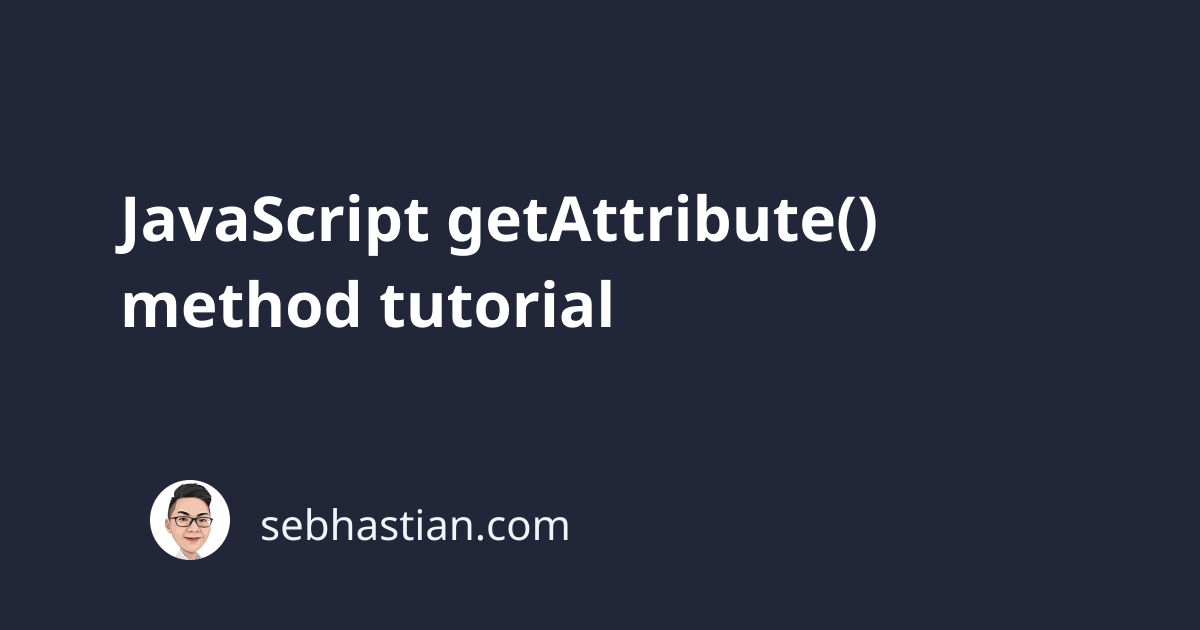
The getAttribute()
method is used when you need to retrieve the value of a known attribute on a certain element.
For example, let’s say you want to find out the value of class
attribute from the HTML <body>
tag. This is how you find it:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>JavaScript getAttribute() method</title>
</head>
<body class="page">
<h1>JavaScript getAttribute() in action</h1>
<script>
let bodyClass = document.body.getAttribute("class");
console.log(bodyClass); // page
</script>
</body>
</html>
Sometimes, you want to find the attribute of a certain element in your HTML. In that case, you need to first get the object representing the element with getElementById()
or querySelector()
method.
The following example shows how you can retrieve the href
attribute of <a>
tag with an id
of "about"
:
<body>
<a id="about" href="/about-page">About</a>
<script>
let aboutLink = document.getElementById("about");
let aboutHref = aboutLink.getAttribute("href");
console.log(aboutHref); // about-page
</script>
</body>
When the specified attribute is missing from the element, the getAttribute()
method will return null
. On the code below,
<body>
<a id="about" href="/about-page">About</a>
<script>
let aboutLink = document.getElementById("about");
let aboutClass = aboutLink.getAttribute("class"); // grab the class attribute
console.log(aboutClass); // null
</script>
</body>
And that’s how you retrieve an attribute using the getAttribute()
method. What to do with the returned attribute value is up to you.
You can use a conditional if/else
code block to decide which code gets executed when the attribute value is of a certain value:
<script>
let aboutLink = document.getElementById("about");
let aboutClass = aboutLink.getAttribute("class"); // grab the class attribute
if (aboutClass === "active") {
// ...
} else {
// ...
}
</script>