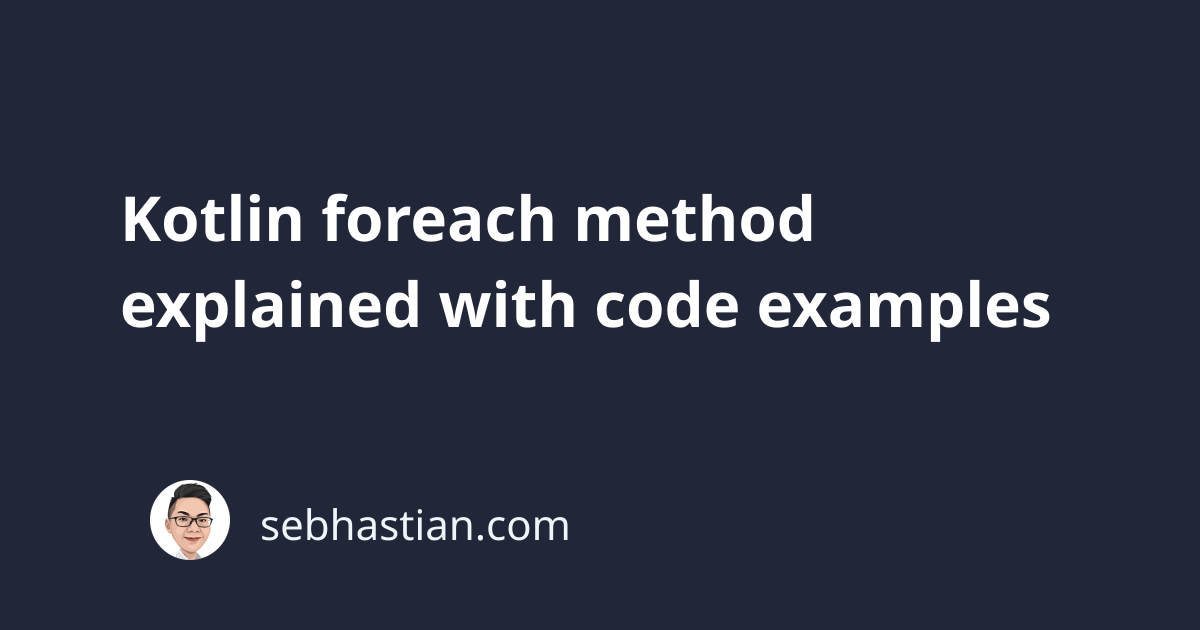
Kotlin has a forEach()
method that you can use to loop over an Array
type variable.
To use it, just call the .forEach
method on the variable with Array data type.
Here’s an example of calling the method on an Array
of String
type:
val nameArr: Array<String> = arrayOf("Jack", "John", "Dan" )
nameArr.forEach {
println("name value = $it")
}
// name value = Jack
// name value = John
// name value = Dan
The forEach
method doesn’t require you to write parentheses and add the name for each iteration of the value.
The current value of the array can be obtained using the it
keyword, which is returned implicitly by the forEach
method.
Finally, the forEach
method can be used in any type of Kotlin Array class (Int
, Boolean
, Char
, etc.)
You can see some example code down below:
val numberArr: Array<Int> = arrayOf(10, 20, 30)
numberArr.forEach {
println("number value = $it")
}
number value = 10
number value = 20
number value = 30
val charArr: Array<Char> = arrayOf('A', 'B', 'C')
charArr.forEach {
println("char value = $it")
}
// char value = A
// char value = B
// char value = C
When calling the method, please make sure that you’re using a capital E
as in forEach
and not foreach
.
Otherwise, you’ll get the Kotlin unresolved reference error thrown in compile time.
Now you’ve learned how the forEach
method works in Kotlin. Well done! 👍