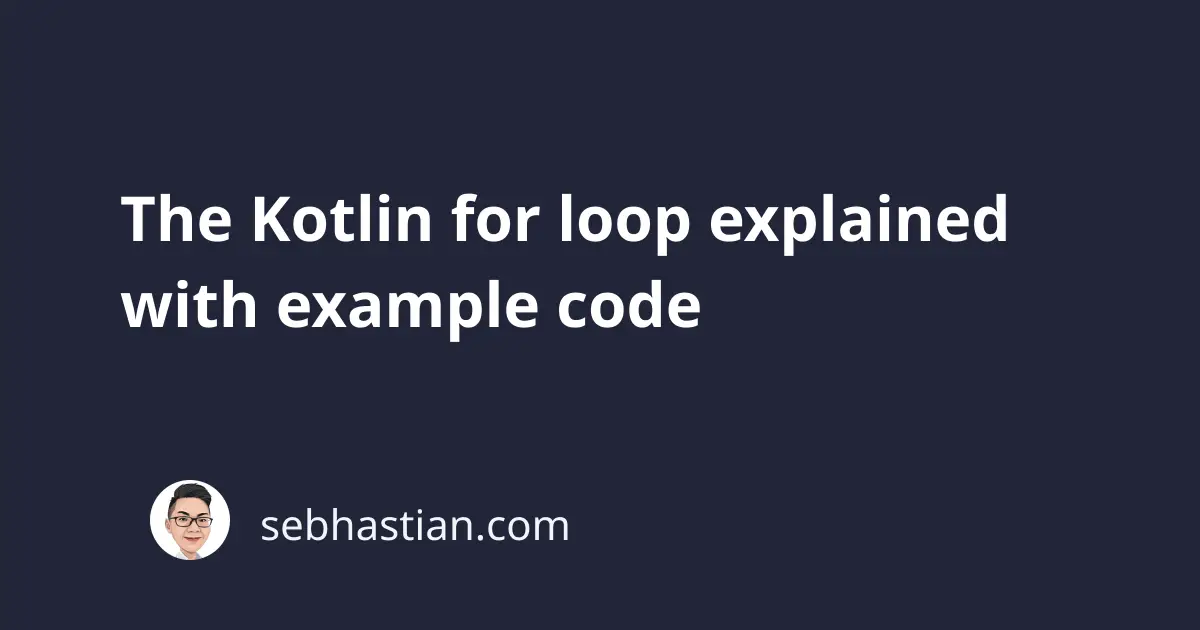
The for
loop in Kotlin works a bit differently than the for
loop in traditional programming languages like Java.
For example in Java, a for
loop is a combination of 3 statements as follows:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
/*
Output:
0
1
2
3
4
*/
But the for
loop in Kotlin doesn’t have a 3 statements part like in Java.
Instead, the Kotlin for
loop allows you to iterate through many things using a simple for ... in
syntax.
Here’s the same Java loop above refactored to Kotlin code:
for (i in 0..4) {
println(i)
}
/*
Output:
0
1
2
3
4
*/
The code above is a valid Kotlin for
loop. The variable i
is used to iterate through a range of numbers between 0
to 4
and print the number to the console.
The in
operator is the magic in Kotlin. It allows you to loop through anything that allows iteration.
In another example, you can loop through a String
value as shown below:
var myString = "Hello!"
for (i in myString) {
println(i)
}
/*
Output:
H
e
l
l
o
!
*/
You can also loop through any array type using the same syntax. Here’s an example of looping over an Int
array:
val numberArr: Array<Int> = arrayOf(10, 20, 30)
for (i in numberArr){
println(i)
}
/*
Output:
10
20
30
*/
And you can also loop over the index stored inside the indices
property of the array. Here’s another example of looping over a String
array:
val nameArr: Array<String> = arrayOf("Jack", "John", "Dan" )
for (i in nameArr.indices){
println("The value at index $i is ${nameArr[i]}")
}
/*
Output:
The value at index 0 is Jack
The value at index 1 is John
The value at index 2 is Dan
*/
Conclusion
To summarize, Kotlin only has one valid syntax of the for
loop, and that is the for ... in
loop.
The for
loop in Kotlin can be used to loop through anything that provides an iterator:
- A range of numbers
- A
String
value - An
Array
object - A
HashMap
object
But keep in mind that some objects like an Array
also have the forEach()
method, so you may want to use that over a for
loop.
When you’re in doubt whether the Kotlin for
loop can iterate through an object, just try writing the statement.
If Kotlin doesn’t complain, it means you can iterate through it 😉
Nice work on learning about Kotlin for
loop! 👍