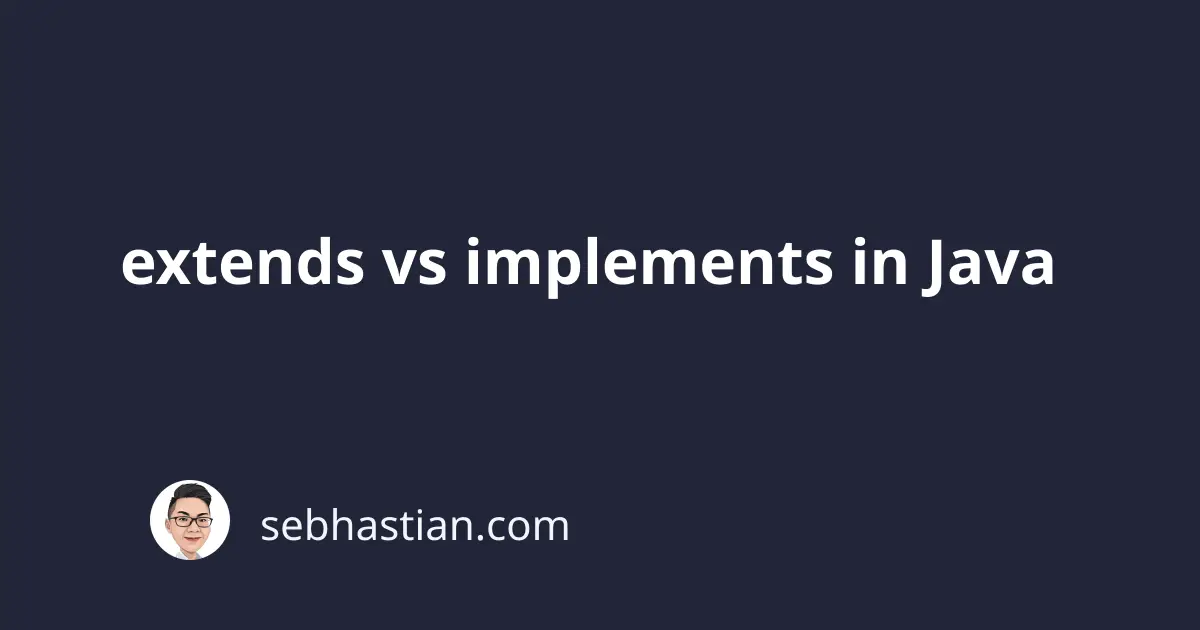
The extends
and implements
keywords are part of the inheritance feature in Java.
The extends
keyword is used whenever you want to extend a class
or an interface
as shown below:
The code below shows how the two keywords work:
// for classes
class Dog {}
class Poodle extends Dog {}
// for interfaces
interface Cat {}
interface Tabby extends Cat {}
A Java class
can only extend one class, while an interface
can extend multiple interfaces:
class Dog {}
class Animal {}
class Poodle extends Dog, Animal {} // ERROR
interface Cat {}
interface Feline {}
interface Tabby extends Cat, Feline {} // OK
The extends
keyword can only be used between the same reference type:
- A class with a class
- An interface with one or more interfaces
When you want to inherit an interface
to a class
, you need to use the implements
keyword:
interface Cat {}
class Tom implements Cat {}
You can implement one or more interfaces to a class, but you can’t extend more than one class.
Now you’ve learned the differences between extends
and implements
keywords. Nice work! 😉