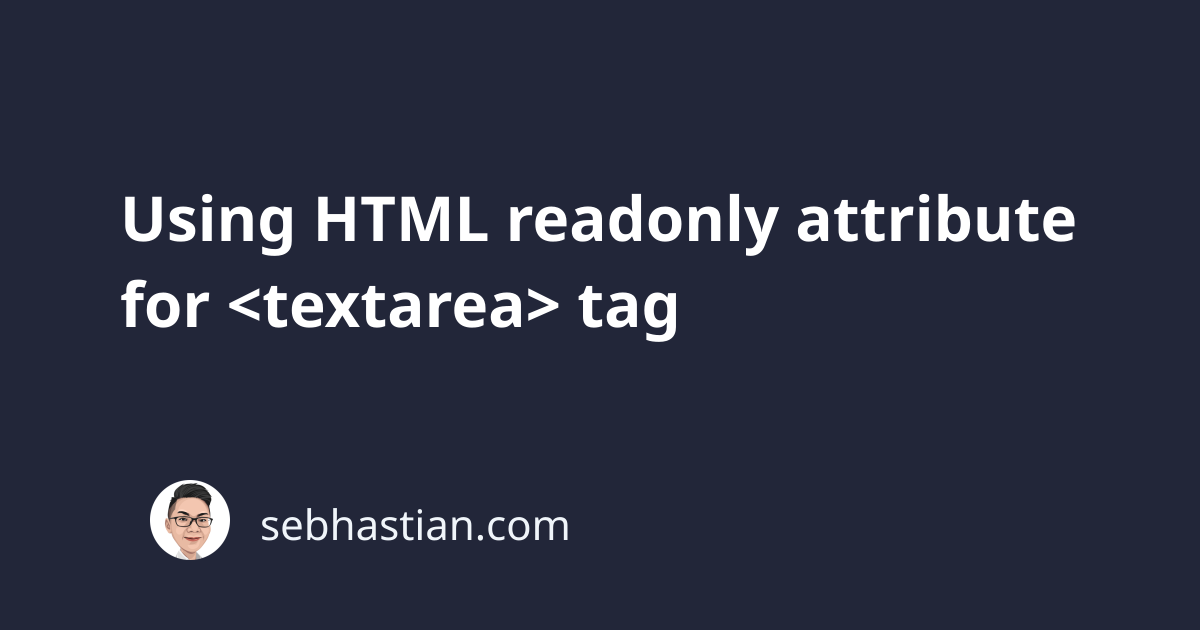
The HTML readonly
attribute is used to make a <textarea>
element as a read-only element.
This means the value
of the rendered <textarea>
element cannot be changed, but you can still copy the text value from the element.
The readonly
attribute is a boolean
attribute, which means you don’t need to assign any value to it.
The presence of the attribute will automatically turn the <textarea>
element into non-editable:
<textarea readonly>
Hello, you are currently learning about the readonly HTML attribute.
This attribute will make your element read-only.
You can't change the value of the element until readonly attribute
is set to false.
</textarea>
At first, the readonly
attribute can only be used on <textarea>
elements, but today you can use the readonly
attribute for <input>
tag of type text
.
<input type="text" name="username" value="Nathan" readonly />
The <input>
element above can be clicked, but you won’t be able to change its value.
The readonly
attribute is a bit similar to the disabled
attribute. You can still copy the value of the element, but you can’t change its value.
The differences between readonly
and disabled
attributes are as follows:
readonly
elements are included in the form submission, whiledisabled
elements are excludedreadonly
elements can be focused by clicking the element, whiledisabled
elements can’t be clicked at all- When navigating input elements using the
Tab
key,disabled
elements will be skipped
The readonly
attribute is usually used when you need to present information obtained from a form.
For example, here’s a basic form presenting the email info that has been submitted previously:
<body>
<form>
<div>
<label for="email">First name:</label>
<input id="email" name="email" type="email" value="[email protected]" readonly />
</div>
<div>
<input type="submit" value="Submit form" />
</div>
</form>
<button id="edit">Edit form</button>
<script>
document.getElementById("edit").addEventListener("click", function () {
document.getElementById("email").readOnly = false;
});
</script>
</body>
The email value from the <input>
element above is retrieved from your server and presented to the user in a readonly
element.
By adding the addEventListener()
method to the edit button, you can remove the readonly
attribute by setting the readOnly
property to false
.
You can learn more about JavaScript addEventListener()
method here:
How to add event listeners to HTML elements using JavaScript
And that’s how the readonly
attribute works 😉