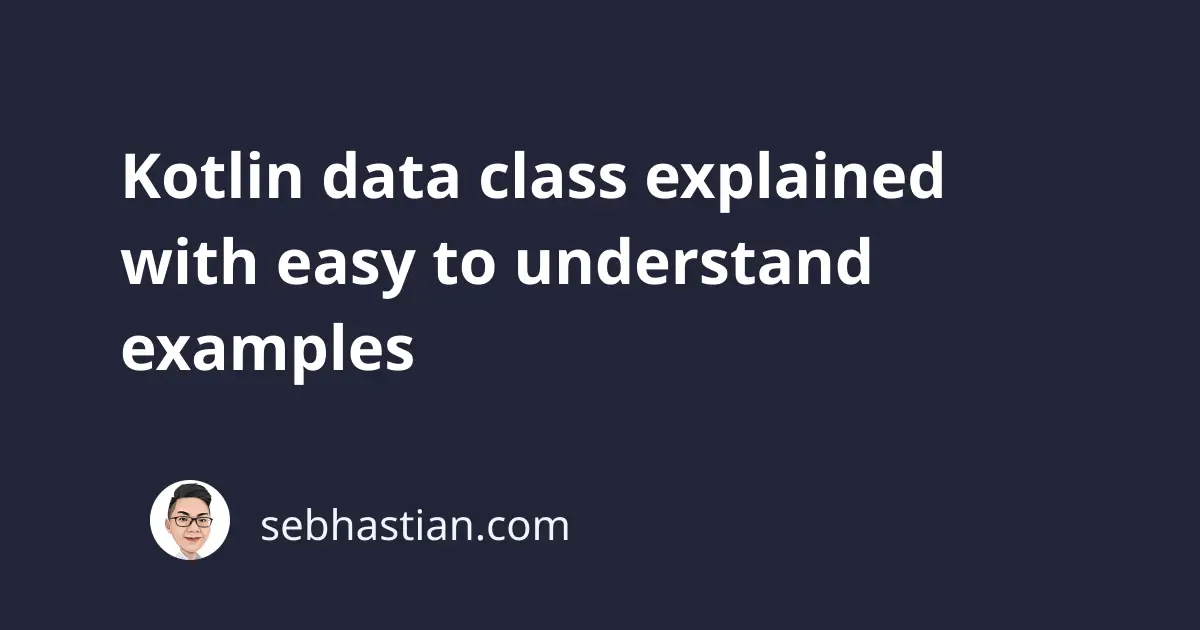
Kotlin data class
is a unique class that’s used to create a class
whose main purpose is to hold data in your source code.
For example, if you have an e-commerce application like Amazon or Shopify, you may have a Product
class that contains data for each of your products.
The following is a simplified example of a Product
class that you may create in Java:
class Product {
public int id;
public String name;
public int price;
public Product(int id, String name, int price) {
this.id = id;
this.name = name;
this.price = price;
}
}
Once you have the Product
class, you can create a new instance of the class in the main()
method as follows:
public static void main(String[] args) {
Product myProduct = new Product(1, "Apple", 5);
myProduct.id = 5;
System.out.println(myProduct.id); // 5
}
In Kotlin, the Product
class can be simplified using the data class
as follows:
data class Product(var id: Int, var name: String, var price: Int)
And that’s it. Once you create the data class
, you can use that class to create a new instance as follows:
var myProduct = Product(1, "Apple", 5)
myProduct.id = 5
println(myProduct.id) // 5
By now you might be wondering what’s the difference between a regular class
and a data class
in Kotlin.
A data class
in Kotlin will generate the following functions for you to use when you need it:
toString()
equals()
/hashCode()
paircomponentN()
function to allow destructuring ofdata class
copy()
function to return a copy of thedata class
instance.
The toString()
function returns the string representation of the constructor. Consider the following example:
var myProduct = Product(1, "Apple", 5)
println(myProduct.toString())
// Product(id=1, name=Apple, price=5)
Without the data class
, the toString()
function will return the object itself instead of the content.
The equals()
and hashCode()
functions are the same as in the normal class
:
- The
equals()
function allows you to compare the object instance equality - The
hashCode()
function returns the hash number of the object instance
The componentN()
function generated for the data class
allows you to destructure the values of the object instance.
Consider the following example:
var myProduct = Product(1, "Apple", 5)
val (id, name) = myProduct
println(id) // 1
println(name) // Apple
Without the componentN()
function, the highlighted line above will cause an error.
Finally, the copy()
function allows you to copy an object instance from a data class
.
You can also change the properties of the class as shown below:
var myProduct = Product(1, "Apple", 5)
val productCopy = myProduct.copy(id = 3, name = "Orange")
println(productCopy.toString()) // Product(id=3, name=Orange, price=5)
Those are the functions generated when you use the data class
keyword in Kotlin.
A data class
also has the following requirements:
- The primary constructor needs to have at least one parameter.
- All primary constructor parameters need to be marked as
val
orvar
. - Data classes cannot be
abstract
,open
,sealed
, orinner
.
And that’s how a data class
works in Kotlin.
A data class
is used when you need a class
whose primary purpose is to hold data in your source code.