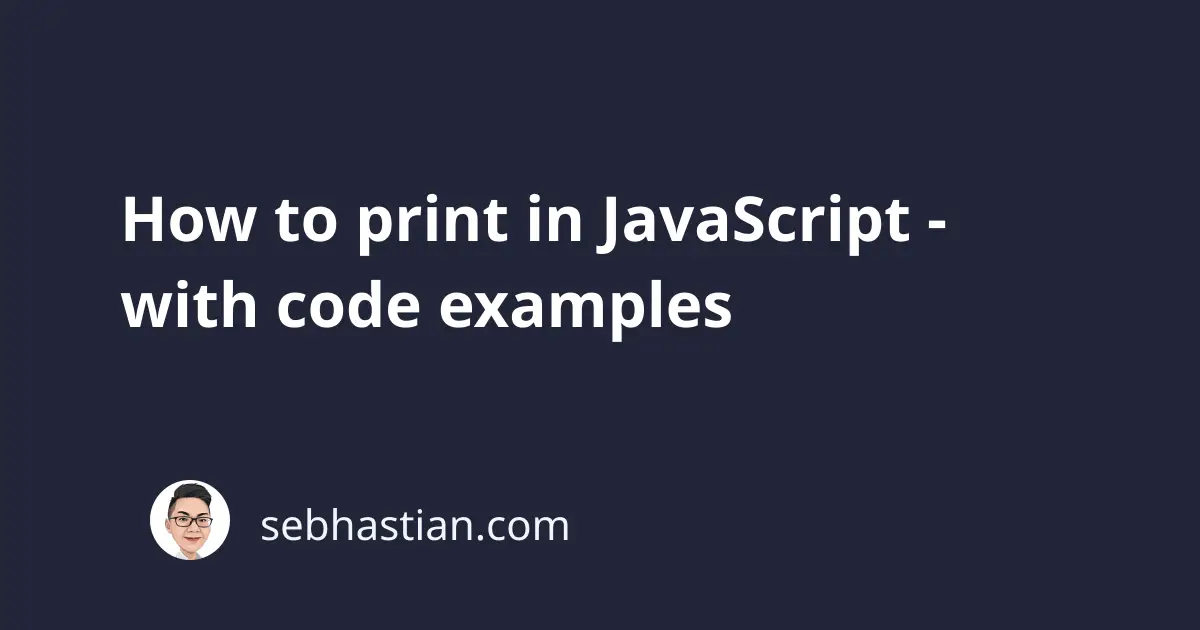
Depending on what you want to print and where you run JavaScript code, there are several ways to print in JavaScript.
When you want to print the current webpage of your browser, you can use the window.print()
method.
The window.print()
method will print the content of the currently active tab in your browser.
You can run the method from the browser console to print the webpage with your printer.
But if you want to print the values and HTML content using JavaScript, then there are several ways to do that:
- Print to the console using
console.log()
- Print to the browser interface using
document.write()
- Print to the alert box using
window.alert()
method - Print to the HTML tag by changing
innerHTML
property value
Let’s see how to do each one of these methods next.
Print with console.log() method
The console.log()
method allows you to print JavaScript data and values to the console.
This is useful when you want to check the value of variables you have in your JavaScript code as shown below:
let name = "Nathan";
console.log(name);
// prints: Nathan
The console.log()
method instructs the JavaScript console to log the value passed as its parameter.
The console is available both on the browser and the Node.js server.
Print with document.write() method
The document.write()
method is used to write data to the <body>
tag of your HTML document.
This method will erase all data stored inside the <body>
tag of your webpage.
For example, suppose you have the following HTML document rendered in your browser:
<html dir="ltr" lang="end">
<head>
<meta charset="utf-8" />
<title>New Tab</title>
</head>
<body>
<h1>Welcome to the Internet</h1>
</body>
</html>
When you run a document.write()
command from the browser’s console, the HTML document above will be overwritten.
Running the following command:
document.write("Hello World!");
Will produce the following output:
<html>
<head></head>
<body>
Hello World!
</body>
</html>
As you can see, all attributes and elements previously written in the document have been erased.
The document
object model is not available in the Node.js server, so you can only use this method from the browser.
Print using window.alert() method
The window.alert()
method is used to create an alert box that’s available in the browser where you run the code.
For example, running the code below:
window.alert("Hello World!");
Will produce the following output:
Using window.alert()
method, you can check on your JavaScript variables and values with the browser’s alert box.
Print by changing the innerHTML property of your element
You can print to a specific HTML element available on your webpage by changing the innerHTML
property of that element.
For example, suppose you have the following HTML document:
<html>
<body>
<h1 id="greetings">Welcome to the Internet</h1>
</body>
</html>
You can select the h1
element above using JavaScript and change its innerHTML
property as shown below:
const header = document.getElementById("greetings");
header.innerHTML = "Hello World!";
The HTML document will have the following changes:
<html>
<body>
<h1 id="greetings">Hello World!</h1>
</body>
</html>
You need to select the HTML element present on your page first.
There are several methods you can use to grab the HTML element present on your browser page. Here are several of them:
Once you get the element, change the innerHTML
property value, and that change will be reflected on your browser page.
Now you’ve learned how to print data and values in JavaScript. Good job! 👍