str = "20%"
# ❌ ValueError: could not convert string to float: '20%'
my_str = "7 weeks"
# ❌ ValueError: could not convert string to float: '7 weeks'
my_str = " "
# ❌ ValueError: could not convert string to float: ' '
The %
symbol, the letters, and a whitespace string are all non-compatible with the float type.
How to fix this error
To resolve this error, you need to remove the characters that are causing the error.
For example, if your string contains commas for the decimal numbers, you need to replace it with a dot .
symbol:
my_str = "30,3"
my_float = float(my_str.replace(",", "."))
print(my_float)
Output:
30.3
Next, you need to replace special characters and letters with an empty string. You can use the re.sub()
function and a regular expression to do so:
import re
my_str = "2.5% today!@#"
new_str = re.sub(r'[^\d.]', '', my_str)
my_float = float(new_str)
print(my_float) # 2.5
The regular expression [^\d.]
will match any character that is not a digit or a decimal point. You need to specify the value that will substitute the matches as the second argument, which is an empty string in this case.
The third argument will be the string you want to modify. As a result, you’ll get a string that represents a float.
You might still get this error if the re.sub()
function returns an empty string, so let’s add a try-except
block to handle the error gracefully. Here’s the complete code to resolve this error:
import re
my_str = "0% sales"
# Replace , with . and filter out non-numeric values
new_str = re.sub(r'[^\d.]', '', my_str.replace(",", "."))
# Try to convert the string to float
try:
my_float = float(new_str)
except ValueError:
my_float = 0
print(my_float) # 2.5
You can create a function that performs the conversion for you:
def str_to_float(str_in):
import re
str_in = re.sub(r'[^\d.]', '', str_in.replace(",", "."))
# Try to convert the string to float
try:
float_res = float(str_in)
except ValueError:
float_res = 0
return float_res
Anytime you need to convert strings to floats, just call the function like this:
print(str_to_float("#$ 89 days"))
print(str_to_float("30.5%"))
print(str_to_float("xyz"))
Output:
89.0
30.5
0
Now you can convert strings to floats without receiving the error.
Conclusion
The Python ValueError: could not convert string to float
occurs when you pass a string that can’t be converted into a float to the float()
function.
To resolve this error, you need to remove all elements that are non-compatible with float type like special characters and letters from the string.
But make sure you keep the dot .
symbol to mark the decimal points, though, or the conversion will not match the original string.
I hope this tutorial is useful. See you in other tutorials! 👋