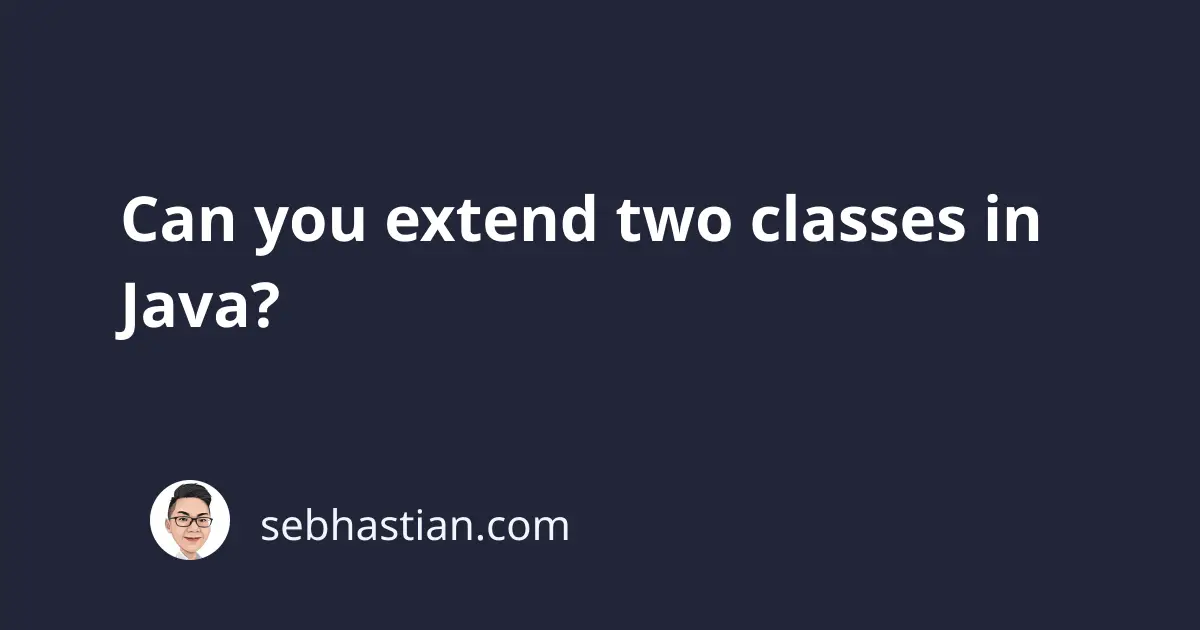
Java is an Object Oriented Programming language that lets you extend a class
to another class
.
Extending a class is also known as the inheritance mechanism. The new class inherits the properties and behaviors of the existing class.
Inheritance is useful because you can reuse the existing class definition as the base of the new class.
In the code below, the Poodle
class inherits the Dog
class, so you can call the Dog
class methods and access its properties from the Poodle
instance:
class Dog {
String genus = "Canis";
void bark() {
System.out.println("Woof!");
}
}
class Poodle extends Dog { }
class Main {
public static void main(String[] args) {
Poodle poo = new Poodle();
poo.bark(); // Woof!
System.out.println(poo.genus); // Canis
}
}
However, Java doesn’t support multiple inheritances. This means you can’t extend two or more classes in a single class.
Extending multiple classes will cause Java to throw an error during compile time:
class Animal {
boolean alive = true;
}
class Poodle extends Dog, Animal { }
// ERROR: Class cannot extend multiple classes
When you need to extend two or more classes in Java, you need to refactor the classes as interfaces.
This is because Java allows implementing multiple interfaces on a single class.
In the following code, the Dog
and Animal
classes are refactored as interfaces:
interface Dog {
String genus = "Canis";
default void bark() {
System.out.println("Woof!");
}
}
interface Animal {
boolean alive = true;
}
class Poodle implements Dog, Animal { }
class Main {
public static void main(String[] args) {
Poodle poo = new Poodle();
poo.bark(); // Woof!
System.out.println(poo.genus); // Canis
System.out.println(poo.alive); // true
}
}
As you can see from the example above, The Dog
and Animal
interfaces can be implemented in the Poodle
class.
This allows you to access the properties and methods of Dog
and Animal
interfaces from any Poodle
class instance.
To conclude, the inheritance mechanism in Java is limited to inheriting one class, but multiple interfaces can be inherited (implemented) on a single class.
An interface
is a reference type used to specify the properties and behaviors of a class
.
You can’t create an instance of an interface
. Instead, you need to implement the interface
on a class
and instantiate the implementing class
.
When you need to extend two classes or more, using interfaces is the only way to go in Java.