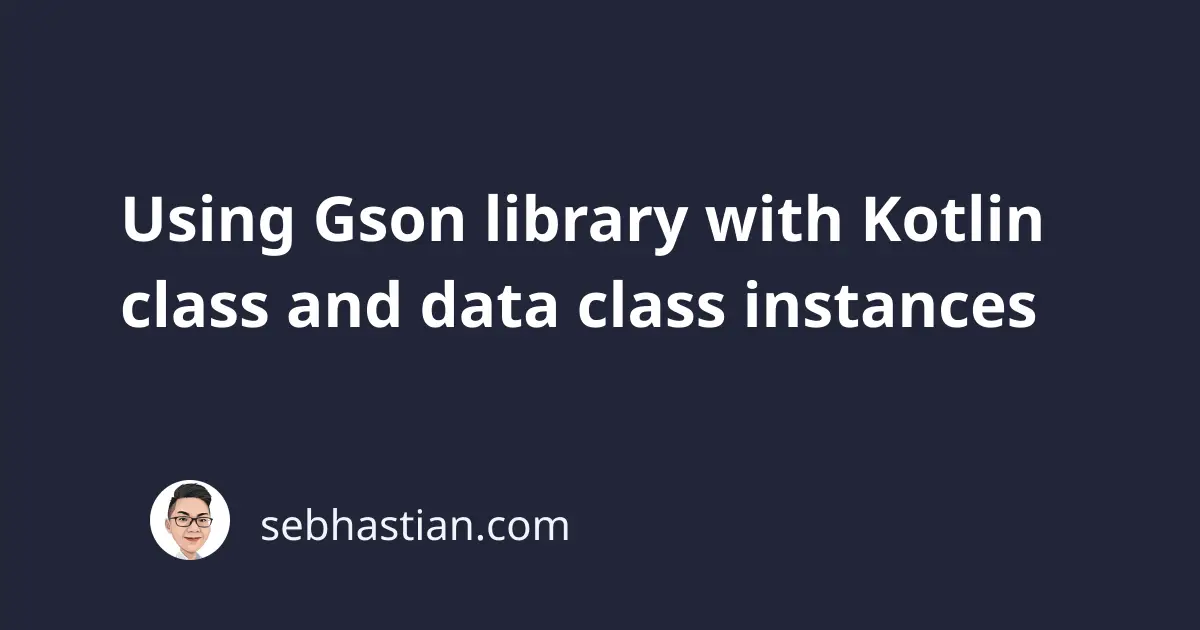
The Gson library is a Java library developed by Google that allows you to serialize and deserialize Java objects to their JSON representation.
The library can also be used in Kotlin to convert Kotlin class
and data class
instances into their JSON representations.
First, you need to add the Gson library as a dependency to your project. If you’re developing an Android application, you can add the dependency in your app/build.gradle
file:
dependencies {
implementation 'androidx.core:core-ktx:1.7.0'
// ...
implementation 'com.google.code.gson:gson:2.8.9'
}
Or if you’re developing a Kotlin app with Maven, add the following to your pom.xml
file <dependencies>
tag:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.9</version>
</dependency>
Once you sync the dependencies for your project, the Gson library should be available for you to use.
Convert Kotlin class into JSON format with Gson
To convert a Kotlin class
instance into its JSON instance, you need to call the Gson().toJson()
method and pass the class instance as its parameter.
Suppose you have a User
data class with the following definition:
data class User(
val firstName: String,
val lastName: String
)
You can create a JSON representation of the User
data class instance as follows:
val myUser = User("Nathan", "Sebhastian")
val jsonString = Gson().toJson(myUser)
println(jsonString)
The jsonString
variable value would be as follows:
{"firstName":"Nathan","lastName":"Sebhastian"}
Both Kotlin class
and data class
instances can be serialized in the same way.
Convert JSON into Kotlin class format
Gson can also be used to convert a JSON string back into Kotlin class instance by calling the fromJson()
method.
The following example takes the userJson
string and converts it into a Kotlin object:
val userJson = """
{
"firstName": "Arya",
"lastName": "Stark"
}"""
val myUser = Gson().fromJson(userJson, User::class.java)
println(myUser.firstName) // Arya
println(myUser.lastName) // Stark
The fromJson()
method accepts two parameters:
- The first is the JSON string to convert to an object
- The second is the class to be used as the object blueprint
And that’s how you deserialize a JSON string using the Gson library.
Adding custom JSON key to Kotlin class
By default, Gson will use the class field names for your JSON keys.
You can define custom JSON keys for each of your field names using the @SerializedName
annotation as follows:
data class User(
@SerializedName("first_name")
val firstName: String,
@SerializedName("last_name")
val lastName: String
)
When you call the toJson()
method, the JSON keys will use the annotations as shown below:
val myUser = User("Nathan", "Sebhastian")
val jsonString = Gson().toJson(myUser)
println(jsonString)
// {"first_name":"Nathan","last_name":"Sebhastian"}
The same goes when you call the fromJson()
method.
And that’s how you can use the Gson library with Kotlin class instances.
When the only purpose of your Kotlin class
is to provide a blueprint for JSON values, it’s recommended to create a data class
instead of a regular class
.
Learn more about Kotlin data class here.