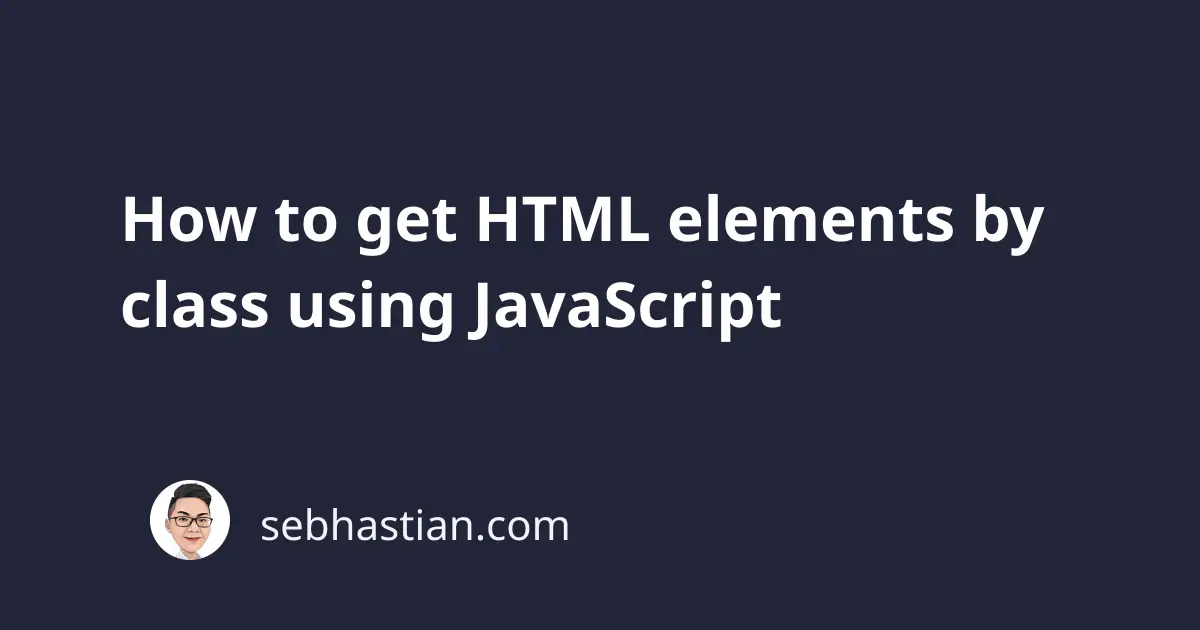
The document.getElementsByClassName()
method is used to get HTML elements by its class name. Suppose you have the following HTML <body>
tag:
<body>
<p class="myParagraph">Hello there!</p>
<p class="myParagraph">Greetings</p>
</body>
You can grab both <p>
tags in the example above by using getElementsByClassName()
method. The tags will be returned as an array-like object called HTMLCollection
:
let paragraphs = document.getElementsByClassName("myParagraph");
console.log(paragraphs); // HTMLCollection(2)
console.log(paragraphs[0].innerHTML); // Hello there!
console.log(paragraphs[1].innerHTML); // Greetings
The getElementsByClassName()
method always returns an array-like HTMLCollection
even if you only have one element with that class name. You can also retrieve elements with more than one class names as follows:
<body>
<p class="paragraph italic">Hello there!</p>
<p class="paragraph bold">Greetings</p>
<p class="paragraph bold">How are you?</p>
<script>
let paragraphs = document.getElementsByClassName("paragraph bold");
console.log(paragraphs.length); // 2
console.log(paragraphs[0].innerHTML); // Greetings
console.log(paragraphs[1].innerHTML); // How are you?
</script>
</body>
Keep in mind that even though you can access HTMLCollection
object’s value like an array, the HTMLCollection
object does not inherit methods from JavaScript Array
prototype object.
This means JavaScript Array
methods like forEach()
, map()
, or filter()
can’t be called from an HTMLCollection
object.
If you want to do something with all elements that match your selection, you need to use the document.querySelectorAll()
method.
See Also: querySelectorAll()
method explained
The querySelectorAll()
method returns a NodeList
object that implements the forEach()
method.
And that will be all about the document.getElementsByClassName()
method. Although there’s nothing wrong with using the method, selecting an element by using its id
attribute is generally preferred over the class
attribute because the id
attribute is more precise and returns only a single element.
But of course, the choice is up to you as the developer to use which method fits your requirement best.